Create a Video Streaming App using React-Native
Last Updated :
25 Dec, 2023
React-Native is an open-source JavaScript framework used to broaden cross-platform packages i.e., you may write code in React-Native and publish it as an Android or IOS app. In this article, we will build a Video Streaming app using React-Native. The app will run on both Android and IOS.
Preview of final output: Let us have a look at how the final application will look like.
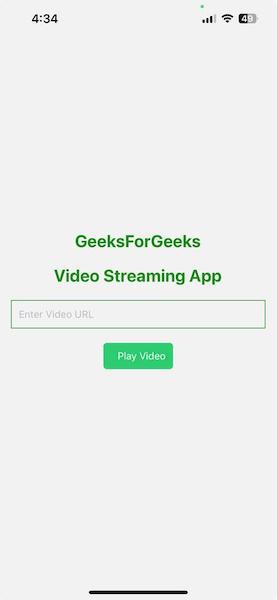
Video Streaming App
Prerequisites
Approach to create Video Streaming App:
In the app, we use two screens. Home Screen and Video Player Screen. The home screen takes the video URL and plays it in the video player screen. To play the video we use the expo-av package. You can input any mp4 video URL that is live in the internet. The app will fetch the URL and play the video. This is how our basic video streaming app works.
Steps to Create Video Streaming App:
Step 1: Create a React Native app by using this command:
npx create-expo-app VideoStreamingApp
Step 2: Navigate to our project through this command:
cd VideoStreamingApp
Step 3: Install the required dependencies using the following command
npm install @react-navigation/native @react-navigation/stack expo-av
Project Structure:
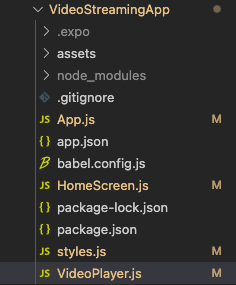
Project Structure
The updated dependencies in package.json will look like this
"dependencies": {
"expo-av": "~13.2.1",
"expo-camera": "~13.2.1",
"expo-status-bar": "~1.4.4",
"expo-permissions": "~14.1.1",
"@expo/vector-icons": "^13.0.0",
"expo-media-library": "~15.2.3",
"react-native-paper": "4.9.2",
"expo-document-picker": "~11.2.2",
"react-native-screens": "~3.20.0",
"@react-navigation/stack": "^6.3.20",
"@react-navigation/native": "6.0.0",
"react-native-gesture-handler": "~2.9.0",
"@react-navigation/bottom-tabs": "^6.5.11",
"react-native-safe-area-context": "4.5.0"
}
Example: Write the code in respective files
Javascript
import React from 'react' ;
import { NavigationContainer } from '@react-navigation/native' ;
import { createStackNavigator } from '@react-navigation/stack' ;
import HomeScreen from './HomeScreen' ;
import VideoPlayer from './VideoPlayer' ;
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator
initialRouteName= "Home"
screenOptions={{ headerShown: false }}
>
<Stack.Screen name= "Home" component={HomeScreen} />
<Stack.Screen name= "VideoPlayer" component={VideoPlayer} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
|
Javascript
import React, { useState } from 'react' ;
import { View, Text, TextInput, Pressable } from 'react-native' ;
import { styles } from './styles' ;
const HomeScreen = ({ navigation }) => {
const [videoUrl, setVideoUrl] = useState( '' );
const handlePlayVideo = () => {
navigation.navigate( 'VideoPlayer' , { videoUrl });
};
return (
<View style={styles.container}>
<Text style={styles.heading}>GeeksForGeeks</Text>
<Text style={styles.heading}>Video Streaming App</Text>
<TextInput
style={styles.input}
placeholder= "Enter Video URL"
onChangeText={(url) => setVideoUrl(url)}
value={videoUrl}
/>
<Pressable
onPress={handlePlayVideo}
style={({ pressed }) => [
styles.button,
{
backgroundColor: pressed ? '#1e8449' : '#2ecc71' ,
},
]}
>
<Text style={styles.buttonText}>Play Video</Text>
</Pressable>
</View>
);
};
export default HomeScreen;
|
Javascript
import React from 'react' ;
import { View, Text } from 'react-native' ;
import { Video } from 'expo-av' ;
import { styles } from './styles' ;
const VideoPlayer = ({ route }) => {
const { videoUrl } = route.params;
return (
<View style={styles.container}>
<Text style={styles.heading}>Video Player</Text>
<Video
source={{ uri: videoUrl }}
style={styles.video}
useNativeControls
/>
</View>
);
};
export default VideoPlayer;
|
Javascript
import { StyleSheet } from "react-native" ;
export const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
padding: 16,
},
heading: {
fontSize: 24,
fontWeight: 'bold' ,
marginBottom: 20,
color: '#228B22' ,
},
input: {
height: 40,
width: '100%' ,
borderColor: '#228B22' ,
borderWidth: 1,
marginBottom: 20,
paddingLeft: 10,
},
button: {
flexDirection: 'row' ,
alignItems: 'center' ,
backgroundColor: '#228B22' ,
padding: 10,
borderRadius: 5,
},
buttonText: {
color: 'white' ,
marginLeft: 10,
},
video: {
width: 300,
height: 300,
},
});
|
You can use any of the below two methods to run the app
Method 1: Open the terminal and enter the following command to run the app
npx expo start
Now download the Expo Go app from the Apple App Store (For iOS) or Google Play Store (For Android) to run the app.
Method 2: You can use emulator or connect computer to your device using USB and run the below command
npx react-native run-android
npx react-native run-ios
Output:
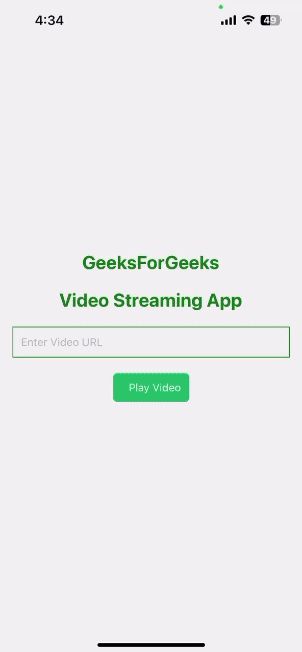
Video Streaming App
Share your thoughts in the comments
Please Login to comment...