C++ Program For Radix Sort
Last Updated :
25 Oct, 2023
Radix Sort is a sorting technique in which we sort the elements by processing each and every digit of that element. It is not a comparison-based sorting algorithm which means we do not compare the elements in a radix sort in order to sort them. Here, we apply counting sort to every digit of an element starting from the Least Significant Digit (LSB) to the Most Significant Digit (MSB) or vice versa.
Prerequisite: Counting Sort
Algorithm for Radix Sort in C++
The Radix Sort is based on the idea that by sorting the dataset on the basis of each decimal place, it will be eventually completely sorted. Radix sort uses the counting sort algorithm as the subroutine.
The algorithm of the radix sort can be written as:
arr[] = {a1, a2, a3 ... an};
for (each decimal place) {
countingSort(arr);
}
Working of Radix Sort in C++
We will understand the working of Radix Sort in C++ using an example.
Let’s first take an array named ‘original_arr’ = {21, 300, 8, 92, 654, 100, 252, 333, 693, 18};
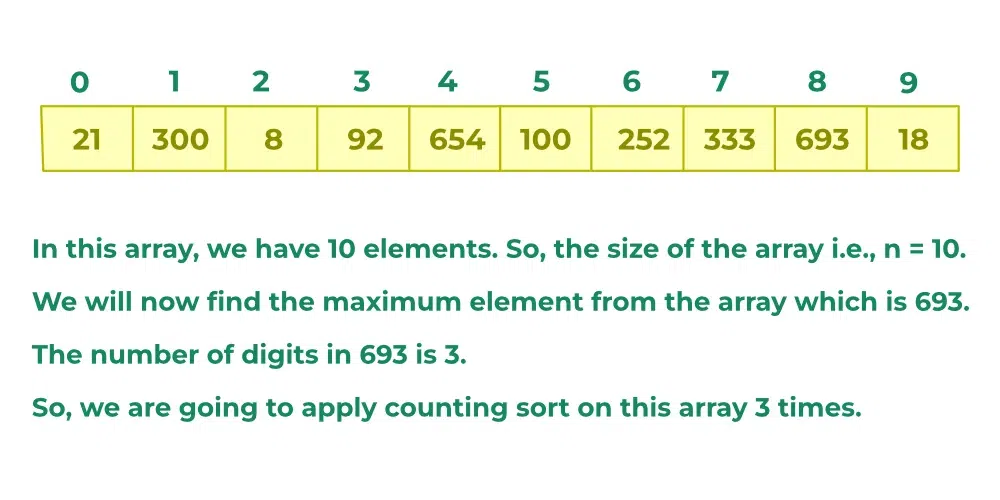
Original Array original-arr[]
Now, as the number of digits in the maximum element is 3 therefore we will represent all the elements of the array as 3-digit numbers.
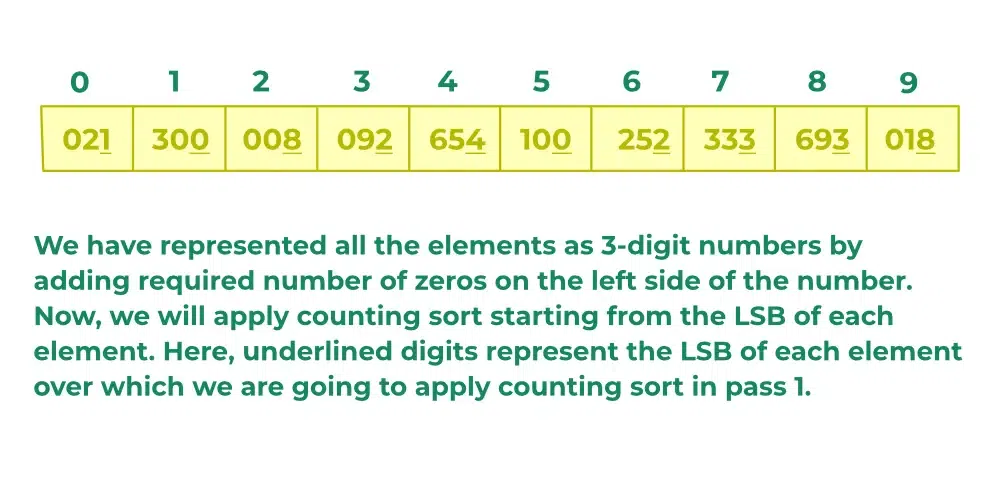
Prefixing Required Zeroes to the Elements of Original Array
Pass 1
We take an array named ‘count’ whose size is 10 as the values will range from 0-9 always. Initially, we will initialize the count array with 0. Then we will update the count array with respect to the frequency of the digits. After that, we will perform prefix sum in the same way we do in a counting sort.
