File I/O in Rust
Last Updated :
09 Feb, 2024
File I/O in Rust enables us to read from and write to files on our computer. To write or read or write in a file, we need to import modules (like libraries in C++) that hold the necessary functions. This article focuses on discussing File I/O in Rust.
Methods for File I/O
Given below are some methods and their syntax for various file I/O operations:
Method
|
Module
|
Syntax
|
Description
|
fs::write()
|
std::fs
|
fs::write(“filepath”, “content”)?;
|
Write a slice as the entire contents of a file.
|
fs::read()
|
std::fs
|
let variable_name = fs::read(“filepath”);
|
Read the entire contents of a file into a bytes vector.
|
fs::read_to_string()
|
std::fs
|
let variable_name = fs::read_to_string(“filepath”);
|
Read the entire contents of a file into a string.
|
File::create()
|
std::fs::File
|
let mut variable_file_name = File::create(“filepath”)?;
|
Creates a new file and write bytes to it (you can also use write())
|
write_all()
|
std::io::Write
|
variable_file_name.write_all(content.as_bytes())?;
|
Attempts to write an entire buffer into this writer.
|
File::open()
|
std::fs::FIle
|
let mut variable_file_name = File::open(“filepath”)?;
|
Attempts to open a file in read-only mode.
|
read_to_string()
|
std::io::Read
|
variable_file_name. read_to_stfing(&mut buffer)?;
|
Read all bytes from a reader into a new String. the content will be copied to buffer variable.
|
remove_file()
|
std::fs
|
fs::remove_file(“filepath”)?;
|
Removes a file from the filesystem.
|
copy()
|
std::fs
|
fs::copy(“original_filepath”, “destination_filepath”)?;
|
Copies the contents of one file to another. This function will also copy the permission bits of the original file to the destination file.
|
Examples
Write a File
Writing a file in Rust involves inserting data or information into a specified file. There are two main methods to achieve this: using fs::write() and using File::create(). Both these methods have the same result, but the difference is in the level of control they provide to the programmer.
1. Using fs::write()
To write a file using `fs::write` function, you can use the write function provided by the fs module. This method is straightforward but overwrites the content of a file if the file with the specified name already exists. We import it using `use std::fs;`.
Syntax:
fs::write(“filepath”, content)?;
Code:
Rust
use std::fs;
fn main() -> std::io::Result<()> {
let contents = "Stop acting so small. You are a universe in ecstatic motion." ;
fs::write( "quote.txt" , contents)?;
println!( "\"{}\" has been inserted in the file." , contents);
Ok(())
}
|
Output:
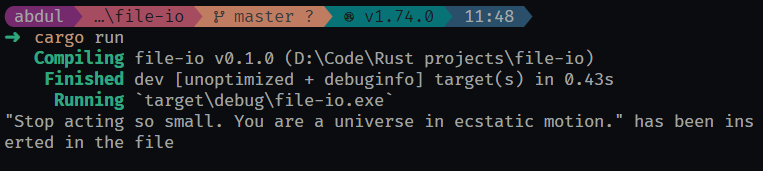
2. Using File::create()
File is an object which provides access to an open file. `File` is an object that provides access to an open file. With `File`, we can perform various operations such as reading, writing, and modifying files. It gives us more control and flexibility over handling files compared to the `read` and `write` functions. We import it using `use std::fs::File;`.
Syntax:
let mut variable_file_name = File::create(“filepath”)?;
Code:
Rust
use std::fs::File;
use std::io::{ self , Write};
fn main() -> io::Result<()> {
let mut file = File::create( "output.txt" )?;
let message = "Hope is the thing with feathers that perches in the soul - and sings the tunes without the words - and never stops at all." .to_string();
file.write_all(message.as_bytes())?;
println!( "Message written to file." );
Ok(())
}
|
Output:
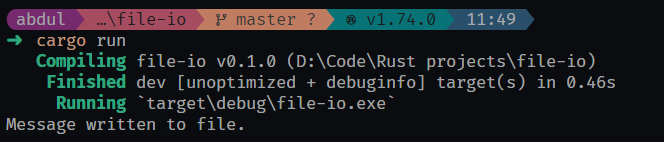
Note:
When `File::create()` was used to create the file, the name and type of the file is specified. But if the file of that name and type already exists then the create method will open that original file instead of creating a new one unlike with `fs::write()` which creates a new file every time it’s called.
