Dockerizing a Python Flask App with MongoDB: Building a Full-Stack App
Last Updated :
28 Nov, 2023
In this article, we are going to build a stack Flask application with the database MongoDB and then we will Dockerize that Flask app with Mongo DB. As it is a Dockerized Full stack application it can run without any system environment setup. In this case, we are going to build a Student’s Information Web application, this app can view student information from MongoDB and also can take input of student information using the HTML form to store it in MongoDB database.
Primary Terminologies
- Flask: It is REST API Micro Services Web Framework used for the development of web applications in Python programming language.
- MongoDB: MongoDB is a NoSQL database that can store data in JSON (JavaScript Object Notation) format.
- pymongo: It is a Python library that allows Python web applications to perform CRUD (Create Read Update Delete) operations on MongoDB’s databases.
- Full Stack Web Application: The Web application is fully responsive and contains a Front-end (Browser side) and Back-end (server side) is called as Full Stack Web Application.
- Dockerization: Packing the Dependencies of Application into the single or multiple containers. so, that the application can run without dependence on the system Environment.
System Requirements
- Operating System: Linux(ubuntu)/Windows.
- Languages: Python (pymongo, Flask), HTML, yaml.
- Softwares: Docker, Vscode.
Step by Step Process
1. Build Full Stack Web Application
Now we are going to build Student’s Information Full Stack Web application. For that create the directories:
Student_information:
├── templates
├── index.html
├── app.py
app.py:
Python
from flask import Flask, request, render_template, redirect, url_for
from pymongo import MongoClient
app = Flask(__name__)
client = MongoClient(host = 'test_mongodb' , port = 27017 ,
username = 'root' , password = 'pass' , authSource = "admin" )
db = client.mytododb
students_collection = db.students
@app .route( '/' )
def home():
students = students_collection.find()
return render_template( 'index.html' , students = students)
@app .route( '/add_student' , methods = [ 'POST' ])
def add_student():
if request.method = = 'POST' :
student_data = {
'name' : request.form[ 'name' ],
'roll_number' : request.form[ 'roll_number' ],
'grade' : request.form[ 'grade' ]
}
students_collection.insert_one(student_data)
return redirect(url_for( 'home' ))
if __name__ = = '__main__' :
app.run(host = '0.0.0.0' , debug = True )
|
inside template folder index.html:
HTML
<!DOCTYPE html>
< html >
< head >
< title >Student Information</ title >
</ head >
< body >
< h1 >Student Information</ h1 >
< form method = "POST" action = "/add_student" >
< label for = "name" >Name:</ label >
< input type = "text" name = "name" required>
< br >
< label for = "roll_number" >Roll Number:</ label >
< input type = "text" name = "roll_number" required>
< br >
< label for = "grade" >Grade:</ label >
< input type = "text" name = "grade" required>
< br >
< button type = "submit" >Add Student</ button >
</ form >
< br >
< h2 >Students:</ h2 >
< ul >
{% for student in students %}
< li >{{ student.name }} (Roll Number: {{ student.roll_number }}, Grade: {{ student.grade }})</ li >
{% endfor %}
</ ul >
</ body >
</ html >
|
now we have successfully build Python Flask App with MongoDB.
2.Dockerization of Web App
create Dockerfile, docker-compose.yml and requirements.txt inside the Student_information folder and the folder Structure look like this:
Student_information:
├── templates
├── index.html
├── app.py
├── Dockerfile
├──docker-compose.yml
├──requirements.txt
Folder Structure in VsCode will looks like this:
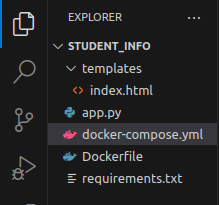
Dockerfile
FROM python:3.6
ADD . /app
WORKDIR /app
RUN pip install -r requirements.txt
docker-compose.yml:
web:
build: .
command: python -u app.py
ports:
- "5000:5000"
volumes:
- .:/app
links:
- db
db:
image: mongo:latest
hostname: test_mongodb
environment:
- MONGO_INITDB_DATABASE=Student_db
- MONGO_INITDB_ROOT_USERNAME=root
- MONGO_INITDB_ROOT_PASSWORD=pass
ports:
- 27017:27017
requirements.txt
pymongo
flask
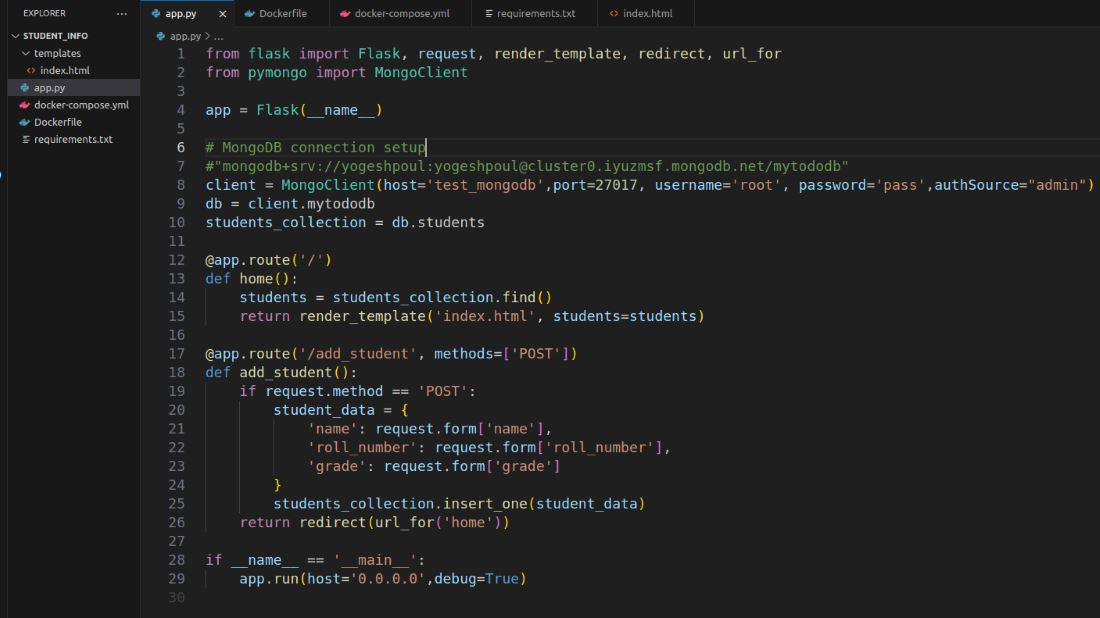
Project in Vs code for reference
3. Build Docker Containers
Enter the following command in the terminal to build and run the docker containers.
