GET and POST in Python CGI
Last Updated :
26 Dec, 2023
In this article, we will explore the GET and POST methods in CGI. In Python, the Common Gateway Interface (CGI) plays an important role as it enables and helps in the creation of dynamic web pages. It basically allows Python scripts to be executed and run on a web server in response to a client’s HTTP request. if you are learning networking for the first time or if you are working on a REST API, you might be familiar with the concept of different HTTP methods. HTTP stands for ‘Hypertext Transfer Protocol,’ and it has no properties. The web operates based on these protocols. For example, we have famous HTTP methods like GET and POST. so let’s see what is GET and POST methods are in CGI.
GET Method in CGI
In GET method, the data is sent in the form of query string to the server’s URL, appending after the question mark (‘?’) structured as key-value pair.
Talking about the GET method, it is said it’s an HTTP request method that is used for fetching data from a specified resource from a web server. Web browsers and web clients primarily use this for fetching or requesting any sort of data such as images, web pages, or any other content from a web server. The GET method is generally used for safe and idempotent operations, meaning it should not have any side effects on the server or the resource being requested. The GET method is like asking a web server nicely for information. It’s a way to get data from a website. Web browsers and other programs use it to request things like pictures, web pages, or other stuff from a website. It’s a safe way to do this, which means it shouldn’t cause any problems or changes on the website or the server.
Let me give you an example to make it clearer. we can understand it by one example.
Example
GetFile.html: This HTML code defines a web page with a form. The form collects user input for a username and email address. When the user clicks the “Submit” button, the form data is sent to a Python script called “File.py” using the GET method, and the data becomes part of the URL as query parameters. The Python script can then retrieve and process this data.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "UTF-8" >
< title > CGI </ title >
</ head >
< body >
< form action = "File.py" method = "get" >
Username: < input type = "text" name = "username" />
< br />
Email Address: < input type = "email" name = "emailaddress" />
< input type = "submit" value = "Submit" />
</ form >
</ body >
</ html >
|
Output
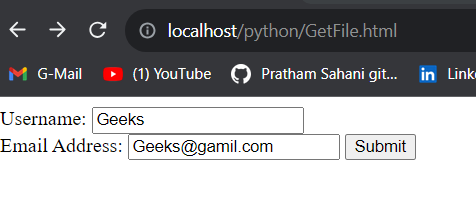
GetFile.html
File.py: This Python script is a CGI (Common Gateway Interface) program. It receives data from an HTML form, specifically the “username” and “emailaddress” fields.
- It imports the necessary CGI modules to handle form data.
- It parses the form data into variables.
- It then generates an HTML response and sends it back to the client’s web browser.
- The response displays the received “username” and “emailaddress” values within an HTML page
Python3
import cgi, cgitb
form = cgi.FieldStorage()
username = form[ "username" ].value
emailaddress = form[ "emailaddress" ].value
print ( "Content-type:text/html\r\n\r\n" )
print ( "<html>" )
print ( "<head>" )
print ( "<title> MY FIRST CGI FILE </title>" )
print ( "</head>" )
print ( "<body>" )
print ( "<h3> This is HTML's Body Section </h3>" )
print (username)
print (emailaddress)
print ( "</body>" )
print ( "</html>" )
|
Output:
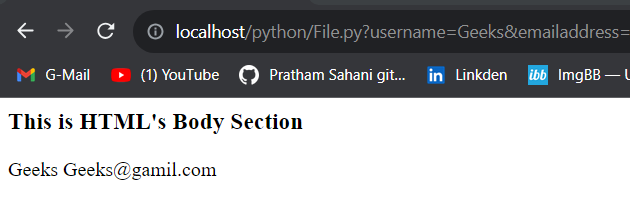
File.py
POST Method in CGI
The POST method in CGI Python scripts is a way to send data to a web server and is commonly used to submit form data or other information from a client to a server.
Talking about the POST method , as it is said it’s a HTTP request method which is used for sending data to the server , as GET method append data to the URL while the POST method sends data in the body of the HTTP request , that is the reason that’s why POST method is suitable for transmitting large quantity of data, and also POST method is used for operations which the the server state, by submitting form, uploading files and by any other data modifying activity. POST method is also one of the HTTP request method which is used for submitting data to a web server. The data sent is basically processed to create, update or modify resources on the server. It is one of the primary ways through which web forms, login pages and other interactive elements on website, send data to the server.
Example
GetFile.html: This HTML document is a simple web page that contains a form. The form is designed to gather user information, specifically a username and an email address. When a user fills in these details and clicks the “Submit” button, the form data will be sent to a Python script named “File.py” using the HTTP POST method. The page’s title is set as “CGI,” and the character encoding is specified as UTF-8 to ensure proper text display.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "UTF-8" >
< title > CGI </ title >
</ head >
< body >
< form action = "File.py" method = "post" >
Username: < input type = "text" name = "username" />
< br />
Email Address: < input type = "email" name = "emailaddress" />
< input type = "submit" value = "Submit" />
</ form >
</ body >
</ html >
|
Output
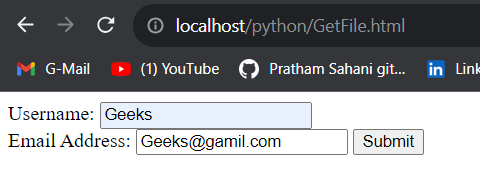
GetFile.html
File.py: The script does four main things. First, it gets information that someone entered into a form on a web page, like their username and email. Second, it creates a special web page (HTML) to show as a response to the person who used the form. Third, it puts the user’s information on this web page along with a title and a message. Finally, it starts by saying which version of Python to use and brings in some tools (modules) for working with web forms and for debugging.
Python3
import cgi, cgitb
form = cgi.FieldStorage()
username = form[ "username" ].value
emailaddress = form[ "emailaddress" ].value
print ( "Content-type:text/html\r\n\r\n" )
print ( "<html>" )
print ( "<head>" )
print ( "<title> MY FIRST CGI FILE </title>" )
print ( "</head>" )
print ( "<body>" )
print ( "<h3> This is HTML's Body Section </h3>" )
print (username)
print (emailaddress)
print ( "</body>" )
print ( "</html>" )
|
Output:
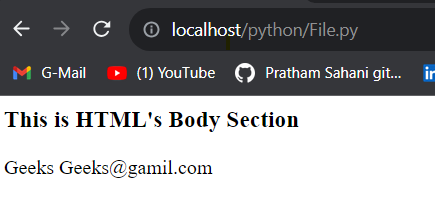
File.py
Share your thoughts in the comments
Please Login to comment...