Create A File If Not Exists In Python
Last Updated :
19 Feb, 2024
In Python, creating a file if it does not exist is a common task that can be achieved with simplicity and efficiency. By employing the open()
function with the ‘x’ mode, one can ensure that the file is created only if it does not already exist. This brief guide will explore the concise yet powerful approach to creating a file in Python, emphasizing simplicity and effectiveness in file management.
How To Create A File If Not Exist in Python?
below, are the methods of How To Create A File If Not Exists In Python.
Example 1: Using open() Function with ‘x’ Mode
In this example, This Python code attempts to create a new file named “example.txt” and writes “Hello, Geeks!” to it. If the file already exists, a `FileExistsError` exception is caught, and a message is printed indicating that the file already exists.
Python3
file_path = "example.txt"
try :
with open (file_path, 'x' ) as file :
file .write( "Hello, Geeks!" )
except FileExistsError:
print (f "The file '{file_path}' already exists." )
|
Output
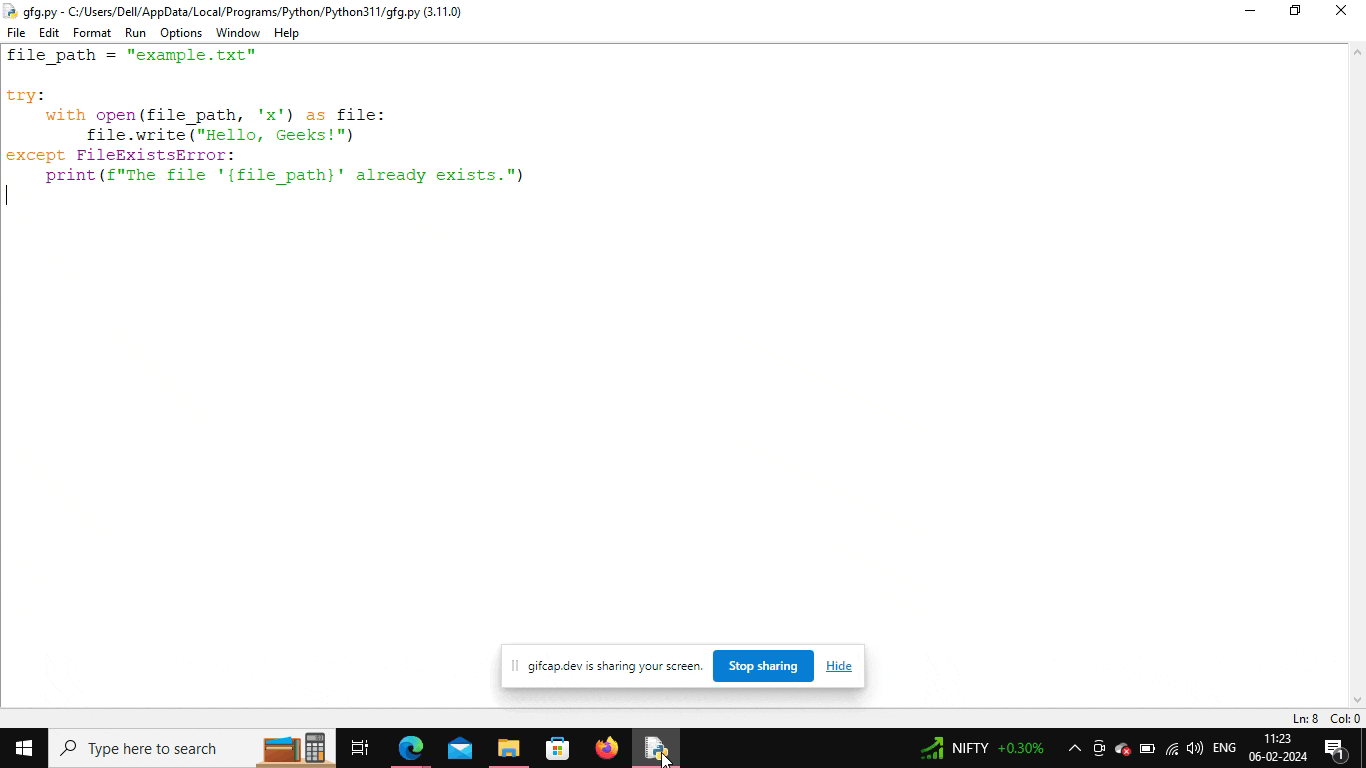
Example 2: Using os.path Module
In this example, This Python code checks if a file named “example.txt” exists using `os.path.exists()`. If the file does not exist, it creates the file and writes “Hello, Geeks!” to it. If the file already exists, it prints a message indicating that the file is already present.