docker-compose up --build
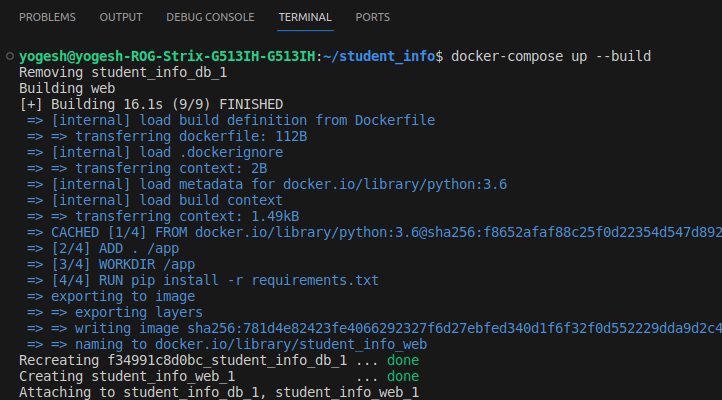
Containers Building
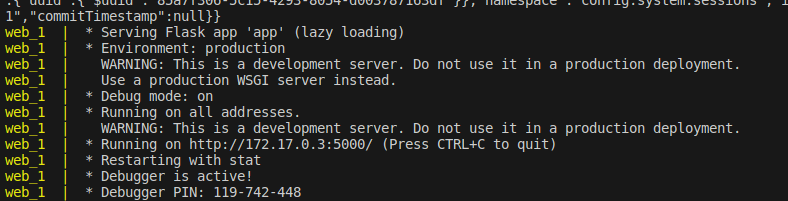
containers are running successfully
If you are getting following error while building docker image by running the command docker-compose up –build:
ERROR: for f34991c8d0bc_student_info_db_1 Cannot start service db: driver failed programming external connectivity on endpoint student_info_db_1 (4a25c142988bf13040049dd935f93873fdb8cffe814d9c8f575072aa3885 3465): Error starting userland proxy: listen tcp4 0.0.0.0:27017: bind: address already in use.ERROR: for db Cannot start service db: driver failed programming external connectivity on endpoint student_info_db_1 (4a25c142980bf13040849dd935f93873fdb8cffe814d9c8f575872aa38853465): Error starting userla nd proxy: listen tcp4 0.0.0.0:27017: bind: address already in use ERROR: Encountered errors while bringing up the project.
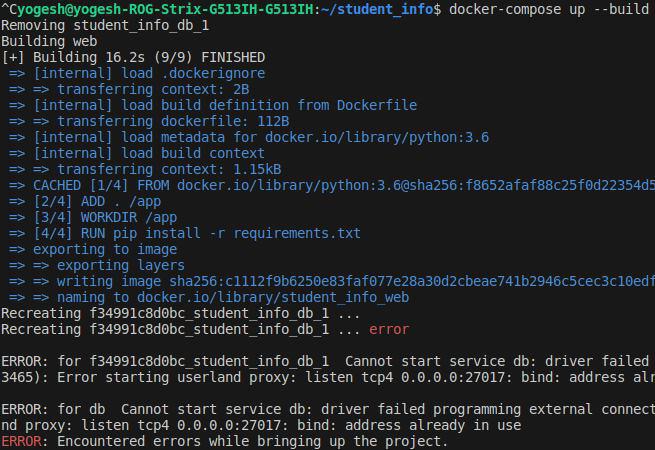
Then change the port number from 27017 to 27018 in the docker-compose.yml:
ports:
– 27018:27017
This error will get resolved.
4. Run Web Application on Browser
Go to Flask server URL: http://172.17.0.3:5000/ this URL is not same for every system. It changes according to system’s IP address and port number where Flask application is running.
Test the Web application:
.gif)
Running Web application
All set, our Dockerized Full Stack Flask Web application with Database MongoDB is up and running.
FAQs on Dockerizing a Python Flask App with MongoDB
1. Can I deploy it on cloud?
Yes, you can deploy it on cloud but there are some complex processes of configuration of cloud providers platform like Orchestration, Load balancing, Auto Scaling, etc.
2. Should I rebuild the Docker image if I made any modifications to the Flask application?
Yes, you need to rebuild the Docker image you make any changes or modifications to your code. using “docker-compose up -d” this command you can rebuild docker image.
3. Why docker is used in this application?
Because Docker can run the web app independent of system environment by containerizing the web app.
4. Does Docker demand additional resources?
Yes, due to its containerization of application. but Docker offers more security due to its isolation environment.
5. What are benefits of using docker?
Docker provides more security, portability, reduces dependencies, making it easier to deploy, etc.
Share your thoughts in the comments
Please Login to comment...