How can we Find an Element using Selenium?
Last Updated :
15 Apr, 2024
Selenium is one of the most popular and powerful tools for automating web applications. Selenium is widely used for automating user interactions on a web application like clicking on a button, navigating to a web page, filling out web forms, and many more. But to interact with a web application we first need to find or locate an element on a webpage.
What is an Element on a web page?
An element is a component of a website or part of the website content that can be identified and interacted with individually.
- Websites or web applications are constructed using HTML (Hyper Text Markup Language) and HTML documents are comprised of various elements each serving a specific purpose and making up the structure and content of the web page.
- Some common examples of elements of a web page are texts, buttons, forms, tables, headings (h1, h2, h3, .. etc.), lists, and many more.
How to Find an Element Using Selenium
To interact with Web applications, we first need to know how to find an element on a web page using Selenium So in this tutorial I’ll show you a step-by-step tutorial on how to Find an element on a Web Page.
Step 1: Setting up Selenium in Python
Make sure to have Python installed in your system then we’ll install Selenium using the following common terminal:
pip install Selenium
Step 2: Installing the necessary modules
Now that we have installed Selenium we need to import Web Driver, By, and ElementNotFoundException from Selenium.
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
Step 3: Initializing the Web Driver and navigating to the web page
Now that we have imported the required modules we will create an instance of the web driver and navigate to the web page
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
url = "http://127.0.0.1:5500/index.html"
driver.get(url)
Explanation:
- webdriver.Chrome() is used to create a instance of the Selenium Web Driver and select Chrome as our automation Web Browser.
- driver.get(url) is a method of selenium web driver which is used to navigate to the specified url.
Step 4: Select a Locating Strategy
Now that we have navigated to the web page to find an element on a web page we first need to select an locating Strategy for the element. Locating Strategy refers to the methods used to identify and locate an element on webpage. Locating Strategies are an important part of interacting with an element on a webpage they allow us to find an element on a webpage.
- ID: ID’s are the unique identifiers for an element in a HTML DOM. In this Strategy ID attribute, an element is used to locate the element on a web page. If an element has an ID attribute then we can use this to locate an element on a webpage.
- CLASS_NAME: Elements with the same functionality share a common class. In this strategy class attribute is used to locate an element on a webpage. If an element has a class then we might consider selecting this locating strategy depending on two conditions. First, if we are locating a single element then the class of the element should be unique it might give false results. Second, if we are thinking about selecting a group of elements then we may select this locating strategy if all the elements of the group have a common class name.
- TAG_NAME: In this strategy, we use the tag name of the element to select an element on a webpage. Similar to the CLASS_NAME locating strategy we can consider selecting this based on two conditions. First, if we are locating a single element we might consider selecting this locating strategy only if the tag name is unique on the HTML which may give false results. Second, if we locate a group of elements then we might consider selecting it only if all the elements of the group have a common tag name.
- NAME: The name attribute is used in HTML forms to handle form controls such as input fields radio buttons etc. In this strategy name attribute is used to locate an element on a webpage
- CSS SELECTOR: In this strategy we use CSS selectors to locate an element on a webpage.
- XPATH: XPATH stands for XML Path Language, XPath is a powerful and flexible way to locate an element on a webpage XPath is used to target an element on a webpage based on its position in an HTML DOM structure.
- LINK TEXTS and PARTIAL LINK TEXTS: Link texts and Partial Link texts are used to locate anchor elements by a link text.
Note:
Make sure you go through the source code in the inspect element of your browser and choose the locating strategy accordingly.
Example:
Here we want to locate the input field of Name on this web page.
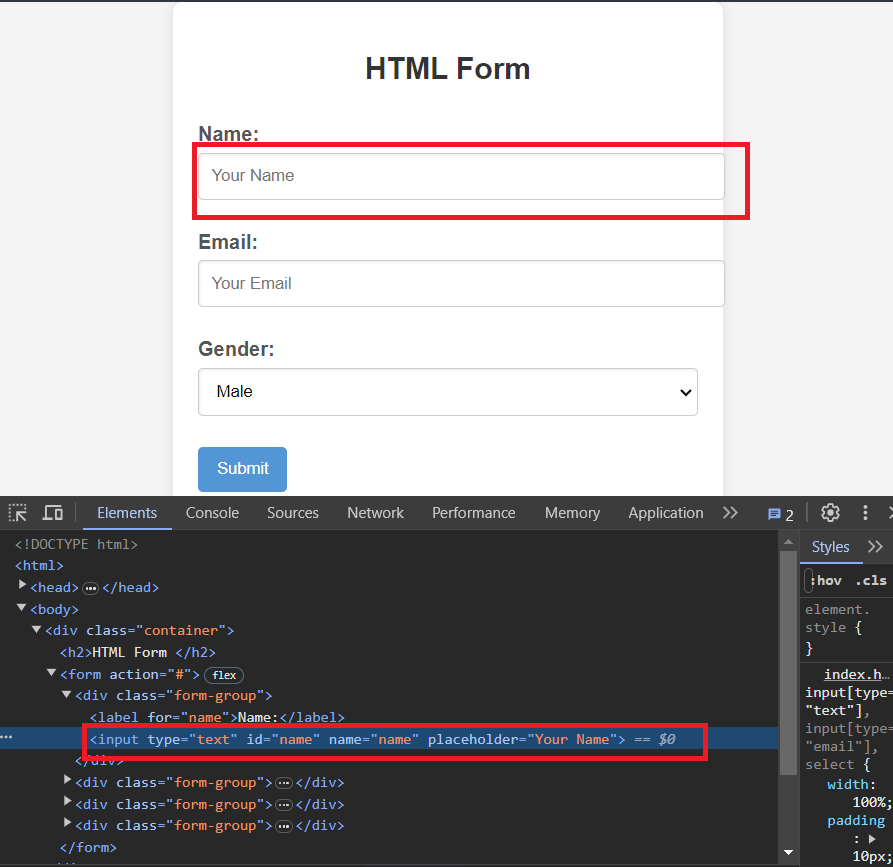
How can we find an element using Selenium?
