How to add text on an image using pillow in Python ?
Last Updated :
17 Dec, 2020
Prerequisites: PIL
In this article we’ll see how we can add text on an image using the pillow library in Python. Python Imaging Library (PIL) is the de facto image processing package for Python language. It incorporates lightweight image processing tools that aids in editing, creating and saving images. Pillow supports numerous image file formats including BMP, PNG, JPEG, and TIFF.
Method Used
Syntax:
ImageDraw.Draw.text((x, y),"Text",(r,g,b))
Approach
- Import module
- Import image
- Add text
- Save image
Image in use:
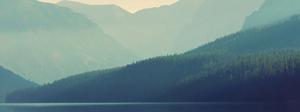
Example 1:
Python3
from PIL import Image
from PIL import ImageFont
from PIL import ImageDraw
img = Image. open ( "gfg.png" )
draw = ImageDraw.Draw(img)
draw.text(( 50 , 90 ), "Sample Text" , ( 255 , 255 , 255 ))
img.save( 'sample.jpg' )
|
Output:
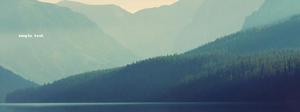
Example 2: Writing text in a specific font
To write the text in a specific font, we need to download the font file for the required font. In the example given below we have used Comic Sans and it can be downloaded from : https://www.wfonts.com/font/comic-sans-ms
Python3
from PIL import Image
from PIL import ImageFont
from PIL import ImageDraw
img = Image. open ( "gfg.png" )
draw = ImageDraw.Draw(img)
font = ImageFont.truetype( "ComicSansMS3.ttf" , 30 )
draw.text(( 50 , 90 ), "Hello World!" , ( 255 , 255 , 255 ), font = font)
img.save( 'sample.jpg' )
|
Output:
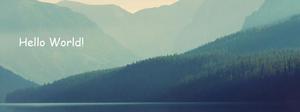
Share your thoughts in the comments
Please Login to comment...