How to Create Carousel with Progress Indicators in Bootstrap ?
Last Updated :
01 Apr, 2024
Bootstrap, the popular front-end framework, provides components to enhance the user experience on websites. One such essential component is the Carousel, which allows you to showcase a series of images or content in an interactive and visually appealing manner. We will look into creating a Bootstrap Carousel with Progress Indicators, adding a dynamic element to your web projects.
Approach
- In the HTML file, Bootstrap CSS and JavaScript files are imported from a CDN to utilize Bootstrap’s styling and functionality for responsive design and carousel components.
- The body section contains a carousel with progress indicators, including carousel items with images and navigation buttons for easy slide transition.
- In the carousel, a progress bar is included to visually indicate the progress of the slideshow, dynamically updating as slides change.
- JavaScript code dynamically adjusts the progress bar width based on slide transitions and retrieves carousel and progress bar elements using document.getElementById and document.querySelector.
- Calculates total carousel slides, initializes variables for the current slide and progress percentage, sets initial attributes and styles for the progress bar, and adds a listener for slide transition events to update the progress bar width.
Example: Implementation of Carousel with Progress Indicators in Bootstrap.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible"
content="IE=edge">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Devloper tools</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity=
"sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH"
crossorigin="anonymous">
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/js/bootstrap.bundle.min.js"
integrity=
"sha384-YvpcrYf0tY3lHB60NNkmXc5s9fDVZLESaAA55NDzOxhy9GkcIdslK1eN7N6jIeHz"
crossorigin="anonymous">
</script>
<style>
@media(width > 700px) {
.carousel {
width: 35%;
}
}
</style>
</head>
<body>
<h1 class="text-center">
Carousal with progress indicators.
</h1>
<div id="carouselExampleIndicators"
class="carousel slide" style="margin: auto;">
<div class="carousel-indicators">
<button type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide-to="0" class="active"
aria-current="true" aria-label="Slide 1">
</button>
<button type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide-to="1"
aria-label="Slide 2">
</button>
<button type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide-to="2"
aria-label="Slide 3">
</button>
</div>
<div class="carousel-inner">
<div class="carousel-item active">
<img width="15px" src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240327032356/gfgimg.png"
class="d-block w-100
mw-[152px] h-[250px] cover">
</div>
<div class="carousel-item">
<img width="15px" src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240327032356/gfgimg.png"
class="d-block w-100
h-[250px] cover">
</div>
<div class="carousel-item">
<img width="15px" src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240327032356/gfgimg.png"
class="d-block w-100
h-[250px] cover">
</div>
</div>
<div class="progress">
<div class="progress-bar"
role="progressbar" style="width: 0%;"
aria-valuenow="0" aria-valuemin="0"
aria-valuemax="100">
</div>
</div>
<button class="carousel-control-prev" type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide="prev">
<span class="carousel-control-prev-icon"
aria-hidden="true"></span>
<span class="visually-hidden">
Previous
</span>
</button>
<button class="carousel-control-next" type="button"
data-bs-target="#carouselExampleIndicators"
data-bs-slide="next">
<span class="carousel-control-next-icon"
aria-hidden="true">
</span>
<span class="visually-hidden">
Next
</span>
</button>
</div>
<script>
const carousel = document.getElementById("carouselExampleIndicators");
const progressBar = document.querySelector(".progress-bar");
const totalSlides = carousel.querySelectorAll(".carousel-item")
.length;
// Assuming initial slide is 1 (can be adjusted if needed)
let currentSlide = 1;
let progressPerSlide = 100 / totalSlides;
progressBar.setAttribute("aria-valuenow", currentSlide);
progressBar.style.width = `${progressPerSlide}%`;
// Update progress bar width on slide change
carousel.addEventListener("slide.bs.carousel", function (event) {
currentSlide = event.to;
const newWidth = progressPerSlide * (currentSlide + 1);
console.log(newWidth, progressPerSlide, currentSlide)
progressBar.setAttribute("aria-valuenow", currentSlide);
progressBar.style.width = `${newWidth}%`;
});
</script>
</body>
</html>
Output:
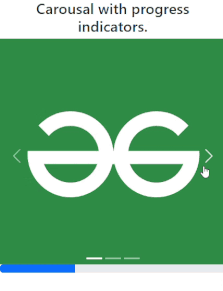
Share your thoughts in the comments
Please Login to comment...