Python3
import os
file_path = "example.txt"
if not os.path.exists(file_path):
with open (file_path, 'w' ) as file :
file .write( "Hello, Geeks!" )
else :
print (f "The file '{file_path}' already exists." )
|
Output
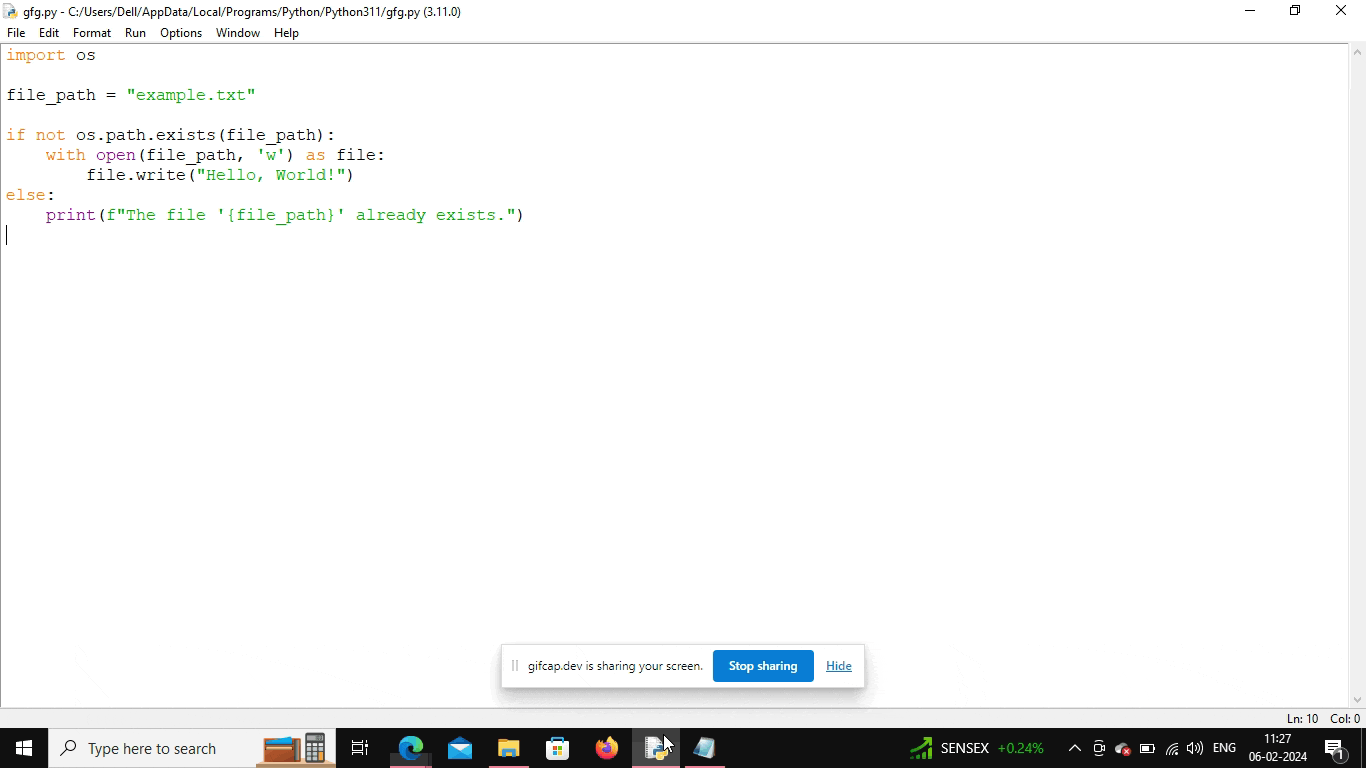
Example 3: Using Path Class From pathlib Module
In this example, below Python code utilizes the `pathlib` module to achieve the same goal. It checks if a file named “example.txt” exists, and if not, creates the file and writes “Hello, Geeks!” to it. If the file already exists, it prints a message indicating that the file is already present. The `Path` class simplifies file path handling and operations.
