Express JS sendfile() vs render()
Last Updated :
25 Dec, 2023
sendFile() function in Express.js is mainly used to send the file at the given path whereas the render() function is used to render a view and send the HTML string to the client as a response. In this article, we will see the detailed difference between this function with their syntax and practical implementation.
Prerequisites:
Steps to Create Express application:
Step 1: In the first step, we will create the new folder by using the below command in the VScode terminal.
mkdir folder-name
cd folder-name
Step 2: After creating the folder, initialize the NPM using the below command. Using this the package.json file will be created.
npm init -y
Step 3: Now, we will install the express dependency for our project using the below command.
npm i express ejs
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.2"
}
What is sendfile() function?
The sendFile() function in Express.js is used to send the file in response to the HTTP request which is done by the client. This function takes the absolute path of the file as the argument and the file which is used to be sent to the client.
Syntax:
res.sendFile(path [, options] [, fn])
Example: Below is the example for the sendFile() function.
Javascript
const express = require( 'express' );
const app = express();
const path = require( 'path' );
const PORT = 3000;
app.get( '/' , function (req, res, next) {
const op = {
root: path.join(__dirname)
};
const gfgFile = 'gfg.txt' ;
res.sendFile(gfgFile, op, function (error) {
if (error) {
next(error);
} else {
console.log( 'File Sent is:' , gfgFile);
}
});
});
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
Output:
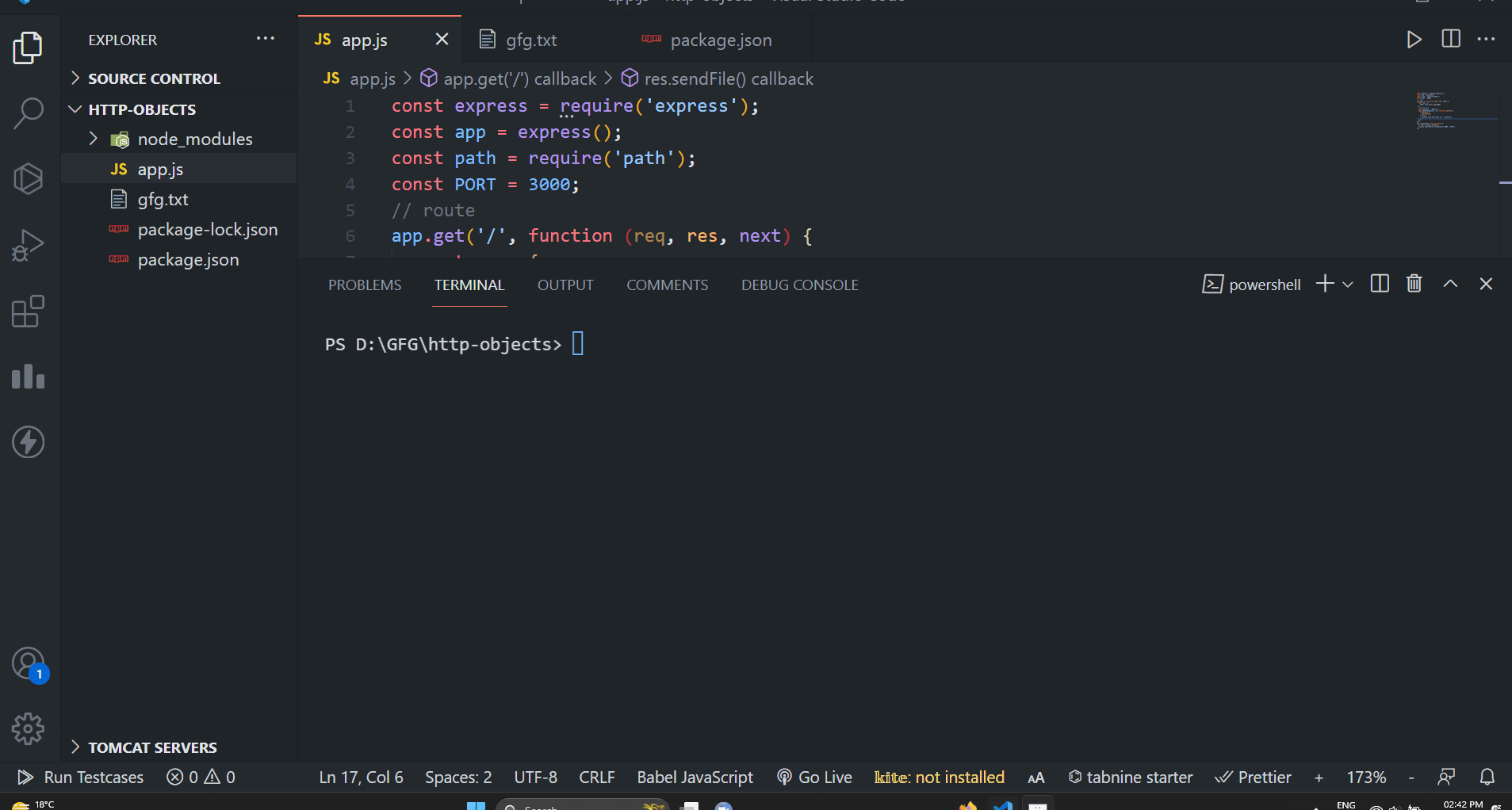
What is render() function?