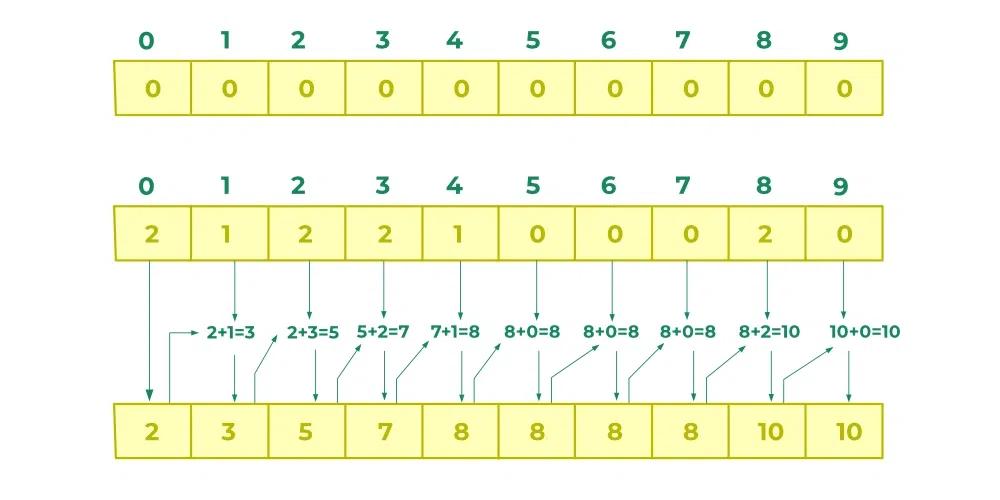
count Array in Pass 1
Now, we will find the final array after Pass 1 in the same way we did the counting sort. Let us name the final array as ans_arr.
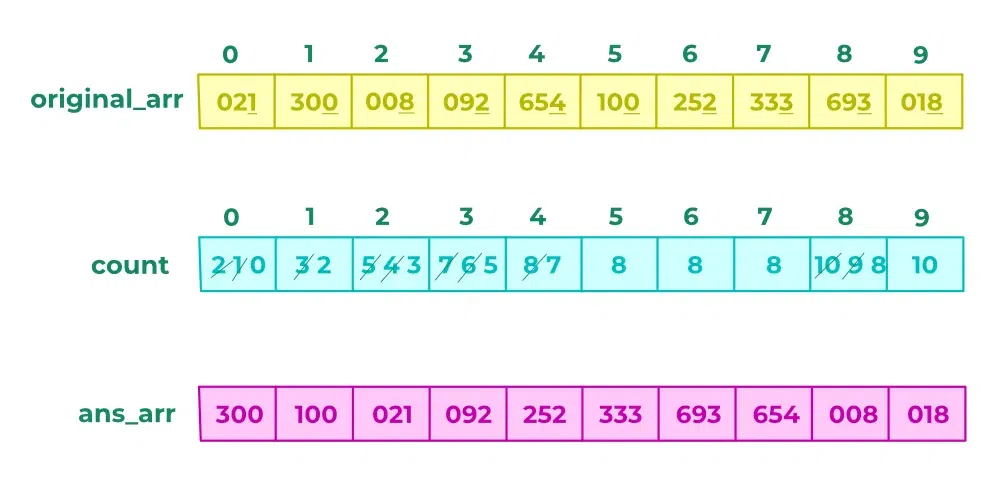
Array after Radix Sort Pass 1
For Pass 2
Now, we will take the ans_arr of Pass 1 as the original_arr for Pass 2 and continue the same steps of counting sort just like Pass 1. But with the difference we will consider the digits at second place.
