Express res.json() Function
Last Updated :
01 Jan, 2024
The res.json() function sends a JSON response. This method sends a response (with the correct content-type) that is the parameter converted to a JSON string using the JSON.stringify() method.
Syntax:Â Â
res.json( [body] )
Parameters: The body parameter is the body that is to be sent in the response.
Return Value: It returns an Object.
Steps to Install the Express Module:
Step 1: You can install this package by using this command.
npm install express
Step 2: After installing the express module, you can check your express version in the command prompt using the command.
npm version express
Project Structure:
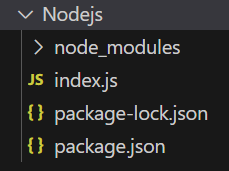
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
}
Example 1: Below is the code of res.json() Function implementation.
javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.get( '/' , function (req, res) {
res.json({ user: 'geek' });
});
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
Steps to run the program:
Run the index.js file using the below command:Â
node index.js
Console Output:Â
Server listening on PORT 3000
Browser Output: Now open the browser and go to http://localhost:3000/, now on your screen you will see the following output:Â
{"user":"geek"}
Example 2: Below is the code of res.json() Function implementation.
javascript
const express = require( 'express' );
const app = express();
const PORT = 3000;
app.use( '/' , function (req, res, next) {
res.json({ title: "GeeksforGeeks" })
next();
})
app.get( '/' , function (req, res) {
console.log( "User Page" )
res.end();
});
app.listen(PORT, function (err) {
if (err) console.log(err);
console.log( "Server listening on PORT" , PORT);
});
|
Steps to run the program:Â
Run the index.js file using the below command:Â
node index.js
Browser Output: Now open a browser and go to http://localhost:3000/, now on your screen you will see the following output:Â
{"title":"GeeksforGeeks"}
Console Output: And you will see the following output on your console:Â
User Page
Share your thoughts in the comments
Please Login to comment...