Read a File
Reading a file is simply accessing data or information stored in a file and using / displaying it. There are two main methods for reading files: using `fs::read()` or `fs::read_to_string()` and using `File::open()`. Both these methods have the same result, but the difference is in the level of control they provide to the programmer.
1. using fs::read() or fs::read_to_string()
Both the functions are provided by the `fs`. The fs::read function reads the entire contents of a file into a Vec<u8>, while fs::read_to_string() reads the file into a String. These methods are convenient for reading the entire file at once.
Syntax:
let variable_name = fs::read(“filepath”);
let variable_name = fs::read(“filepath”);
Code:
Rust
use std::fs;
fn main() -> std::io::Result<()> {
let data_bytes = fs::read( "quote.txt" )?;
println!( "Data as bytes: {:?}" , data_bytes);
let data_string = fs::read_to_string( "quote.txt" )?;
println!( "Data as string: {:?}" , data_string);
Ok(())
}
|
Output:
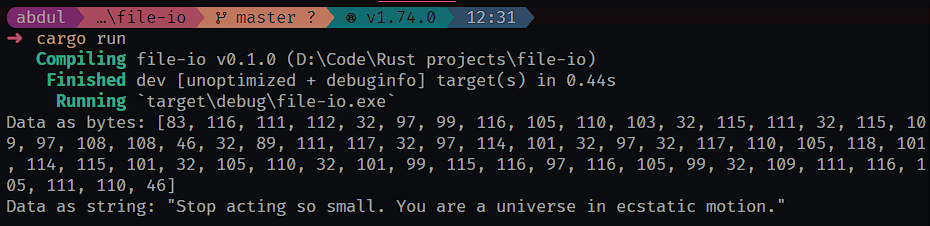
2. Using File::open()
For more control over the reading process, you can use the File::open() method. This method returns a File object, allowing you to use other methods for reading, such as `read_to_end()` or `read_to_string()`.
Syntax:
let mut variable_file_name = File::open(“filepath”)?;
Code:
Rust
use std::fs::File;
use std::io::{ self , Read};
fn main() -> io::Result<()> {
let mut file = File::open( "quote.txt" )?;
let mut buffer = String::new();
file.read_to_string(& mut buffer)?;
println!( "File content:\n{}" , buffer);
Ok(())
}
|
Output:
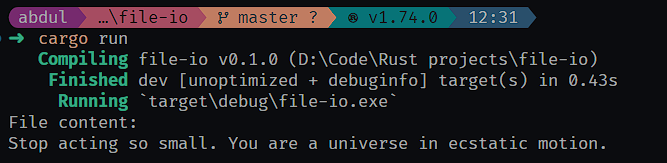
Delete a File
When you are finished with a file and want to remove it, you can use the `remove_file()` provided by the fs module.
Syntax:
fs::remove_file(“filepath”);
Code:
Rust
use std::fs;
use std::io;
fn main() -> io::Result<()> {
fs::remove_file( "quote.txt" )?;
println!( "File removed." );
Ok(())
}
|
Output:
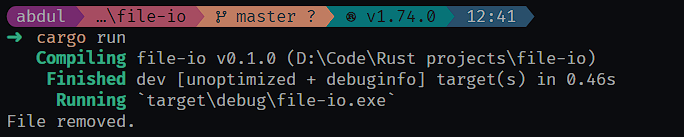
Append Data to a File
Appending data to a file means adding data at the end of a file without overriding the existing data. Appending data in Rust is achieved using the `std::fs::OpenOptions` struct along with the `append` mode .
