How to automatically create API Documentation in Django REST Framework?
Last Updated :
16 Mar, 2021
Prerequisite – https://www.geeksforgeeks.org/how-to-create-an-app-in-django/
Writing documentation can be pretty daunting for developers and to be honest, nobody likes writing long explanations, I personally don’t but as we all know, documentation is equally important as writing a properly functioning code. So, there is a pretty simple way of automatically generating documentation, and wait, not only docs but I will also show you how you can create an online playground too for testing out the APIs.
I don’t know about you but for me, Django is the to-go framework for web development so I will show you how you can do so in Django. So, before we get started, you need to have Django and Django REST Framework installed. I am assuming you already have that. Next, you need to install drf-yasg. To do so, simply type the following in the terminal.
pip install drf-yasg
Now, let’s start with the steps.
First, create a new Django project. I am assuming that you already know how to do that.
Next, go to settings.py and add drf_yasg to INSTALLED_APPS
INSTALLED_APPS = [
...
'drf_yasg',
...
]
Then, go into urls.py and add the driver code for drf_yasg
Python3
from rest_framework import permissions
from drf_yasg.views import get_schema_view
from drf_yasg import openapi
...
schema_view = get_schema_view(
openapi.Info(
title = "Dummy API" ,
default_version = 'v1' ,
description = "Dummy description" ,
contact = openapi.Contact(email = "contact@dummy.local" ),
license = openapi.License(name = "BSD License" ),
),
public = True ,
permission_classes = (permissions.AllowAny,),
)
urlpatterns = [
url(r '^playground/$' , schema_view.with_ui( 'swagger' , cache_timeout = 0 ), name = 'schema-swagger-ui' ),
url(r '^docs/$' , schema_view.with_ui( 'redoc' , cache_timeout = 0 ), name = 'schema-redoc' ),
...
]
|
Now, if you simply run the server and go to localhost:8000/docs, you can see the documentation and if you go to localhost:8000/playground but wait what will the docs show, we have not created any route yet to show the docs. So, let’s quickly create a new app and add a route to it.
First, create an app named demo. I am assuming that you already know to create and add an app to Django settings along with the urls.
Next, go to demo/views.py and add the following code to it.
Python3
from drf_yasg.utils import swagger_auto_schema
from rest_framework import status, permissions, serializers
from rest_framework.response import Response
from rest_framework.views import APIView
class ContactForm(serializers.Serializer):
email = serializers.EmailField()
message = serializers.CharField()
class SendEmail(APIView):
permission_classes = (permissions.AllowAny,)
@swagger_auto_schema (request_body = ContactForm)
def post( self , request):
serializer = ContactForm(data = request.data)
if serializer.is_valid():
json = serializer.data
return Response(
data = { "status" : "OK" , "message" : json},
status = status.HTTP_201_CREATED,
)
return Response(serializer.errors, status = status.HTTP_400_BAD_REQUEST)
|
After this, add this APIView to urls.py to the /email endpoint. That’s it. Now, if you simply run the server on your localhost on port 8000, and go to localhost:8000/docs, you should see the documentation and the playground can be viewed on localhost:8000/playground. It should look something like these.
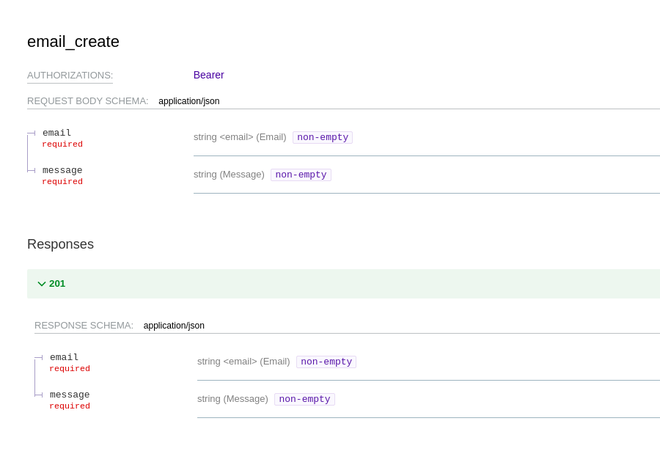
Docs
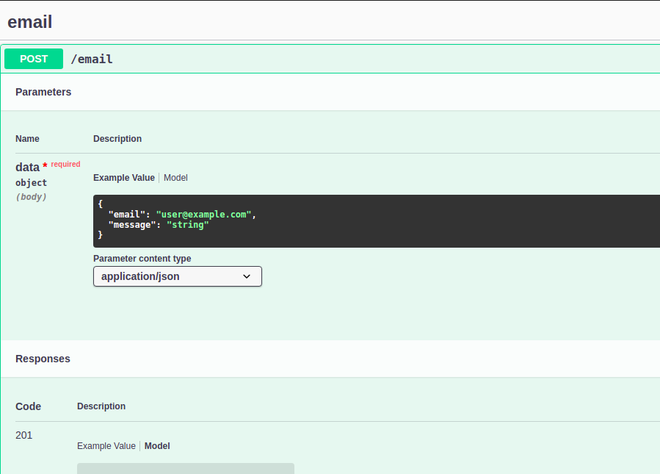
playground
Share your thoughts in the comments
Please Login to comment...