Dependency Injection(DI) Design Pattern
Last Updated :
12 Jan, 2024
Dependency Injection is one of the most widely used design patterns. It comes under the Software Design. In this article, we will discuss dependency injection with examples, why we need it, what is the use of dependency injection, advantages and disadvantages of dependency injection.
Important Topics for the Dependency Injection(DI) Design Pattern
What is the Dependency Injection Method Design Pattern?
In software design, dependency injection (DI) is a design pattern that aims to decouple objects from their dependencies. Instead of creating their own dependencies internally, objects receive them from an external source.
Here’s an explanation of dependency injection with a practical example:
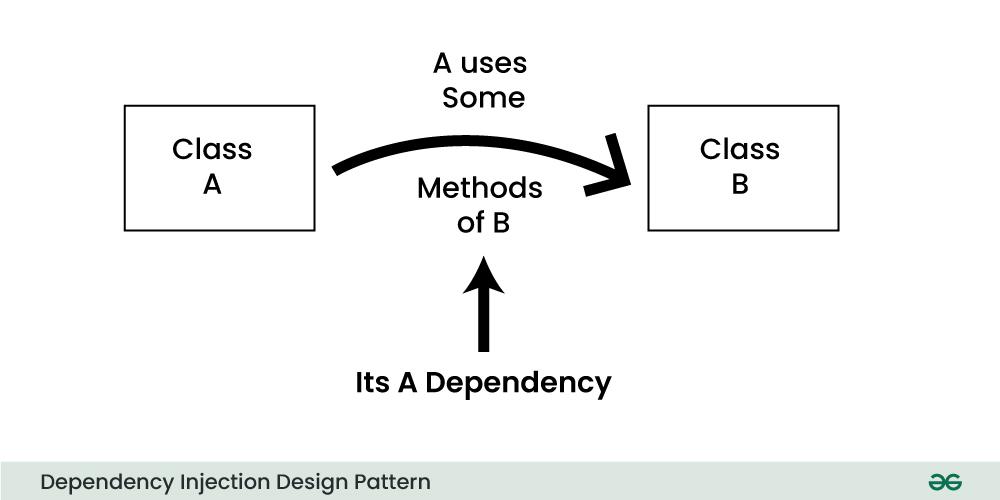
Imagine a coffee shop:
- The barista (object) class A needs coffee beans class B (dependency) to make coffee.
- Without dependency injection, the barista would have to grow, roast, and grind the beans themselves.
- This makes them tightly coupled to the bean-growing process, making it hard to change bean suppliers or use different roasts.
Four Roles of Dependency Injection
In Dependency Injection, the dependencies of a class are injected from the outside, rather than the class creating or managing its dependencies internally. This pattern has four main roles:
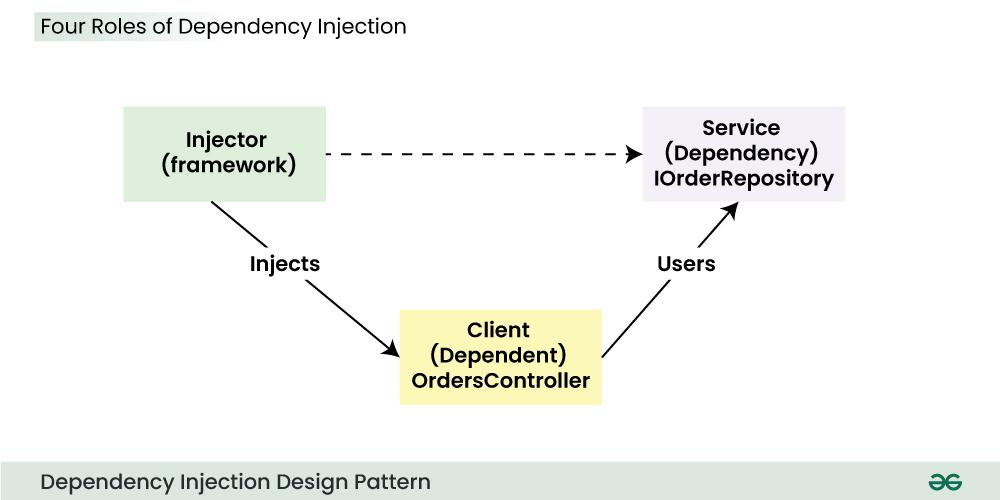
- Client:
- The client is the component or class that depends on the services provided by another class or module.
- It does not create or manage the dependencies itself but relies on external entities to provide those dependencies.
- Service:
- The service is the component or class that provides a particular functionality or service that the client depends on.
- It is designed to be independent of the clients and focuses on providing specific functionality.
- Injector:
- The injector is responsible for creating instances of services and injecting them into the client.
- It is aware of the dependencies of the client and provides the necessary services during runtime.
- Interface:
- The interface defines the contract or set of methods that a service must implement.
- Clients rely on these interfaces rather than specific implementations, promoting flexibility and the ability to swap implementations.
