Count unvisited leaves after Frog Jumps
Last Updated :
04 Jan, 2024
Given an array arr[] of size N, an integer K representing the number of leaves in the pond, and the arr[i] indicating the strength of the ith frog’s jump, where 0 <= i < N. The frogs are at one end of the pond and are trying to get to the other end by jumping on the leaves, the task is to count the number of unvisited leaves. After each frog has reached the end while ensuring that every frog jumps with its respective strength.
Examples:
Input: N = 3 , K = 4 , arr [ ] = {3, 2, 4}Â
Output: 1
Explanation: leaf 2 will be visited by Frog 1, leaf 3 will be visited by frog 0, leaf 4 will be visited by Frog 1, and Frog 2, only leaf 1 remains unvisited.
Input: N = 3 , K = 6 , arr [ ] = {1, 3, 5}Â
Output: 0
Explanation: Frog 0 will visit all the leaves as it jumps a distance of 1, so no leaf remains unvisited.
Naive Approach: We can follow the below idea to solve the problem :
The problem states to find the count of leaves which are unvisited after all the N frogs have crossed the pond. For each frog i with strength strengthi we can iterate over the n leaves starting from 0 where the frog is currently present by jumping the frog from it’s present_leaf to present_leaf + strengthi (the next leaf it will jump on to), which will be the next present_leaf for this ith frog. Thus we can mark the leaves which are visited by this ith frog (the leaf with index that are perfectly divisible by strength of frog i). Similarly after iterating over all the frogs we marked all the leaves that were visited during the crossing of the frogs.
Follow the steps to solve the problem:
- Initialize the visited vector of size leaves+1, and mark them as false
- For each frog, iterate over all the leaves that the frog will visit, and mark that leaf as visited
- Iterate over all the leaves, and increase the answer count by 1 when an unvisited leaf is found
Below is the implementation for the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int unvisitedLeaves( int N, int leaves, int frogs[])
{
vector< bool > visited(leaves + 1, false );
for ( int i = 0; i < N; ++i) {
int strength_i = frogs[i];