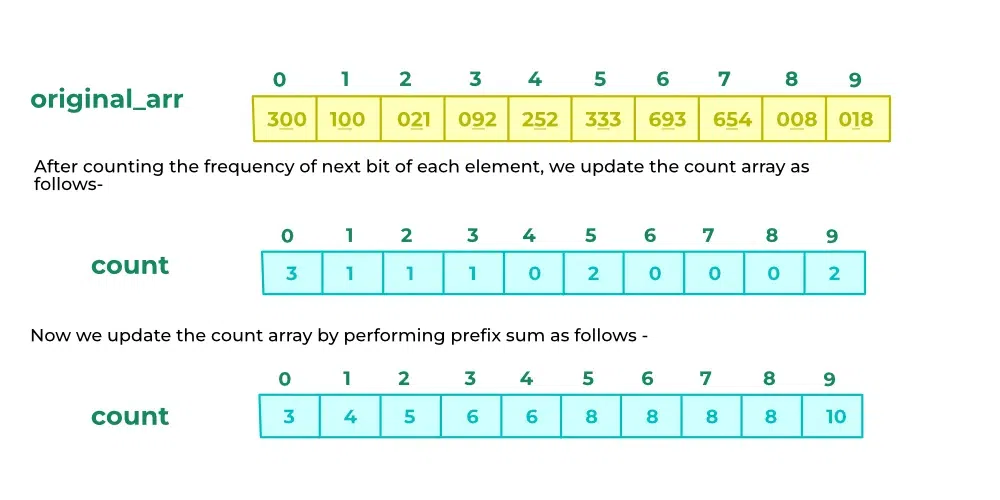
Count Array in Pass 2
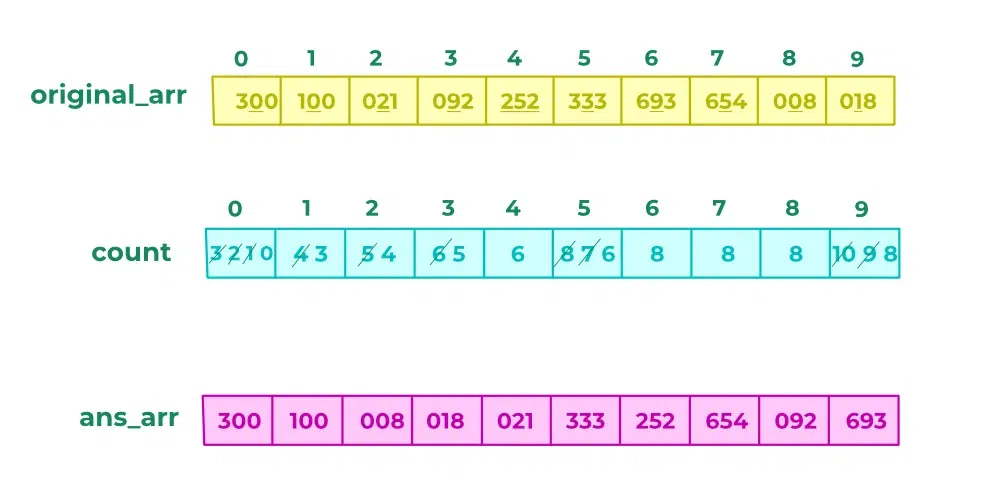
Array after Radix Sort Pass 2
For Pass 3
Now, we will take the ans_arr of Pass 2 as the original_arr for Pass 3 and continue the same steps of counting sort just like Pass 1 and Pass 2 but again increase the place of the digits i.e. we will consider
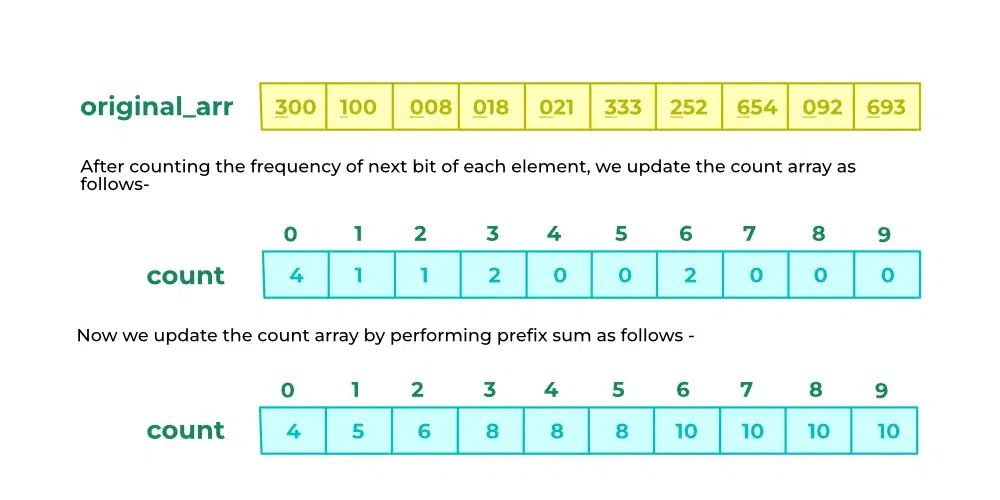
Count Array in Pass 3
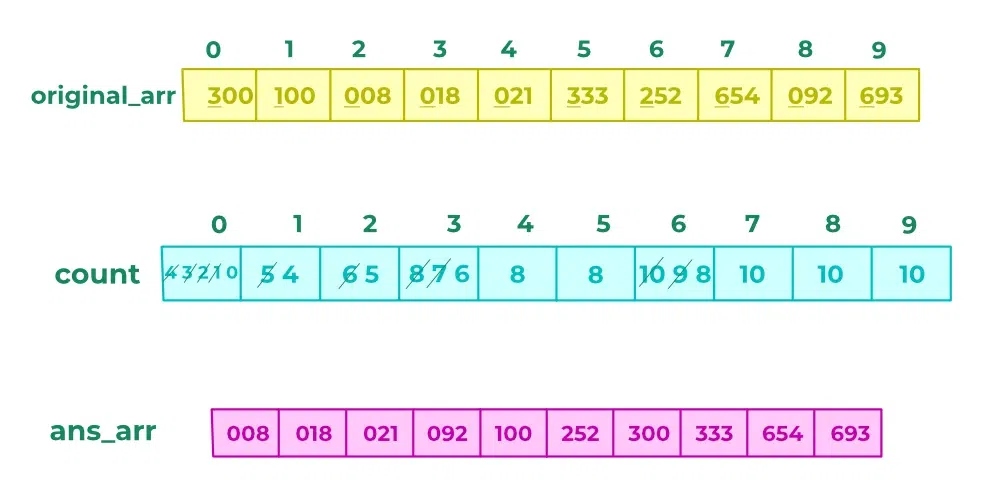
Array after Radix Sort Final Pass
The ans_arr that we get after Pass 3 is our final array after performing the Radix sort.
C++ Program for Radix Sort
C++
#include <bits/stdc++.h>
using namespace std;
void count_sort( int arr[], int n, int pos)
{
int count[10] = { 0 };
for ( int i = 0; i < n; i++) {
count[(arr[i] / pos) % 10]++;
}
for ( int i = 1; i < 10; i++) {
count[i] = count[i] + count[i - 1];
}
int ans[n];
for ( int i = n - 1; i >= 0; i--) {
ans[--count[(arr[i] / pos) % 10]] = arr[i];
}
for ( int i = 0; i < n; i++) {
arr[i] = ans[i];
}
}
void radix_sort( int arr[], int n)
{
int k = *max_element(arr, arr + n);
for ( int pos = 1; (k / pos) > 0; pos *= 10) {
count_sort(arr, n, pos);
}
}
int main()
{
int arr[] = { 6, 210, 300, 600, 1, 3 };
int n = sizeof (arr) / sizeof (arr[0]);
radix_sort(arr, n);
cout << "Array after performing radix sort : " << endl;
for ( int i = 0; i < n; i++) {
cout << arr[i] << " " ;
}
return 0;
}
|
Output
Array after performing radix sort :
1 3 6 210 300 600
Complexity Analysis of Radix Sort
- Time Complexity of Radix Sort: O(d*(n + k)).
- Space Complexity of Radix Sort: O(n + k).
where d is the no. of digits, n is the total no. of elements and k is the base of the number system.
Benifits of Radix Sort
The radix sort has the following benefits:
- It is faster than other comparison-based sorting algorithms.
- It is a stable sort.
Limitations of Radix Sort:
The radix sort also has some limitations which are as follows:
- Radix sort is inefficient for sorting small data sets.
- Space Complexity is higher as compared to the other sorting algorithms.
- Processing negative numbers requires extra steps.
Share your thoughts in the comments
Please Login to comment...