How are actions defined in Redux ?
Last Updated :
23 Nov, 2023
Redux is the state management library for JavaScript apps. It is used to manage the data globally in the app. In redux, there are three parts:
- Actions
- Reducer
- Store
Actions: Actions are the JavaScript objects that have information. Actions provide information for the store. There is one more term that is used to create the action called Action creator, there are simple functions that create fun and return the action object.
Reducer: A reducer in React is a pure function that specifies how the application’s state changes in response to dispatched actions.
Store: A store is an object that holds the application’s state and provides methods for state access and modification in a predictable manner.
Syntax:
function fun_name(parameters) {
return { // action object
type: 'TYPE_NAME',
task: task
}
}
Approach:
Let’s make a simple todo application with help of redux.
- In components add two jsx files
- In redux folder
- Create a folder named actions and add a file index.js inside actions folder.
- Create a folder named reducer and add two files index.js and todoreducer.js inside it.
- Create js file store.js
Steps to Create the React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app example
Step 2: After creating your project folder i.e. example, move to it using the following command:
cd example
Step 3: Installing npm modules:
npm install redux
npm install react-redux
Project Structure:
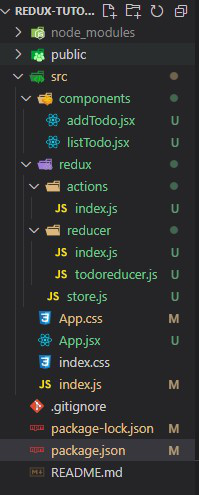
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^8.1.3",
"react-scripts": "5.0.1",
"redux": "^4.2.1",
"web-vitals": "^2.1.4",
}
Example: Below is the implementation of the above discussed approach.
Javascript
import React from 'react' ;
import ReactDOM from 'react-dom' ;
import './index.css' ;
import App from './App' ;
import store from '../src/redux/store' ;
import {Provider} from 'react-redux'
ReactDOM.render(
<React.StrictMode>
<Provider store={store}>
<App />
</Provider>
</React.StrictMode>,
document.getElementById( 'root' )
);
|
Javascript
import AddTodo from './components/addTodo' ;
import ListTodo from './components/listTodo' ;
function App() {
return (
<div className= "App" >
<h1>Todo List</h1>
<AddTodo></AddTodo>
<ListTodo></ListTodo>
</div>
);
}
export default App;
|
Javascript
import React, { useState } from "react" ;
import { useDispatch } from "react-redux" ;
import { addtodoitem } from "../redux/actions" ;
const AddTodo = () => {
const [todo, setTodo] = useState( "" );
const dispatch = useDispatch();
return (
<div>
<h2>Enter the todo</h2>
<label htmlFor= "todoitem" ></label>
<input
type= "text"
id= "todoitem"
value={todo}
onChange={(e) => {
setTodo(e.target.value);
}}
/>
<button
onClick={() => {
dispatch(
addtodoitem({
title: todo,
done: false ,
})
);
setTodo( "" );
}}
>
Add todo
</button>
</div>
);
};
export default AddTodo;
|
Javascript
import React from 'react'
import { useSelector,useDispatch } from 'react-redux' ;
import { deltodoitem } from '../redux/actions' ;
const ListTodo = () => {
const todos = useSelector((state) => state.todo);
const dispatch = useDispatch();
return (
<div>
{ todos.map((todo,index) => (
<div>
<p style={{display: "inline" ,margin: "10px" }}>{todo.title}</p>
<button onClick={()=> {
dispatch(deltodoitem(index))
}} >Delete</button>
</div>
))}
</div>
)
}
export default ListTodo;
|
Javascript
const todoreducer = (state =[],action) => {
if (action.type === "ADD_TODO" ) {
return [...state,action.payload];
}
else if (action.type=== "DELETE_TODO" ) {
return state.filter((item,index) => index !== action.payload )
}
else {
return state;
}
}
export default todoreducer;
const addtodoitem = (item) => {
return {
type: "ADD_TODO" ,
payload : item,
};
};
const deltodoitem = (id) => {
return {
type: "DELETE_TODO" ,
payload : id,
};
};
export {addtodoitem,deltodoitem};
|
Javascript
import todoreducer from "./todoreducer" ;
import { combineReducers } from "redux" ;
const rootReducer = combineReducers({
todo: todoreducer,
})
export default rootReducer;
|
Javascript
import rootReducer from "./reducer" ;
import { createStore } from "redux" ;
const store = createStore(rootReducer);
export default store;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output:
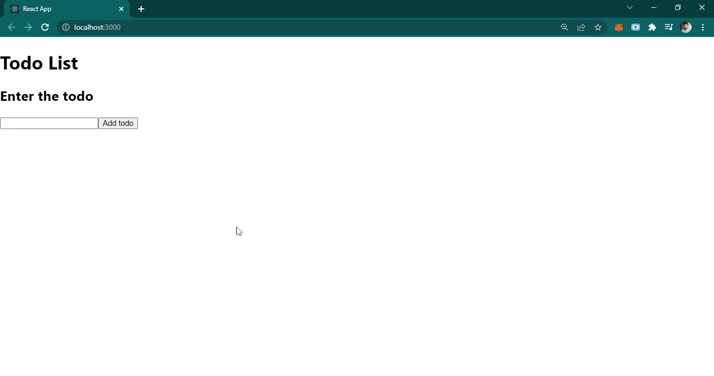
Output
Share your thoughts in the comments
Please Login to comment...