Diamond Problem in Java
Last Updated :
22 Sep, 2023
Diamond Problem is a problem faced during Multiple Inheritance in Java. Let’s see what is Diamond Problem and how to solve this problem.
What is a Diamond Problem in Java?
Inheritance in Java is when a child class inherits the properties of the parent class. There are certain types of inheritance in Java as mentioned below:
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
- Hybrid Inheritance
Talking about Multiple inheritance is when a child class is inherits the properties from more than one parents and the methods for the parents are same (Method name and parameters are exactly the same) then child gets confused about which method will be called. This problem in Java is called the Diamond problem.
Example of Java Diamond Problem
Below is the illustration of the Diamond Problem in Java:
Java
import java.io.*;
class Parent1 {
void fun() { System.out.println( "Parent1" ); }
}
class Parent2 {
void fun() { System.out.println( "Parent2" ); }
}
class test extends Parent1, Parent2 {
public static void main(String[] args)
{
test t = new test();
t.fun();
}
}
|
Output:
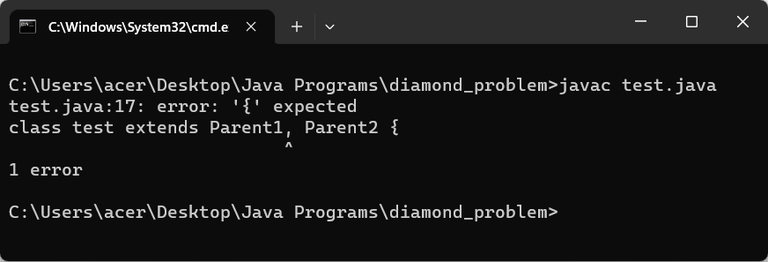
Explanation : In above example we have seen that “test” class is extending two classes “Parent1 ” and “Parent2” and its calling function “fun()” which is defined in both parent classes so now here it got confused which definition it should inherit.
Solution of Diamond Problem in Java
Although Diamond Problem is a serious issue but we can create a solution for it which is Interface. Interface are created by using interface keyword. It contains all methods by default as abstract we don’t need to declared as abstract ,compiler will do it implicitly. We can’t instantiate interface for this we have to use a class which will implement the interface and will write the definitions of its all functions.
Here below we will see , how can we implement multiple inheritance by interface.
Java
import java.io.*;
interface Parent1 {
void fun();
}
interface Parent2 {
void fun();
}
class test implements Parent1, Parent2 {
public void fun()
{
System.out.println( "fun function" );
}
}
class test1 {
public static void main(String[] args)
{
test t = new test();
t.fun();
}
}
|
Conclusion
We are able to implement multiple inheritance through interface just because in case of interface we does not provide the definition of function and when class implement that function then it write definition of function only once.
Share your thoughts in the comments
Please Login to comment...