Create a Scientific Calculator using React-Native
Last Updated :
05 Dec, 2023
In this article, we are going to create a scientific calculator using React Native. A scientific calculator is a type of calculator specifically designed to perform complex mathematical, scientific, and engineering calculations. It goes beyond basic arithmetic and includes functions such as trigonometry, logarithms, exponentials, square roots, and other advanced mathematical operations.
Preview of final output: Let us have a look at how the final output will look like.
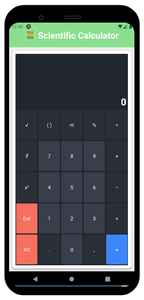
Preview of the app
Prerequisite:
Approach to create Scientific Calculator:
- We Used the react-native-scientific-calculator npm package for the calculator.
- We Define a callback function onOperationPress to handle button presses and log the pressed operation to the console.
- We also added UI with a header and a calculator container.
Steps to Create React Native Application:
Step 1: Create a react native application by using this command in the command prompt
React-native init ScientificCalculator
Step 2: After initiating the project, install the react-native-scientific-calculator package to use the calculator in the app.
npm i react-native-scientific-calculator
Project Structure:
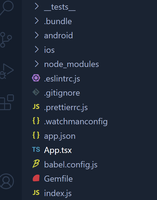
Project structure
The updated dependencies in package.json file will look like:
"dependencies": {
"mobx": "4.1.0",
"mobx-react": "5.0.0",
"@expo/vector-icons": "^13.0.0",
"react-native-elements": "0.18.5",
"react-native-scientific-calculator": "*"
}
Example: Write the below source code into the App.js file.
Javascript
import React from 'react' ;
import {
View, Text,
StyleSheet, StatusBar
} from 'react-native' ;
import ScientificCalculator
from 'react-native-scientific-calculator' ;
const App = () => {
const onOperationPress = (operation) => {
console.log(`Operation pressed: ${operation}`);
};
return (
<View style={styles.container}>
<StatusBar barStyle= "dark-content" />
<View style={styles.header}>
<Text style={styles.title}>
???? Scientific Calculator
</Text>
</View>
<View style={styles.calculatorContainer}>
<ScientificCalculator
onOperationPress={onOperationPress}
theme={{
backgroundColor: '#ffffff' ,
primaryTextColor: '#333333' ,
secondaryTextColor: '#666666' ,
buttonBackgroundColor: '#61dafb' ,
buttonTextColor: '#ffffff' ,
}}
/>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#f0f0f0' ,
},
header: {
backgroundColor: '#90EE90' ,
paddingVertical: 20,
alignItems: 'center' ,
justifyContent: 'center' ,
},
title: {
fontSize: 28,
fontWeight: 'bold' ,
color: '#ffffff' ,
},
calculatorContainer: {
flex: 1,
margin: 10,
padding: 10,
borderRadius: 10,
backgroundColor: '#ffffff' ,
shadowColor: '#000' ,
shadowOffset: { width: 0, height: 2 },
shadowOpacity: 0.3,
shadowRadius: 3,
elevation: 5,
},
});
export default App;
|
Step to Run the Project:
Step 1: Depending on your operating system, type the following command in terminal
React-native run-android
React-native run-ios
Output:
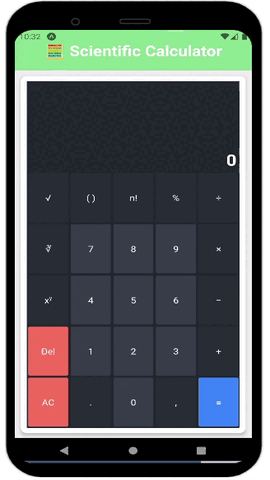
Output
Share your thoughts in the comments
Please Login to comment...