Download file from URL using PHP
Last Updated :
17 Jan, 2022
In this article, we will see how to download & save the file from the URL in PHP, & will also understand the different ways to implement it through the examples. There are many approaches to download a file from a URL, some of them are discussed below:
Using file_get_contents() function: The file_get_contents() function is used to read a file into a string. This function uses memory mapping techniques that are supported by the server and thus enhances the performance making it a preferred way of reading the contents of a file.
Syntax:
file_get_contents($path, $include_path, $context,
$start, $max_length)
Example 1: This example illustrates the use of file_get_contents() function to read the file into a string.
PHP
<?php
$url =
$file_name = basename ( $url );
if ( file_put_contents ( $file_name , file_get_contents ( $url )))
{
echo "File downloaded successfully" ;
}
else
{
echo "File downloading failed." ;
}
?>
|
Output:
Before running the program:

php source folder
After running the program:

File downloaded after successful execution
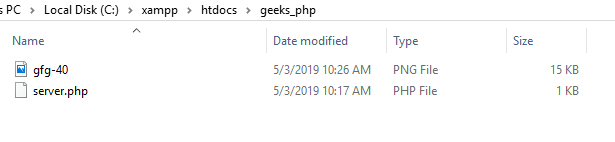
Downloaded image file
Using PHP Curl: The cURL stands for ‘Client for URLs’, originally with URL spelled in uppercase to make it obvious that it deals with URLs. It is pronounced as ‘see URL’. The cURL project has two products libcurl and curl.
Steps to download the file:
- Initialize a file URL to the variable.
- Create cURL session.
- Declare a variable and store the directory name where the downloaded file will save.
- Use the basename() function to return the file basename if the file path is provided as a parameter.
- Save the file to the given location.
- Open the saved file location in write string mode.
- Set the option for cURL transfer.
- Perform cURL session and close cURL session and free all resources.
- Close the file.
Example: This example illustrates the use of the PHP Curl to make HTTP requests in PHP, in order to download the file.
PHP
<?php
$url =
$ch = curl_init( $url );
$dir = './' ;
$file_name = basename ( $url );
$save_file_loc = $dir . $file_name ;
$fp = fopen ( $save_file_loc , 'wb' );
curl_setopt( $ch , CURLOPT_FILE, $fp );
curl_setopt( $ch , CURLOPT_HEADER, 0);
curl_exec( $ch );
curl_close( $ch );
fclose( $fp );
?>
|
Output:
Before running the program:

php source folder
After running the program:
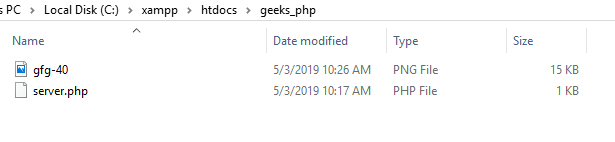
Downloaded image file
PHP is a server-side scripting language designed specifically for web development. You can learn PHP from the ground up by following this PHP Tutorial and PHP Examples.
Share your thoughts in the comments
Please Login to comment...