Draggable Element Using JavaScript
Last Updated :
10 Jul, 2023
Dragging an object and moving it to another place within the page is a very interactive and user-friendly concept that makes it easier for the user. This feature allows the user to click and hold the mouse button over an element, drag it to another location, and release the mouse button to drop the element.
Let us have a look at how the final feature will look like:
Prerequisite:
Project Structure:
--index.html
--style.css
--script.js
Approach: We will make this draggable feature to drag any element. Here is the step-by-step approach to creating a draggable element.
- HTML Section: HTML section of the page will be inside the index.html file and we are only creating a div for this project. You can take any element to make it draggable.
- CSS Section: This section will contain all the code related to the styling of the page or the div that we have taken for this project. We are only styling the div and giving it a background color of light green. The code of this file is given below.
- JavaScript Section: This section handles user-defined JavaScript functions like onMouseDrag(). Inside the function, we are just tracking the mouse movement whenever the user clicks on the Element. By taking these values and assigning them to the styling of the div we can easily make the div draggable. To call the onMouseDrag() function we will add EventListener on mousedown and mouseup movement.
Example: Write the below code in the respective files.
- index.html: This file contains the structure of the feature.
- style.css: This file contains the styling of the draggable component.
- script.js: This file contains the logic to move the element.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< link rel = "stylesheet" href = "style.css" >
< title >Draggable Element</ title >
</ head >
< body >
< div class = "container" >
< header >Draggable element</ header >
< div class = "draggable-container" >
< p > This is the example. < br >
Click on me and try to drag within this Page.
</ p >
</ div >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
* {
margin : 0 ;
padding : 0 ;
box-sizing: border-box;
font-family : "Poppins" , sans-serif ;
}
body {
background-color : azure;
}
.container {
position : absolute ;
left : 50% ;
top : 50% ;
min-height : 2 rem;
width : 15 rem;
padding : 10px ;
background-color : rgb ( 218 , 255 , 194 );
border-radius: 5px ;
transform: translate( -50% , -50% );
cursor : move ;
user-select: none ;
}
.container header {
font-weight : 600 ;
text-transform : uppercase ;
border-bottom : 1px solid black ;
}
.draggable-container {
margin : 10px 0 ;
}
|
Javascript
const container = document.querySelector( ".container" );
function onMouseDrag({ movementX, movementY }) {
let getContainerStyle = window.getComputedStyle(container);
let leftValue = parseInt(getContainerStyle.left);
let topValue = parseInt(getContainerStyle.top);
container.style.left = `${leftValue + movementX}px`;
container.style.top = `${topValue + movementY}px`;
}
container.addEventListener( "mousedown" , () => {
container.addEventListener( "mousemove" , onMouseDrag);
});
document.addEventListener( "mouseup" , () => {
container.removeEventListener( "mousemove" , onMouseDrag);
});
|
Output: Click here to see live code output
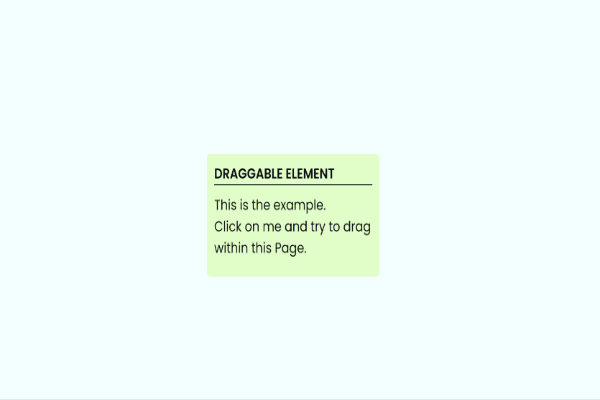
Draggable Element
Share your thoughts in the comments
Please Login to comment...