Here as we can see the name input field has both name and ID attributes so we can use any one of the Name or ID locating Strategies. In this article, we’ll use ID as our locating strategy but you could we Name as well.
Step 5: Finding an Element
Now that we have selected the locating strategy, we’ll find an element on a webpage using the find_element() method and BY.
find_element()– find_element is a method in Selenium which is used to find an element using the specified locator. It returns an instance of the element if found else throws a NoSuchElementException. So we’ll have to use try and catch block to handle the exception.
Python
from selenium import webdriver
from selenium.common import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
url = "http://127.0.0.1:5500/index.html"
driver.get(url)
try:
name_input = driver.find_element(By.NAME, "name")
except NoSuchElementException:
print("Element Not Found")
else:
print("Element Found")
Output:
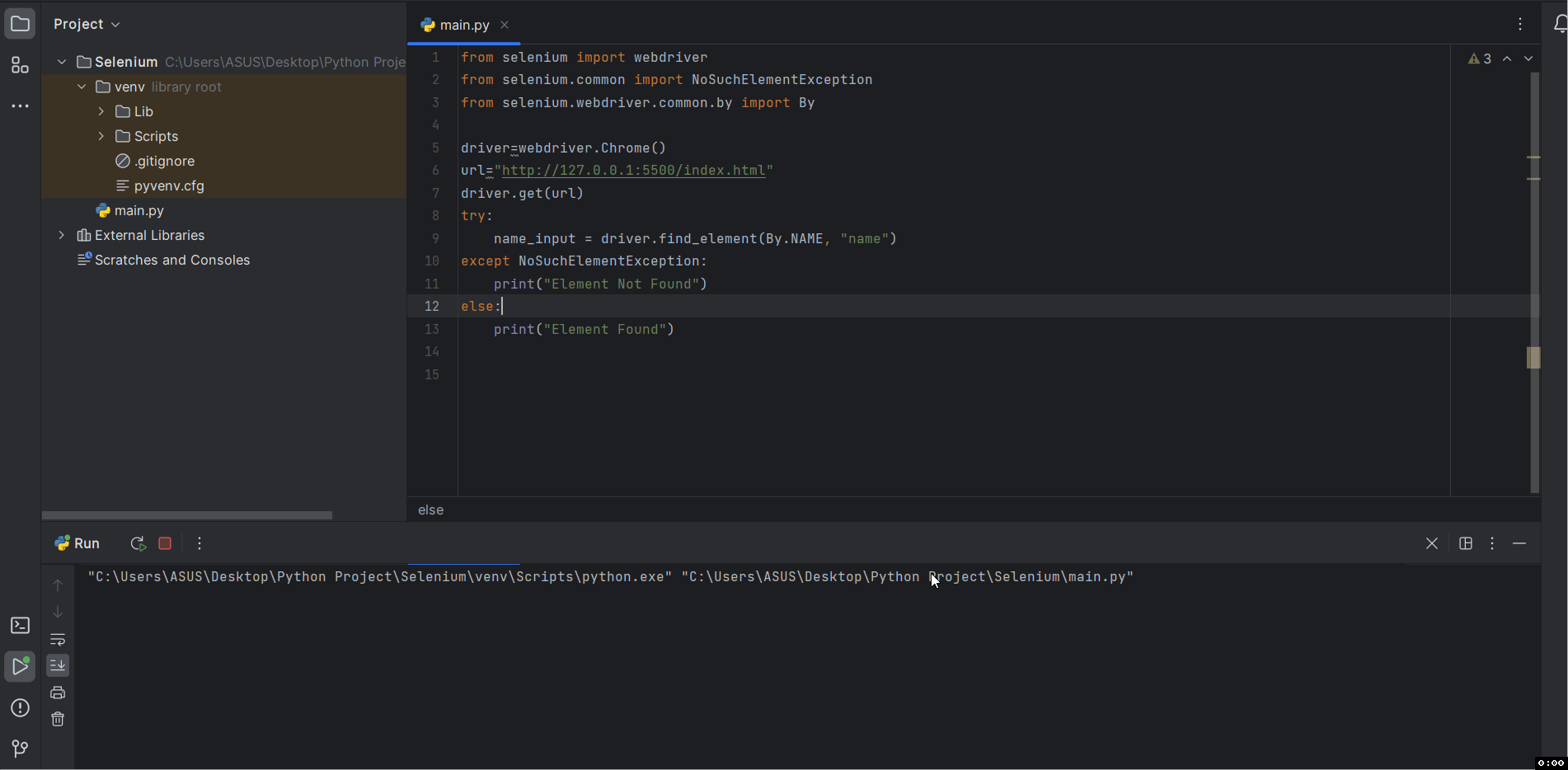
How can we find an element using Selenium?
Step 6: Interacting with the element
Now that we have located the element we can now interact with the element using various methods in Selenium. They are:
- .click(): It is used to click on the element on a website.
- .text: It is an attribute in Selenium that returns the text content inside the element.
- .clear(): It is used to clear the text content of an input field in Selenium.
- .is_displayed(): It is used to check if the element is displayed on the website.
- .is_enabled(): It checks if the element is enabled and interactive on a website.
and many more.
Share your thoughts in the comments
Please Login to comment...