Python3
from pathlib import Path
file_path = Path( "example.txt" )
if not file_path.exists():
with open (file_path, 'w' ) as file :
file .write( "Hello, Geeks!" )
else :
print (f "The file '{file_path}' already exists." )
|
Output:
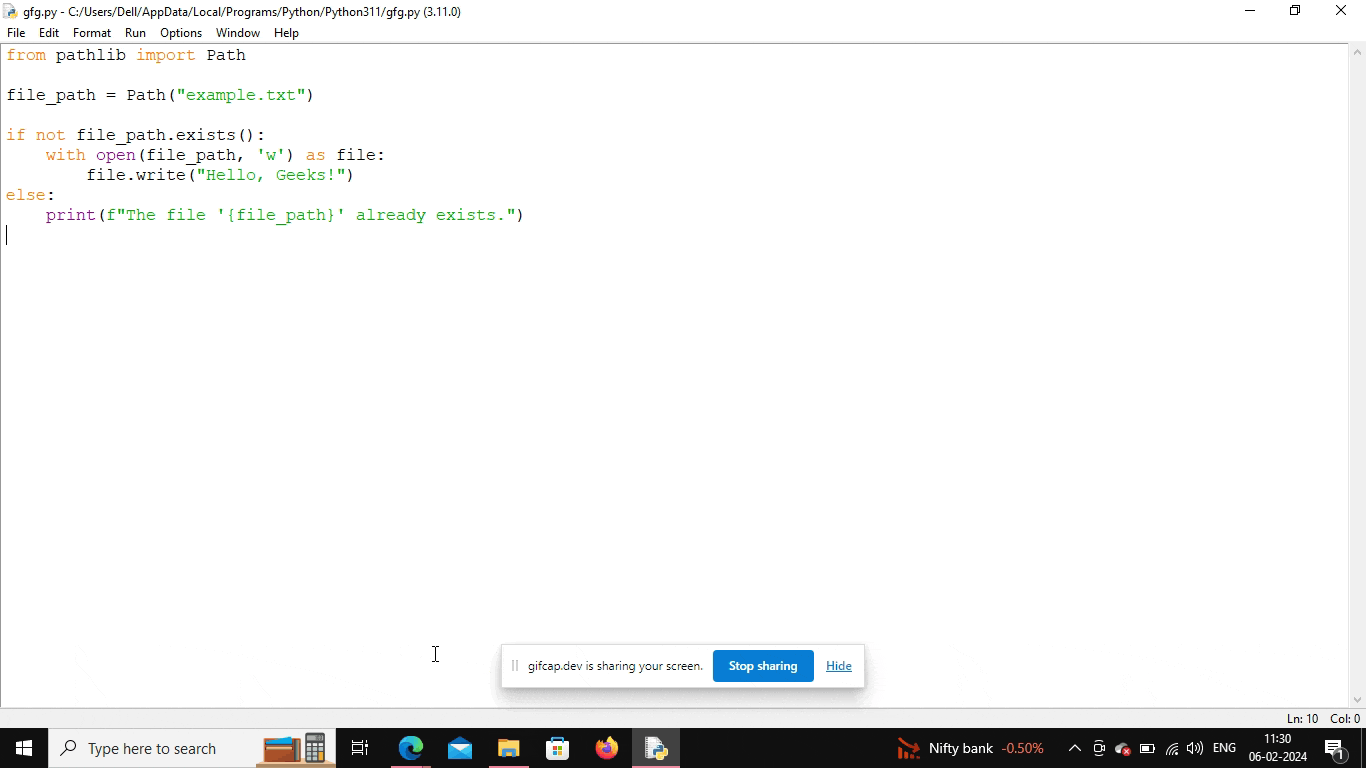
Conclusion
In conclusion, creating a file if it does not exist in Python is a straightforward process. Leveraging the ‘x’ mode in the `open()` function allows for the seamless creation of files while avoiding potential conflicts with existing ones. This concise technique enhances file management, offering a robust solution for ensuring the presence of a file before further operations.
Share your thoughts in the comments
Please Login to comment...