OpenOptions allows us to configure how a file is opened. When we set to file to append, we will be able to append data into it.
Syntax:
let file_name = OpenOptions::new().write(true).append(true).open(“filepath”);
Code:
Rust
use std::io::{ self , Write};
use std::fs::OpenOptions;
fn main() -> io::Result<()> {
let file = OpenOptions::new()
.write( true )
.append( true )
.open( "foobar.txt" );
let african_proverb = "\nIf you want to go fast, go alone. If you want to go far, go together." ;
file?.write_all(african_proverb.as_bytes())?;
println!( "Data has been appended." );
Ok(())
}
|
Output:
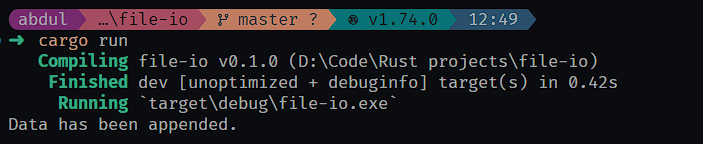
Copy a File
In Rust, we can also copy the file content into another file. The function for copying is provided by the fs module.
Syntax:
fs::copy(“original_filepath”, “destination_filepath”)?;
Code:
Rust
use std::fs;
use std::io;
fn main() -> io::Result<()> {
fs::copy( "output.txt" , "foobar.txt" )?;
println!( "File content copied." );
Ok(())
}
|
Output:
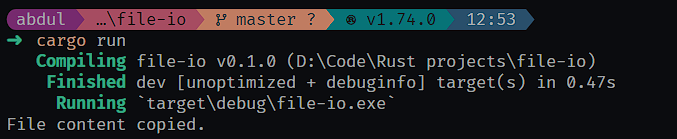
Different Types of Files
1. .txt File
.txt is a plain text file that contains human-readable text without any specific structure. It can any kind of textual information. we use a text file to store data, documentation, source code, etc.
Below is the program to implement .txt file:
Rust
use std::io::{ self , Write};
use std::fs::File;
fn main() -> io::Result<()> {
let message: String = "geekforgeeks.org" .to_string();
let mut file: File = File::create( "website.txt" )?;
file.write(message.as_bytes())?;
println!( "Message written successfully." );
Ok(())
}
|
Output:
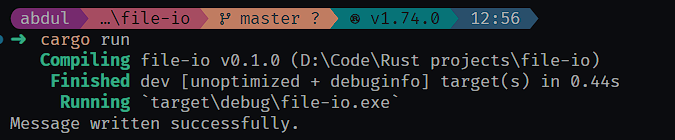
2. .json File
JSON (JavaScript Object Notation) is a popular data format with diverse uses in data interchange. json is a text-based format that is language-indpendent and can be read and used as a data format by any programming language.
We use json files to store structured data, configurations, and even objects. json files can be easily parsed (analyzed) and generated by most programming languages, making it a convenient data interchange format.
Below is the program to implement .json file:
Rust
use std::io::{ self , Write};
use std::fs::File;
use serde_json::json;
fn main() -> io::Result<()> {
let data = json!({
"name" : "GeeksForGeeks" ,
"type" : "Educational" ,
});
let mut file: File = File::create( "website.json" )?;
file.write(data.to_string().as_bytes())?;
println!( "Data written successfully as JSON." );
Ok(())
}
|
Output:
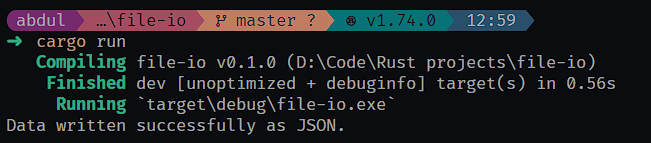
In conclusion, File Input and Output is a very important function of everyday computing life. Rust helps in reading, writing, and deleting files with its inbuilt modules and functions. These tools empower developers to efficiently manage and file interactions in their Rust applications.
Share your thoughts in the comments
Please Login to comment...