Differentiate between worker threads and clusters in Node JS.
Last Updated :
14 Nov, 2023
In this article, we will learn about worker threads & clusters, along with discussing the significant distinction that differentiates between worker threads & clusters.
Let us first understand what is worker threads and clusters in Node.js?
What is Worker Thread in Node.JS?
Worker threads are the useful feature in Node.Js which allows us to run JavaScript code in parallel with the main thread. Before worker threads NodeJ.Js could execute one operation at a time. Worker threads provide the capability to perform parallel processing by creating separate threads.
Worker threads are useful for performing CPU-intensive JavaScript operations, but they don’t not help much with I/O intensive work. They are also able to share memory by transferring ArrayBuffer instances or sharing SharedArrayBuffer instances.
Creating a simple worker-thread
Example: Create two files named worker.js and index.js in your Node.Js project
Javascript
const { workerData, parentPort } = require( 'worker_threads' )
console.log( 'Worker Threads by ' + workerData);
parentPort.postMessage({ fileName: workerData, status: 'Done' })
|
Javascript
const { Worker } = require( 'worker_threads' )
function runService(workerData) {
return new Promise((resolve, reject) => {
const worker = new Worker(
'./worker.js' , { workerData });
worker.on( 'message' , resolve);
worker.on( 'error' , reject);
worker.on( 'exit' , (code) => {
if (code !== 0)
reject( new Error(
`Worker Thread stopped with the exit code: ${code}`));
})
})
}
async function run() {
const result = await runService( 'GeeksForGeeks' )
console.log(result);
}
run(). catch (err => console.error(err))
|
Output: Here, the function runService() return a Promise and runs the worker thread. The function run() is used for calling the function runService() and giving the value for workerData.

Simple Worker Thread example
What is clusters in Node.JS?
Node.Js clusters are used to run multiple instances in single threaded Node application. By using cluster module you can distribute workloads among application threads. The cluster module allows us to create child processes that all share server ports. To handle heavy load, we need to launch a cluster of Node.js processes and hence make use of multiple cores.
Each child process has its own event loop, memory, V8 instance, and shares the same server port.
Creating a simple cluster
Example: Write the following code to create a cluster
Javascript
const cluster = require( 'cluster' );
const os = require( 'os' );
const express=require( "express" );
const app=express();
if (cluster.isMaster) {
console.log(`Master ${process.pid} is running`);
for (let i = 0; i < os.cpus().length; i++) {
cluster.fork();
}
cluster.on( 'exit' , (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
cluster.fork();
});
} else {
console.log(`Worker ${process.pid} started`);
const port=8000;
app.listen(port,(req,res)=>{
console.log(`server running at port ${port}`);
});
}
|
Output:
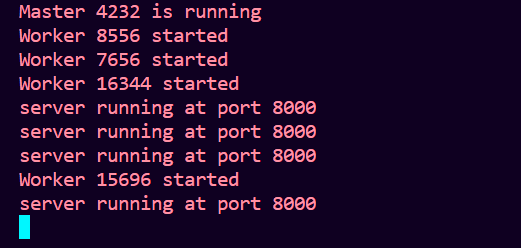
Simple Cluster example
Explanation: Here, The master process (identified by cluster.isMaster) forks multiple worker processes.Each worker process (identified by cluster.isWorker) runs independently and performs its own tasks.The master process listens for worker exit events and forks a new worker when one exits.
Difference between Worker threads and Clusters:
Through both Worker threads and Clusters are mechanisms for concurrent and parallel processing, but still they have different use cases and operate at different levels of abstraction that differentiates both Worker threads and Clusters, which is given below.
Worker threads operate at thread level, providing a way to run JavaScript code in parallel within a single process.
|
Clusters operate at process level, allowing you to create multiple Node.js processes (workers) to handle incoming network requests.
|
Communication between worker threads is typically achieved through message passing using the postMessage API.
|
Communication between the master process and worker processes is achieved using IPC mechanisms.
|
Worker threads have their own isolated JavaScript context, means they don’t share variables or memory directly.
|
Each worker process in a cluster is a separate Node.js process, which means they have their own memory space.
|
Worker threads are not built for I/O-intensive operations, as Node.js’s built-in asynchronous I/O mechanisms are often more efficient.
|
Clusters are built for handle I/O-intensive operations efficiently. Each worker in a cluster can handle incoming requests independently.
|
Worker threads can share memory using ArrayBuffer or SharedArrayBuffer instances, which allows more direct communication and shared data.
|
Clusters operate in separate processes so memory is isolated between them. Communication between clusters is often achieved through message passing.
|
Worker threads are good for CPU-intensive tasks where parallel processing can significantly improve performance.
|
Clusters are good for improving the scalability of networked applications by distributing incoming requests among multiple processes.
|
Conclusion:
The decision on whether to use worker threads, which used for parallelizing CPU-intensive tasks within single process, or Clusters, which are used to distribute operations across multiple processes utilizing the multi processor, for better scalability and dependability, depends on the particular usecase of the application.
Share your thoughts in the comments
Please Login to comment...