for ( int j = 0; j <= leaves; j++) {
if (j % strength_i == 0)
visited[j] = true ;
}
}
int unvisitedLeavesCount = 0;
for ( int i = 0; i <= leaves; ++i) {
if (visited[i] == false ) {
unvisitedLeavesCount++;
}
}
return unvisitedLeavesCount;
}
int main()
{
int N = 3;
int leaves = 6;
int frogs[N] = { 3, 6, 2 };
cout << unvisitedLeaves(N, leaves, frogs) << endl;
return 0;
}
|
Java
import java.util.*;
public class GFG {
static int unvisitedLeaves( int N, int leaves, int [] frogs) {
boolean [] visited = new boolean [leaves + 1 ];
for ( int i = 0 ; i < N; ++i) {
int strength_i = frogs[i];
for ( int j = 0 ; j <= leaves; j++) {
if (j % strength_i == 0 )
visited[j] = true ;
}
}
int unvisitedLeavesCount = 0 ;
for ( int i = 0 ; i <= leaves; ++i) {
if (!visited[i]) {
unvisitedLeavesCount++;
}
}
return unvisitedLeavesCount;
}
public static void main(String[] args) {
int N = 3 ;
int leaves = 6 ;
int [] frogs = { 3 , 6 , 2 };
System.out.println(unvisitedLeaves(N, leaves, frogs));
}
}
|
Python
def unvisitedLeaves(N, leaves, frogs):
visited = [ False ] * (leaves + 1 )
for i in range (N):
strength_i = frogs[i]
for j in range (leaves + 1 ):
if j % strength_i = = 0 :
visited[j] = True
unvisitedLeavesCount = 0
for i in range (leaves + 1 ):
if not visited[i]:
unvisitedLeavesCount + = 1
return unvisitedLeavesCount
if __name__ = = "__main__" :
N = 3
leaves = 6
frogs = [ 3 , 6 , 2 ]
print (unvisitedLeaves(N, leaves, frogs))
|
C#
using System;
public class GFG
{
static int UnvisitedLeaves( int N, int leaves, int [] frogs)
{
bool [] visited = new bool [leaves + 1];
for ( int i = 0; i < N; ++i)
{
int strength_i = frogs[i];
for ( int j = 0; j <= leaves; j++)
{
if (j % strength_i == 0)
visited[j] = true ;
}
}
int unvisitedLeavesCount = 0;
for ( int i = 0; i <= leaves; ++i)
{
if (!visited[i])
{
unvisitedLeavesCount++;
}
}
return unvisitedLeavesCount;
}
public static void Main( string [] args)
{
int N = 3;
int leaves = 6;
int [] frogs = { 3, 6, 2 };
Console.WriteLine(UnvisitedLeaves(N, leaves, frogs));
}
}
|
Javascript
function GFG(N, leaves, frogs) {
const visited = new Array(leaves + 1).fill( false );
for (let i = 0; i < N; ++i) {
const strength_i = frogs[i];