When to use Dependency Injection Design Pattern?
Here are the key scenarios where dependency injection is a valuable approach:
- Loose Coupling and Reusability:Objects don’t create their own dependencies, breaking tight connections and making them more independent.
- Testability: Inject mock or test doubles for dependencies, allowing you to test individual objects in isolation without relying on external systems or services.
- Maintainability and Flexibility: Dependency injection frameworks often manage dependencies, making it easier to track and configure them.
- Scalability and Extensibility: In large-scale applications, DI helps manage complex dependency graphs and enables easier scaling and extension. Add new features or modify existing ones without significant code refactoring, as dependencies can be easily injected or replaced.
- Cross-Cutting Concerns: Inject services for logging, security, caching, or other cross-cutting concerns that are used across multiple components, avoiding code duplication and promoting a consistent approach.
Example for Dependency Injection Design Pattern
Problem Statement:
Imagine you’re building an application that sends notifications to users. You want to make the notification system flexible so you can change the notification provider (email, SMS, push notifications, etc.) without modifying the core application logic.
Code Without Dependency Injection:
Java
public class NotificationService {
private EmailProvider emailProvider = new EmailProvider();
public void sendNotification(String message, String recipient) {
emailProvider.sendEmail(message, recipient);
}
}
|
Issues:
- Tight Coupling:Â TheÂ
NotificationService
 is tightly coupled to the EmailProvider
, making it difficult to switch to a different provider without code changes.
- Testability:Â TestingÂ
NotificationService
 in isolation is challenging as it directly uses EmailProvider
.
Code With Dependency Injection:
Java
public interface NotificationProvider {
void sendNotification(String message, String recipient);
}
public class EmailProvider implements NotificationProvider {
@Override
public void sendNotification(String message, String recipient) {
}
}
public class SMSProvider implements NotificationProvider {
@Override
public void sendNotification(String message, String recipient) {
}
}
public class NotificationService {
private NotificationProvider notificationProvider;
public NotificationService(NotificationProvider notificationProvider) {
this .notificationProvider = notificationProvider;
}
public void sendNotification(String message, String recipient) {
notificationProvider.sendNotification(message, recipient);
}
}
|
Benefits of using Dependency Injection Design Pattern in this solution above:
- Loose Coupling:Â
NotificationService
 no longer depends on a specific implementation, making it adaptable to different providers.
- Testability:Â You can easily inject mock providers for testingÂ
NotificationService
 in isolation.
- Flexibility:Â You can change the notification provider at runtime by configuring the injection mechanism.
- Maintainability:Â Code becomes more modular and easier to manage as dependencies are explicit.
Advantages of using Dependency Injection Design Pattern
Dependency injection offers a plethora of benefits for your software development. Here are some key advantages:
- Increased Modularity and Maintainability:
- Code becomes cleaner and more modular by decoupling components from their dependencies.
- Components rely on abstract interfaces or base classes, not concrete implementations, making them easier to change and test.
- Changes in one component’s dependencies don’t ripple through the entire system, simplifying maintenance.
- Improved Testability:
- Mocks and stub dependencies can be easily injected for unit testing, facilitating isolated testing of components.
- This leads to more reliable and efficient test suites.
- Reduced Coupling and Improved Loose Coupling:
- Components depend on abstractions, not specific implementations, promoting loose coupling.
- This makes the system more flexible and adaptable to changes.
- Easier Collaboration and Reusability:
- Developers can focus on implementing core functionalities without worrying about dependencies.
- Components with injected dependencies can be easily reused in different contexts.
- Encourages Loose Coupling and Promotes Dependency Management:
- Dependency injection frameworks can manage dependencies across the application, preventing dependency conflicts and ensuring version compatibility.
Disadvantages of using Dependency Injection Design Pattern
While undeniable benefits come with dependency injection (DI), it’s essential to acknowledge potential downsides and consider them in your software development decisions. Here are some key disadvantages to be aware of:
- Increased Complexity:
- Introducing DI frameworks or managing manual injection can add complexity to smaller projects or simple codebases.
- Understanding and applying DI concepts necessitates an initial learning curve for developers.
- Runtime Errors:
- Improper configuration or injection of incompatible dependencies can lead to runtime errors that are harder to debug than compile-time errors in tightly coupled code.
- Overhead and Performance:
- DI frameworks can introduce additional overhead in terms of memory usage and runtime performance, especially compared to tightly coupled architectures.
- Testing Dependency Injection Itself:
- Testing your DI configuration and its interactions with injected dependencies can be more challenging than testing directly coupled components.
- Debugging Challenges:
- Tracing the flow of execution and dependencies in a DI-based system can be more complex than in statically linked systems, making debugging more intricate.
- Increased Abstraction and Reduced Transparency:
- Heavy reliance on abstractions can remove direct visibility into how components interact, potentially lowering code intelligibility for some developers.
Also read: Java Dependency Injection (DI) Design Pattern
Share your thoughts in the comments
Please Login to comment...