Flutter – How to Get App Name, Package Name, Version & Build number
Last Updated :
09 Jan, 2024
In this article, we will learn about how to get package name, app name, version and build number and many more things in our Flutter app. The main usecase of using this is when we want to give a new update to users and have to notify them from our app.
Step By Step Implementation
Step 1: Create a New Project in Android Studio
To set up Flutter Development on Android Studio please refer to Android Studio Setup for Flutter Development, and then create a new project in Android Studio please refer to Creating a Simple Application in Flutter.
Step 2: Add a package in the pubspec.yaml file
Dart
dependencies:
package_info_plus: ^5.0.1
|
Step 3: Import the Package
Import the package in the file to access different package
Dart
import 'package:package_info_plus/package_info_plus.dart' ;
|
Step 4: Get Details
Dart
@override
void initState() {
super.initState();
PackageInfo.fromPlatform().then((value) {
print(value);
});
}
|
Step 5: Use it in your app as per your requirement
We will simply display it in text
Complete Code:
Dart
import 'package:flutter/material.dart' ;
import 'package:package_info_plus/package_info_plus.dart' ;
class PackageInfoScreen extends StatefulWidget {
const PackageInfoScreen({super.key});
@override
State<PackageInfoScreen> createState() => _PackageInfoScreenState();
}
class _PackageInfoScreenState extends State<PackageInfoScreen> {
Map<String, dynamic> myPackageData = {};
@override
void initState() {
super.initState();
PackageInfo.fromPlatform().then((value) {
myPackageData = value.data;
setState(() {});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text( "Package Infos Details" ),
),
body: Padding(
padding: const EdgeInsets.all(8.0),
child: Center(
child: Text(
myPackageData.toString(),
style: const TextStyle(fontSize: 20),
),
),
),
);
}
}
|
Sample data for pubspec.yaml file
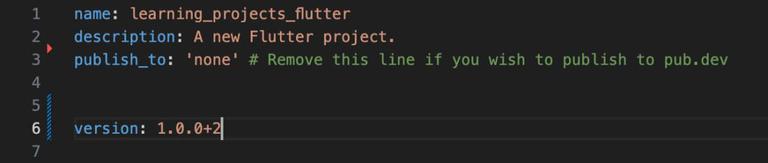
Output:
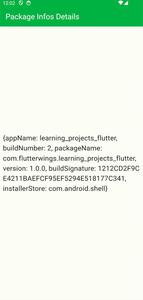
Let’s discuss about each variable we recieves through this package
This is used to show app’s name which you give while creating your app
|
learning_projects_flutter
|
It is build number written in pubspec.yaml file after an version number. For Example 1.0.0+2 your build number will be 3
and your version number will be 1.0.0
|
1
|
Package name of that app with its organization,project_name
|
com.flutterwings.learning_projects_flutter
|
version written in pubspec.yaml file It is build number written in pubspec.yaml file after an version number.
For Example 1.0.0+2
your build number will be 3
and your version number will be 1.0.0
|
1.0.0
|
The build signature. Empty string on iOS, signing key signature (hex) on Android
|
1212CD2F9CE4211BAEFCF95EF5294E518177C341
|
This will give you store from where you have installed this application
|
playstore,appstore,
|
Share your thoughts in the comments
Please Login to comment...