for (let j = 0; j <= leaves; j++) {
if (j % strength_i === 0)
visited[j] = true ;
}
}
let unvisitedLeavesCount = 0;
for (let i = 0; i <= leaves; ++i) {
if (!visited[i]) {
unvisitedLeavesCount++;
}
}
return unvisitedLeavesCount;
}
const N = 3;
const leaves = 6;
const frogs = [3, 6, 2];
console.log(GFG(N, leaves, frogs));
|
Time Complexity: O(N.leaves)
Auxiliary Space: O(N)
Efficient Approach: To solve the problem using the Sieve Approach:
Since each frog is crossing the pond, there will be leaves that have been visited by another frog already. For those leaves, we do not need to traverse the ponds for such a frog for marking the leaves it visits as true, as they have already been marked true by a frog before it.
Let’s take an example: N = 3 , leaves = 6 , frogs = [ 3, 6, 2 ]
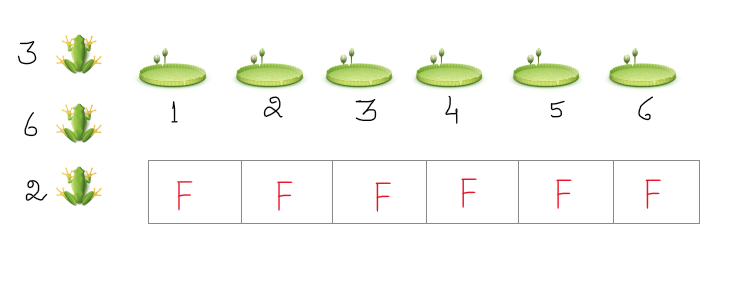
Let’s look for the first frog with strength 3 :
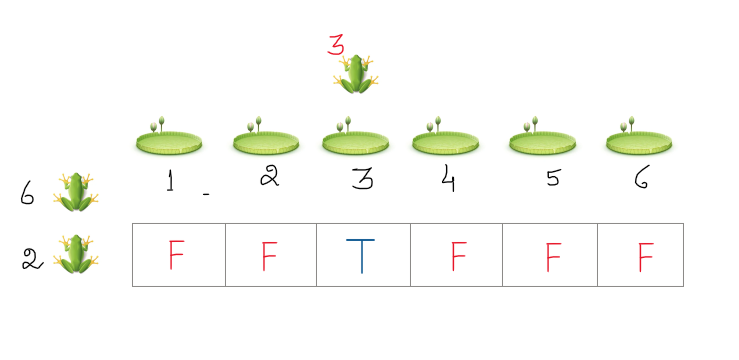
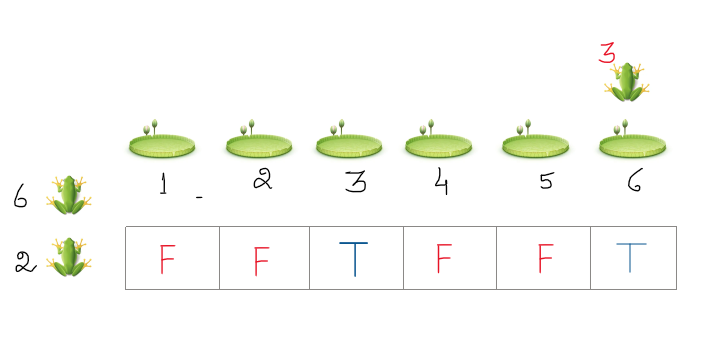
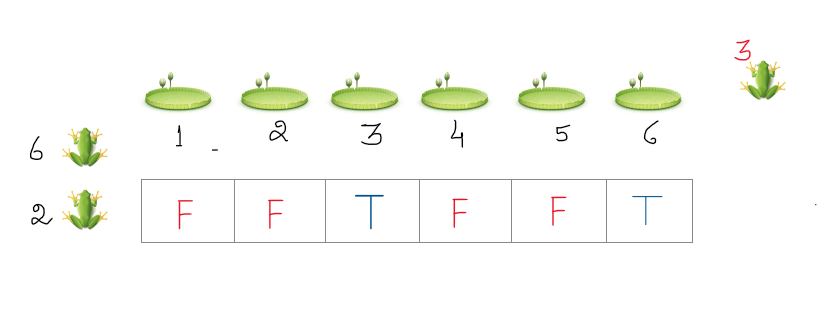
It jumps to 3, then 6, then so on until it reaches the other side of the pond, in this case after 6, in the next jump it reaches the other side.
Now for the next frog with strength 6:
the
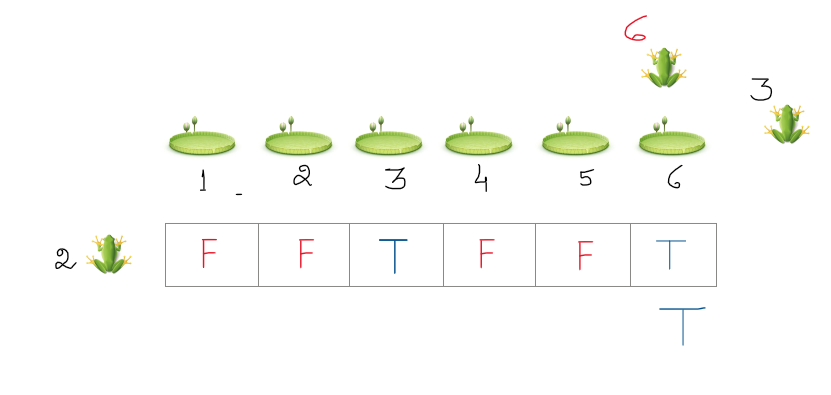
In the first jump, it goes to 6 and marks it as visited. Now if the leaves were more, we can observe that since 3 (strength of frog 1) is a factor of 6(strength of frog 2), frog 1 will visit all the leaves that frog 2 will visit. Thus we can skip the iteration for frog 2, and still mark all the leaves that were going to be visited.
Follow the steps to solve the problem:
- Sort Strength in increasing order
- Initialize the visited vector of size leaves+1, and mark them as false
- Initialize the count of unvisited leaves by the total number of leaves
- For each frog, check if the leaf jumped on after the first jump of the frog visited or not. (If in the first jump, it reaches the other end, skip this frog as well, as it will not visit any leaves). If it is visited, skip the iteration for this frog. Else, iterate over all the leaves this frog is going to, mark them as visited, and decrease the number of unvisited leaves by 1.
