Explain the Benefits of State Normalization in Redux
Last Updated :
24 Apr, 2024
Redux is a predictable state container for JavaScript apps and offers a powerful solution for managing application states in complex web applications. One strategy that Redux uses is state normalization in which we can restructure state data to improve performance, maintainability, and scalability. In this article, we will discuss the benefits of state normalization in Redux and see why it’s a good practice for front-end developers.
What is State Normalization ?
State normalization is a key concept in Redux, a popular state management library used primarily with React applications. Normalization refers to structuring the state in such a way that it minimizes redundancy and improves data consistency and performance. In this article, we’ll delve into the benefits of state normalization in Redux, covering various aspects and scenarios where normalization plays a crucial role.
Benefits of State Normalization in Redux
Reduces Redundancy
State normalization helps in reducing redundancy by avoiding duplicated data across the state tree. Instead of storing the same data in multiple places, normalization promotes a flat and normalized data structure where related data is stored in separate entities.
Syntax:
// Organizes data to avoid repetition.
const initialState = {
users: {},
posts: {}
};
Improves Data Consistency
By normalizing the state, you can ensure better data consistency throughout your application. Updates to shared data entities are reflected uniformly across components, reducing the chances of inconsistencies or discrepancies.
Syntax:
// Dispatch action to update a post
dispatch({
type: 'UPDATE_POST',
payload: { postId: 'postId', title: 'New Title' }
});
Normalized state can lead to optimized performance, especially in scenarios involving large datasets or complex data relationships. With normalized data, you can efficiently update specific parts of the state without affecting unrelated components or triggering unnecessary re-renders.
Syntax:
// Select specific post from state
const post = state.posts['postId'];
Facilitates Data Retrieval and Updates
Normalized state simplifies data retrieval and updates. Redux selectors can easily extract specific slices of normalized data, making it convenient to access and manipulate data in a predictable manner.
Syntax:
// Retrieve user data using a selector
const user = useSelector(state => state.users['userId']);
Enables Relationship Management
State normalization is beneficial when dealing with relational data structures. It allows you to manage relationships between entities, such as one-to-many or many-to-many relationships, effectively within the state tree.
Syntax:
// Create a comment with related user and post
const comment = {
userId: 'userId',
postId: 'postId',
text: 'Great post!'
};
Enhances Scalability and Maintainability
Normalized state contributes to the scalability and maintainability of Redux applications. As your application grows, a normalized state structure makes it easier to add new features, handle data transformations, and refactor code without introducing complex state management issues.
Syntax:
// Define initial state with normalized structure
const initialState = {
users: {},
posts: {}
};
Supports Immutable Updates
Redux encourages immutable updates to the state, where modifications create new state objects instead of mutating the existing state. Normalization aligns well with this approach, as it promotes updating entities immutably while maintaining referential integrity.
Example: This example where you have a normalized state for managing users and posts data:
{
users: {
byId: {
'user1': { id: 'user1', name: 'John Doe' },
'user2': { id: 'user2', name: 'Jane Smith' },
// More user entities...
},
allIds: ['user1', 'user2'] // Array of all user IDs
},
posts: {
byId: {
'post1': { id: 'post1', title: 'Redux Benefits', author: 'user1' },
'post2': { id: 'post2', title: 'State Normalization', author: 'user2' },
// More post entities...
},
allIds: ['post1', 'post2'] // Array of all post IDs
}
}
Example:
JavaScript
// App.js
import React, { useState } from 'react';
// Before state normalization
const initialStateBefore = {
users: [
{ id: 1, name: 'Vijay', posts: [{ id: 1, title: 'Post 1' }] },
{ id: 2, name: 'Ankur', posts: [{ id: 2, title: 'Post 2' }] }
]
};
// After state normalization
const initialStateAfter = {
users: {
byId: {
1: { id: 1, name: 'Sourav', posts: [1] },
2: { id: 2, name: 'Vishal', posts: [2] }
},
allIds: [1, 2]
},
posts: {
byId: {
1: { id: 1, title: 'Post 1' },
2: { id: 2, title: 'Post 2' }
},
allIds: [1, 2]
}
};
const App = () => {
const [stateBefore] = useState(initialStateBefore);
const [stateAfter] = useState(initialStateAfter);
return (
<div>
<div>
<h2>Before State Normalization</h2>
<p>
In the initial state, the users and their
posts are stored together in a nested structure.
This can lead to duplication of data and
inefficient updates when the same user or
post is referenced in multiple places.
</p>
<div>
<h3>Users</h3>
<ul>
{stateBefore.users.map(user => (
<li key={user.id}>
{user.name}
<ul>
{user.posts.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</li>
))}
</ul>
</div>
</div>
<div>
<h2>After State Normalization</h2>
<p>
After state normalization, the data is
structured in a flat format with entities
separated into individual objects.
This results in better performance,
easier data management, and reduced redundancy.
</p>
<div>
<h3>Users</h3>
<ul>
{stateAfter.users.allIds.map(userId => {
const user = stateAfter.users.byId[userId];
return (
<li key={user.id}>
{user.name}
<ul>
{user.posts.map(postId => {
const post = stateAfter.posts.byId[postId];
return <li key={post.id}>{post.title}</li>;
})}
</ul>
</li>
);
})}
</ul>
</div>
</div>
</div>
);
};
export default App;
Output:
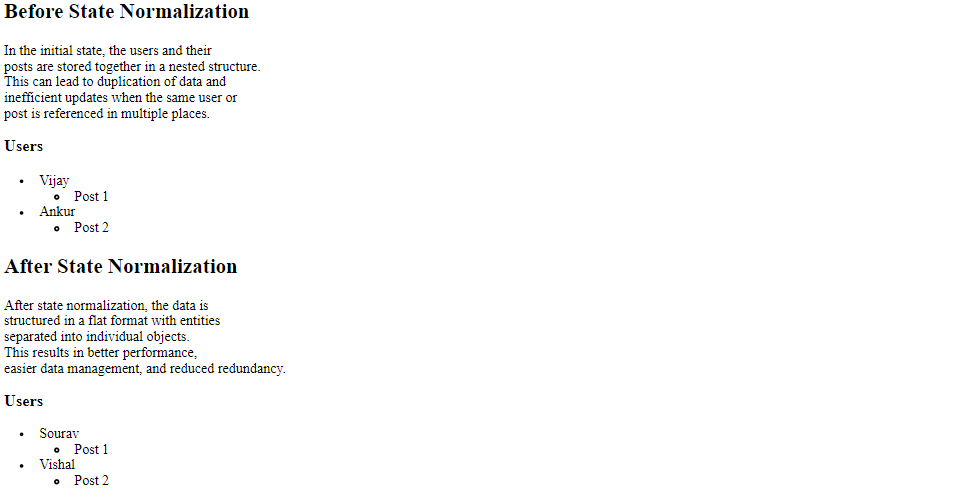
Conclusion
State normalization is a fundamental concept in Redux that brings several benefits to Redux-based applications. By reducing redundancy, improving data consistency, optimizing performance, and facilitating data management, normalization enhances the overall structure, scalability, and maintainability of Redux state, leading to more robust and efficient applications. Incorporating state normalization practices in your Redux projects can significantly improve the development experience and the performance of your applications.
Share your thoughts in the comments
Please Login to comment...