How to Add a Responsive Carousel with Captions in Bootstrap ?
Last Updated :
21 Mar, 2024
Carousels are interactive components in web design, often used to display multiple images or content sequentially. They’re useful for showcasing images one after another, with captions providing additional context or information. We will see how to create a responsive carousel with captions in Bootstrap.
Approach
- First, create a basic HTML structure for the carousel and add the Bootstrap 5 CDN links.
- Then use the “container“ class which contains the carousel and then use the “carousel” class to define the carousel.
- Within the carousel, use the “carousel-inner” to hold the carousel items. Each carousel item should include an image (<img>) and a caption (<div class=”carousel-caption”>).
- And use those utility classes like “carousel-caption”, “img-fluid”, “bg-dark”, “bg-gradient”, and “text-light” for caption, to make the responsive image, background colors, and text color.
- Use flexbox utilities like “d-flex” and “justify-content-center” to center the carousel horizontally.
Example: Implementation of adding a responsive carousel with captions in Bootstrap.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width,
initial-scale=1.0">
<title>Responsive Carousel</title>
<link rel="stylesheet" href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css">
</head>
<body class="bg-success d-flex flex-column min-vh-100">
<div class="container py-5">
<div class="row">
<div class="col-12 text-center">
<h1 class="text-light">
Geeksforgeeks Responsive Carousel in Bootstrap 5
</h1>
</div>
</div>
</div>
<div class="container flex-grow-1">
<div class="row">
<div class="col-md-8 offset-md-2 d-flex
justify-content-center">
<div id="carouselExampleIndicators"
class="carousel slide"
data-bs-ride="carousel"
style="max-width: 600px;">
<div class="carousel-inner">
<div class="carousel-item active">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240308154939/html-(1).jpg"
class="d-block w-100
img-fluid"
alt="Slide 1">
<div class="carousel-caption bg-dark
bg-gradient p-3 rounded">
<h5 class="text-light">HTML Basics</h5>
<p class="text-light">
Master the fundamentals
of HTML programming.
</p>
</div>
</div>
<div class="carousel-item">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240308154940/js-(1).jpg"
class="d-block w-100 img-fluid"
alt="Slide 2">
<div class="carousel-caption bg-dark
bg-gradient p-3 rounded">
<h5 class="text-light">
JavaScript Essentials
</h5>
<p class="text-light">
Explore essential concepts of
JavaScript programming.
</p>
</div>
</div>
<div class="carousel-item">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240308154942/web-(1).jpg"
class="d-block w-100 img-fluid"
alt="Slide 3">
<div class="carousel-caption bg-dark
bg-gradient p-3 rounded">
<h5 class="text-light">
Web Development Basics
</h5>
<p class="text-light">
Learn the core concepts of
web development.
</p>
</div>
</div>
<div class="carousel-item">
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240308154945/web2-(1).jpg"
class="d-block w-100 img-fluid"
alt="Slide 4">
<div class="carousel-caption bg-dark
bg-gradient p-3 rounded ">
<h5 class="text-light">
Advanced Web Topics
</h5>
<p class="text-light">
Dive into advanced topics
in web development.
</p>
</div>
</div>
</div>
<button class="carousel-control-prev"
type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide="prev">
<span class="carousel-control-prev-icon"
aria-hidden="true">
</span>
<span class="visually-hidden">
Previous
</span>
</button>
<button class="carousel-control-next"
type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide="next">
<span class="carousel-control-next-icon"
aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
</div>
</div>
</div>
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js">
</script>
</body>
</html>
Output:
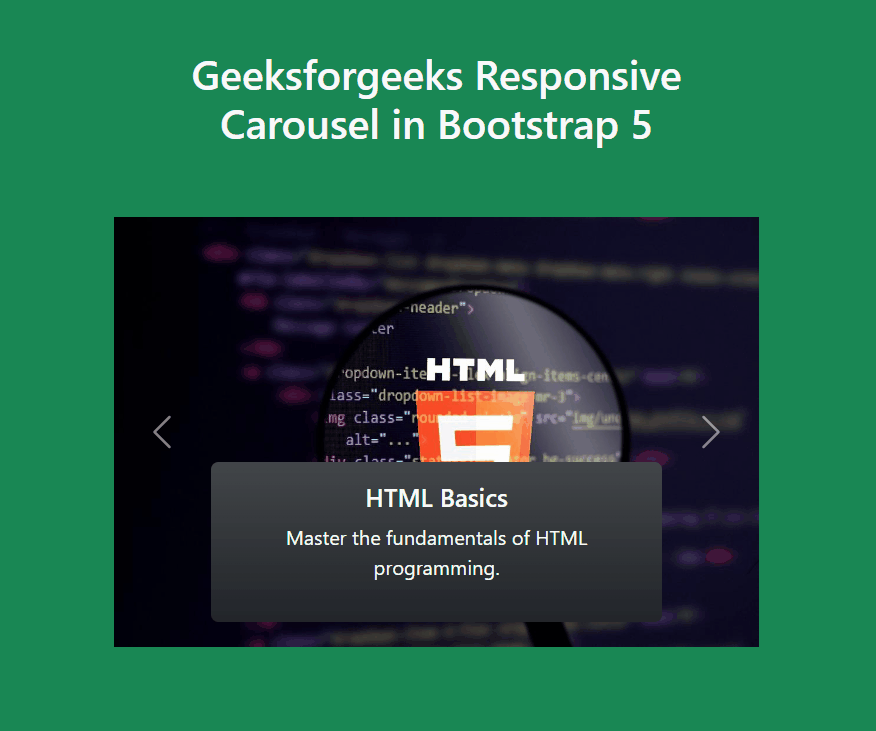
Output
Share your thoughts in the comments
Please Login to comment...