Below is the implementation for the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
int unvisitedLeaves( int N, int leaves, int frogs[])
{
vector< bool > visited(leaves + 1, false );
int unvisitedLeavesCount = leaves;
sort(frogs, frogs + N);
for ( int i = 0; i < N; ++i) {
int strength_i = frogs[i];
if (strength_i == 1)
return 0;
if (strength_i > leaves)
continue ;
if (visited[strength_i] == true )
continue ;
for ( int j = strength_i; j <= leaves;
j += strength_i) {
if (visited[j] == false )
unvisitedLeavesCount--;
visited[j] = true ;
}
}
return unvisitedLeavesCount;
}
int main()
{
int N = 3;
int leaves = 6;
int frogs[N] = { 3, 6, 2 };
cout << unvisitedLeaves(N, leaves, frogs) << endl;
return 0;
}
|
Java
import java.util.Arrays;
public class Main {
static int unvisitedLeaves( int N, int leaves, int [] frogs) {
boolean [] visited = new boolean [leaves + 1 ];
int unvisitedLeavesCount = leaves;
Arrays.sort(frogs);
for ( int i = 0 ; i < N; ++i) {
int strength_i = frogs[i];
if (strength_i == 1 )
return 0 ;
if (strength_i > leaves)
continue ;
if (visited[strength_i])
continue ;
for ( int j = strength_i; j <= leaves; j += strength_i) {
if (!visited[j])
unvisitedLeavesCount--;
visited[j] = true ;
}
}
return unvisitedLeavesCount;
}
public static void main(String[] args) {
int N = 3 ;
int leaves = 6 ;
int [] frogs = { 3 , 6 , 2 };
System.out.println(unvisitedLeaves(N, leaves, frogs));
}
}
|
Python3
def unvisited_leaves(N, leaves, frogs):
visited = [ False ] * (leaves + 1 )
unvisited_leaves_count = leaves
frogs.sort()
for i in range (N):
strength_i = frogs[i]
if strength_i = = 1 :
return 0
if strength_i > leaves:
continue
if visited[strength_i]:
continue
for j in range (strength_i, leaves + 1 , strength_i):
if not visited[j]:
unvisited_leaves_count - = 1
visited[j] = True
return unvisited_leaves_count
if __name__ = = "__main__" :
N = 3
leaves = 6
frogs = [ 3 , 6 , 2 ]
print (unvisited_leaves(N, leaves, frogs))
|
C#
using System;
class Program
{
static int UnvisitedLeaves( int N, int leaves, int [] frogs)
{
bool [] visited = new bool [leaves + 1];
int unvisitedLeavesCount = leaves;
Array.Sort(frogs);
for ( int i = 0; i < N; ++i)
{
int strength_i = frogs[i];
if (strength_i == 1)
return 0;
if (strength_i > leaves)
continue ;
if (visited[strength_i])
continue ;
for ( int j = strength_i; j <= leaves; j += strength_i)
{
if (!visited[j])
unvisitedLeavesCount--;
visited[j] = true ;
}
}
return unvisitedLeavesCount;
}
static void Main()
{
int N = 3;
int leaves = 6;
int [] frogs = { 3, 6, 2 };
Console.WriteLine(UnvisitedLeaves(N, leaves, frogs));
}
}
|
Javascript
function unvisitedLeaves(N, leaves, frogs) {
let visited = Array(leaves + 1).fill( false );
let unvisitedLeavesCount = leaves;
frogs.sort((a, b) => a - b);
for (let i = 0; i < N; ++i) {
let strength_i = frogs[i];
if (strength_i === 1) {
return 0;
}
if (strength_i > leaves) {
continue ;
}
if (visited[strength_i]) {
continue ;
}
for (let j = strength_i; j <= leaves; j += strength_i) {
if (!visited[j]) {
unvisitedLeavesCount--;
}
visited[j] = true ;
}
}
return unvisitedLeavesCount;
}
let N = 3;
let leaves = 6;
let frogs = [3, 6, 2];
console.log(unvisitedLeaves(N, leaves, frogs));
|
Time Complexity: O(N.log(N) + N*leaves*log(log(leaves))), N.log(N) for sorting strength of frogs. N*(leaves*log(log(leaves))) for iterating over the leaves. So the total time complexity would be a max of N.log(N), N*(leaves*log(log(leaves))).
Auxiliary Space: O(N)
Share your thoughts in the comments
Please Login to comment...