The render() function in Express is used to generate the dynamic HTML views. It mainly renders specified template files by combining them with the data and facilitating thw creation of dynamic web pages in response to the HTTP request of the clients.
Syntax:
res.render(view, [locals], callback);
Example: Below is the example for the render() function.
Javascript
const express = require( 'express' );
const app = express();
const path = require( 'path' );
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
const data = {
greeting: 'Hello' ,
name: 'GFG'
};
res.render( 'index' , data);
});
app.set( 'views' , path.join(__dirname, 'views' ));
const PORT = 3000;
app.listen(PORT, () => {
console.log(`Server listening at http:
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Express Render Function</ title >
</ head >
< body >
< h1 >
<%= greeting %>, <%= name %>!
</ h1 >
</ body >
</ html >
|
Output:
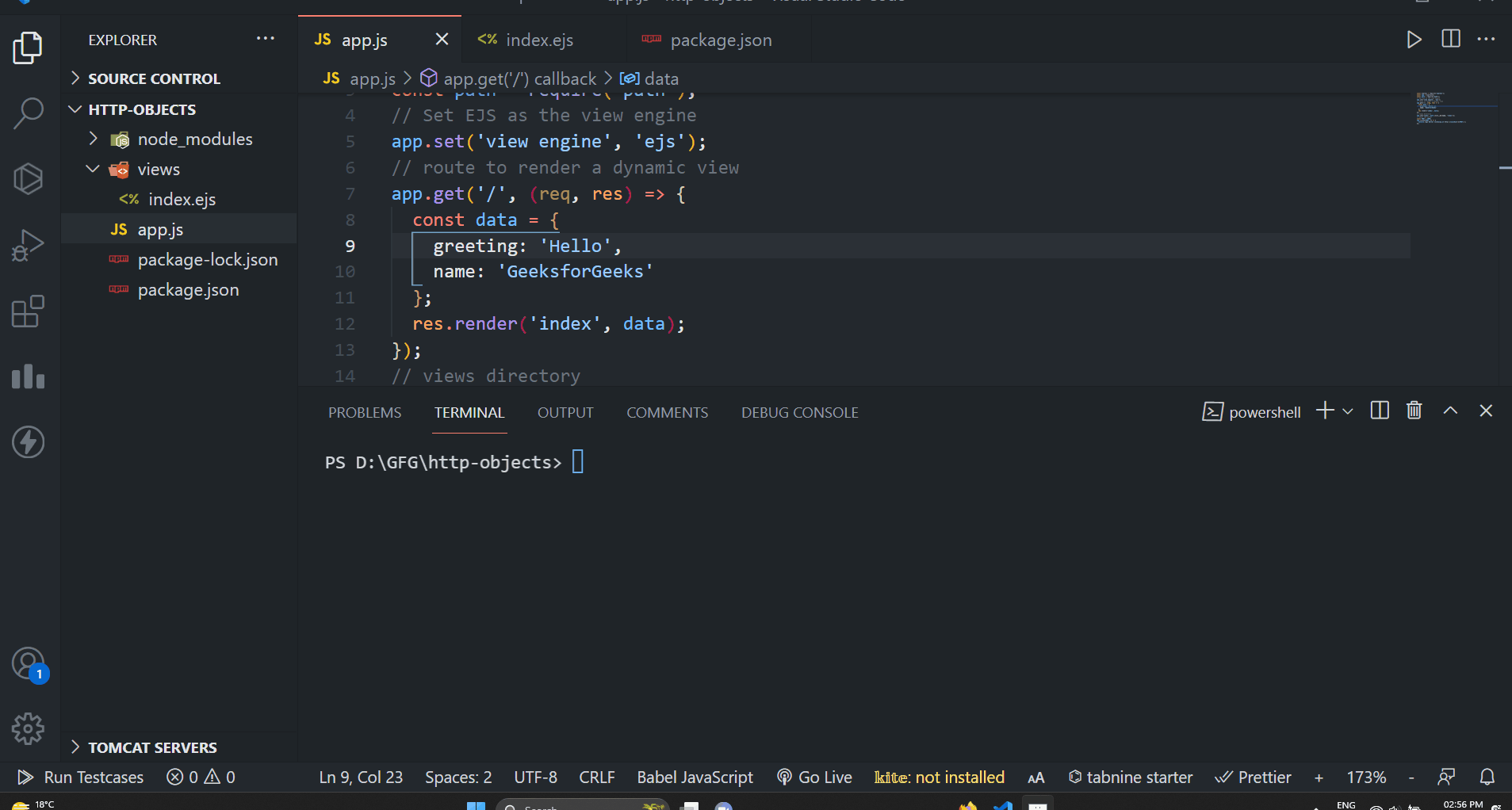
Difference between Express sendfile() and render():
File or Template
|
This function servers the static files directory from the server
|
This function mainly renders the dynamic HTML views using templates
|
Dynamic Data
|
This function doesn’t take dynamic content, it is mostly used for static files.
|
This function requires the object with the data for rendering dynamic content.
|
Middleware Dependency
|
This function is used with template engine middleware
|
This function requires the template engine middleware.
|
Response Handling
|
This function handles file transmission with a callback
|
This function handles dynamic content rendering with a callback.
|
Share your thoughts in the comments
Please Login to comment...