Create a Video Player App using React-Native
Last Updated :
25 Dec, 2023
React-Native is an open-source JavaScript framework used to broaden cross-platform packages i.e., you may write code in React-Native and publish it as an Android or IOS app. In this article, we will build a Video Player app with the usage of React-Native. The app will run on both Android and IOS.
Preview of the Final Output: Let us have a look at how the final application will look like.
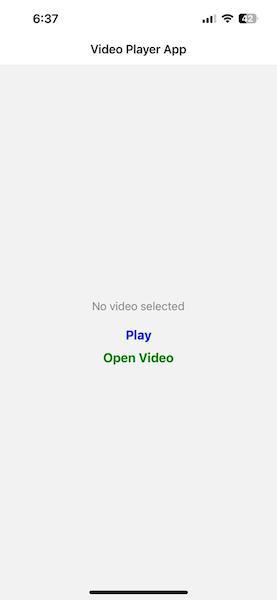
Video Player App
Prerequisites
Approach to create Video PLayer App:
The app contains two buttons Play/Pause button and Open Video button. We use expo-document-picker package to select the vide file. We mention type as mp4 so that only mp4 files are selected. This can be called using the function DocumentPicker.getDocumentAsync({ type: ‘video/mp4’ }); For playing the video, we use expo-av package. The basic video player app can play any mp4 video.
Steps to create our Video Player App
Step 1: Create a React Native app by using this command:
npx create-expo-app VideoPlayerApp
Step 2: Navigate to our project through this command:
cd VideoPlayerApp
Step 3: To add the required dependencies use the below command :
npm install @react-navigation/native @react-navigation/stack expo-av expo-document-picker
Project Structure:
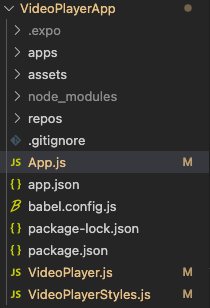
Project Structure
The updated dependencies in package.json file will look like this:
"dependencies": {
"expo-av": "~13.2.1",
"expo-camera": "~13.2.1",
"expo-status-bar": "~1.4.4",
"expo-permissions": "~14.1.1",
"@expo/vector-icons": "^13.0.0",
"expo-media-library": "~15.2.3",
"react-native-paper": "4.9.2",
"expo-document-picker": "~11.2.2",
"react-native-screens": "~3.20.0",
"@react-navigation/stack": "^6.3.20",
"@react-navigation/native": "6.0.0",
"react-native-gesture-handler": "~2.9.0",
"@react-navigation/bottom-tabs": "^6.5.11",
"react-native-safe-area-context": "4.5.0"
}
Example: Write the following code in the respective files:
Javascript
import React from 'react' ;
import { NavigationContainer } from '@react-navigation/native' ;
import { createStackNavigator } from '@react-navigation/stack' ;
import VideoPlayer from './VideoPlayer' ;
const Stack = createStackNavigator();
const App = () => {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name= "Video Player App" component={VideoPlayer} />
</Stack.Navigator>
</NavigationContainer>
);
};
export default App;
|
Javascript
import React, { useState, useEffect } from 'react' ;
import { View, TouchableOpacity, Text } from 'react-native' ;
import { Video } from 'expo-av' ;
import * as DocumentPicker from 'expo-document-picker' ;
import VideoPlayerStyles from './VideoPlayerStyles' ;
const styles = VideoPlayerStyles;
const VideoPlayer = () => {
const [videoStatus, setVideoStatus] = useState({});
const [videoUri, setVideoUri] = useState( null );
const videoRef = React.useRef( null );
useEffect(() => {
const currentVideoRef = videoRef.current;
return () => {
videoStatus.isPlaying && currentVideoRef && currentVideoRef.pauseAsync();
};
}, [videoStatus]);
const togglePlayPause = async () => {
if (videoRef.current) {
if (videoStatus.isPlaying) {
await videoRef.current.pauseAsync();
} else {
await videoRef.current.playAsync();
}
setVideoStatus((prevStatus) => ({ isPlaying: !prevStatus.isPlaying }));
}
};
const openVideoPicker = async () => {
try {
const result = await DocumentPicker.getDocumentAsync({ type: 'video/*' });
if (result.type === 'success' && result.uri) {
setVideoUri(result.uri);
setVideoStatus({ isPlaying: false });
}
} catch (error) {
console.error( 'Error picking video:' , error);
}
};
return (
<View style={styles.container}>
{videoUri ? (
<Video
ref={videoRef}
source={{ uri: videoUri }}
style={styles.video}
resizeMode= "contain"
shouldPlay={videoStatus.isPlaying}
isLooping
useNativeControls
/>
) : (
<Text style={styles.noVideoText}>No video selected</Text>
)}
<TouchableOpacity onPress={togglePlayPause} disabled={!videoUri}>
<Text style={styles.playPauseButton}>{videoStatus.isPlaying ? 'Pause' : 'Play' }</Text>
</TouchableOpacity>
<TouchableOpacity onPress={openVideoPicker}>
<Text style={styles.openVideoButton}>Open Video</Text>
</TouchableOpacity>
</View>
);
};
export default VideoPlayer;
|
Javascript
import { StyleSheet } from 'react-native' ;
export default VideoPlayerStyles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center' ,
alignItems: 'center' ,
},
video: {
width: 300,
height: 200,
marginBottom: 20,
},
noVideoText: {
fontSize: 16,
color: 'gray' ,
marginBottom: 20,
},
playPauseButton: {
fontSize: 18,
color: 'blue' ,
fontWeight: 'bold' ,
marginBottom: 10,
},
openVideoButton: {
fontSize: 18,
color: 'green' ,
fontWeight: 'bold' ,
},
});
|
You can use any of the below two methods to run the app
Method 1: Open the terminal and enter the following command to run the app
npx expo start
You can download the Expo Go app from the Apple App Store (For iOS) or Google Play Store (For Android) to run the app.
Method 2: You can use emulator or connect computer to your device using USB and run the below command
npx react-native run-android
npx react-native run-ios
Output:
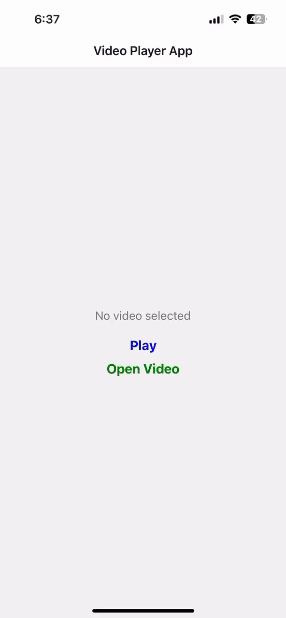
Video Player App
Share your thoughts in the comments
Please Login to comment...