Extract a number from a string using JavaScript
Last Updated :
08 Apr, 2024
In this article, we will learn how we can extract the numbers if exist in a given string. we will have a string and we need to print the numbers that are present in the given string in the console.
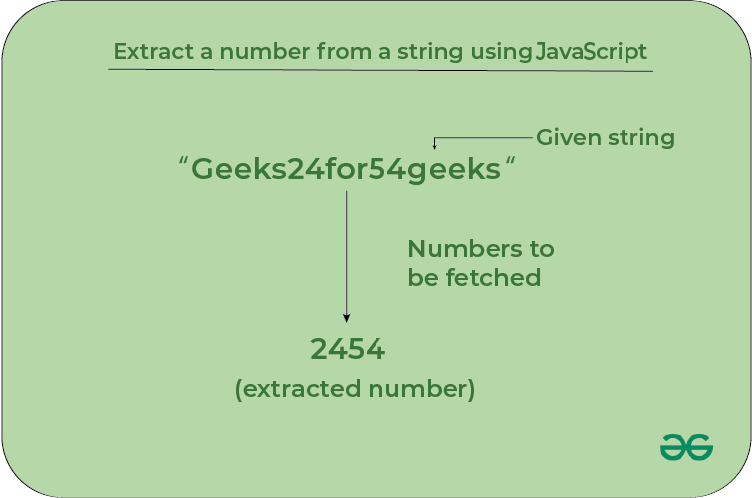
These are the following methods by using these we can solve the problems:
The number from a string in javascript can be extracted into an array of numbers by using the match method. This function takes a regular expression as an argument and extracts the number from the string. The regular expression for extracting a number is (/(\d+)/).Â
Example 1: This example uses the match() function to extract numbers from strings with regular expressions.Â
Javascript
// Function to extract numbers
function myGeeks() {
// Input string
let str = "jhkj7682834";
console.log(str)
// Using match with regEx
let matches = str.match(/(\d+)/);
// Display output if number extracted
if (matches) {
console.log(matches[0]);
}
}
// Function call
myGeeks();
Outputjhkj7682834
7682834
Example 2: This example uses the match() function to extract numbers from strings.Â
Javascript
// Function to extract numbers
function myGeeks() {
// Input string
let str = "foo35bar5jhkj88";
console.log(str)
// Using match with regEx
let matches = str.match(/\d+/g);
// Display output if number extracted
if (matches) {
console.log(matches[0] +', '+ matches[1]+', ' + matches[2]);
}
}
// Function call
myGeeks();
Outputfoo35bar5jhkj88
35, 5, 88
The Javascript arr.reduce() method in JavaScript is used to reduce the array to a single value and executes a provided function for each value of the array (from left to right) and the return value of the function is stored in an accumulator.Â
Example: This example spread operator to convert into an array and the reduce method to extract all the numbers from the string.
Javascript
// Function to extract numbers
function myGeeks() {
// Input string
let str = "jhkj7682834";
let c = "0123456789";
console.log(str);
function check(x) {
return c.includes(x) ? true : false;
}
let matches = [...str].reduce(
(x, y) => (check(y) ? x + y : x),"");
// Display output if number extracted
if (matches) {
console.log(matches);
}
}
// Function call
myGeeks();
Outputjhkj7682834
7682834
The string.replace() is an inbuilt method in JavaScript that is used to replace a part of the given string with another string or a regular expression. The original string will remain unchanged.
Example: In this example, we will use the JavaScript replace() method with regEx to extract the numbers out of given string
Javascript
// Function to extract numbers
function myGeeks() {
// Input string
let str = "jhkj7682834";
console.log(str);
// Using match with regEx
let matches = str.replace(/[^0-9]/g, "");
// Display output if number extracted
if (matches) {
console.log(matches);
}
}
// Function call
myGeeks();
Outputjhkj7682834
7682834
This approach loops through each character in the string and checks if it’s a number using isNaN(). If the character is a number, it appends it to numberStr.
Example: This example use for loop to extract all numbers present in the string.
Javascript
function extractNumbers(str) {
let numbers = "";
for (let i = 0; i < str.length; i++) {
if (!isNaN(str[i])) {
numbers += str[i];
}
}
console.log(numbers)
}
// Input string
let str = "jhkj7682834hhdg237";
console.log(str);
extractNumbers(str);
Outputjhkj7682834hhdg237
7682834237
JavaScript is best known for web page development but it is also used in a variety of non-browser environments. You can learn JavaScript from the ground up by following this JavaScript Tutorial and JavaScript Examples.
Share your thoughts in the comments
Please Login to comment...