Find two integers X and Y with given GCD P and given difference between their squares Q
Last Updated :
16 Dec, 2021
Given two integers P and Q, the task is to find any two integers whose Greatest Common Divisor(GCD) is P and the difference between their squares is Q. If there doesn’t exist any such integers, then print “-1”.
Examples:
Input: P = 3, Q = 27
Output: 6 3
Explanation:
Consider the two number as 6, 3. Now, the GCD(6, 3) = 3 and 6*6 – 3*3 = 27 which satisfies the condition.
Input: P = 1, Q = 100
Output: -1
Approach: The given problem can be solved using based on the following observations:
The given equation can also be written as:
=> 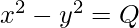
=>
Now for an integral solution of the given equation:
(x+y)(x-y) is always an integer
=> (x+y)(x-y) are divisors of Q
Let (x + y) = p1 and (x + y) = p2
be the two equations where p1 & p2 are the divisors of Q
such that p1 * p2 = Q.
Solving for the above two equation we have:
=>
and 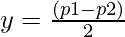
From the above calculations, for x and y to be integral, then the sum of divisors must be even. Since there are 4 possible values for two values of x and y as (+x, +y), (+x, -y), (-x, +y) and (-x, -y).
Therefore the total number of possible solution is given by 4*(count pairs of divisors with even sum).
Now among these pairs, find the pair with GCD as P and print the pair. If no such pair exists, print -1.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int printValidPair( int P, int Q)
{
for ( int i = 1; i * i <= Q; i++) {
if (Q % i == 0) {
int L = i;
int R = Q / i;
int A = (L + R) / 2;
int B = (R - L) / 2;
if (L % 2 != R % 2) {
continue ;
}
if (__gcd(A, B) == P) {
cout << A << " " << B;
return 0;
}
}
}
cout << -1;
return 0;
}
int main()
{
int P = 3, Q = 27;
printValidPair(P, Q);
return 0;
}
|
Java
import java.util.*;
class GFG{
static int printValidPair( int P, int Q)
{
for ( int i = 1 ; i * i <= Q; i++) {
if (Q % i == 0 ) {
int L = i;
int R = Q / i;
int A = (L + R) / 2 ;
int B = (R - L) / 2 ;
if (L % 2 != R % 2 ) {
continue ;
}
if (__gcd(A, B) == P) {
System.out.print(A+ " " + B);
return 0 ;
}
}
}
System.out.print(- 1 );
return 0 ;
}
static int __gcd( int a, int b)
{
return b == 0 ? a:__gcd(b, a % b);
}
public static void main(String[] args)
{
int P = 3 , Q = 27 ;
printValidPair(P, Q);
}
}
|
Python3
import math
def printValidPair(P, Q):
for i in range ( 1 , int (math.sqrt(Q)) + 1 ):
if (Q % i = = 0 ):
L = i
R = Q / / i
A = (L + R) / / 2
B = (R - L) / / 2
if (L % 2 ! = R % 2 ):
continue
if (math.gcd(A, B) = = P):
print (f "{A} {B}" )
return 0
print ( - 1 )
return 0
if __name__ = = "__main__" :
P = 3
Q = 27
printValidPair(P, Q)
|
C#
using System;
class GFG
{
static int printValidPair( int P, int Q)
{
for ( int i = 1; i * i <= Q; i++)
{
if (Q % i == 0)
{
int L = i;
int R = Q / i;
int A = (L + R) / 2;
int B = (R - L) / 2;
if (L % 2 != R % 2)
{
continue ;
}
if (__gcd(A, B) == P)
{
Console.Write(A + " " + B);
return 0;
}
}
}
Console.Write(-1);
return 0;
}
static int __gcd( int a, int b)
{
return b == 0 ? a : __gcd(b, a % b);
}
public static void Main()
{
int P = 3, Q = 27;
printValidPair(P, Q);
}
}
|
Javascript
<script>
function __gcd(a, b) {
if (a == 0)
return b;
if (b == 0)
return a;
if (a == b)
return a;
if (a > b)
return __gcd(a - b, b);
return __gcd(a, b - a);
}
function printValidPair(P, Q) {
for (let i = 1; i * i <= Q; i++) {
if (Q % i == 0) {
let L = i;
let R = Q / i;
let A = (L + R) / 2;
let B = (R - L) / 2;
if (L % 2 != R % 2) {
continue ;
}
if (__gcd(A, B) == P) {
document.write(A + " " + B);
return 0;
}
}
}
document.write(-1);
return 0;
}
let P = 3, Q = 27;
printValidPair(P, Q);
</script>
|
Time Complexity: O(sqrt(Q))
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...