Count even paths in Binary Tree
Last Updated :
27 Mar, 2023
Given a Binary Tree, the task is to count the number of even paths in the given Binary Tree. Even Path is a path where root to leaf path contains all even nodes only.
Examples:
Input: Below is the given Binary Tree:
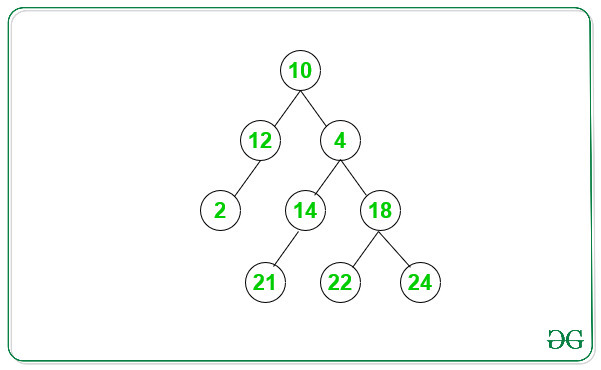
Output: 3
Explanation:
There are 3 even path for the above Binary Tree:
1. 10->12->2
2. 10->4->18->22
3. 10->4->18->24
Input: Below is the given Binary Tree:
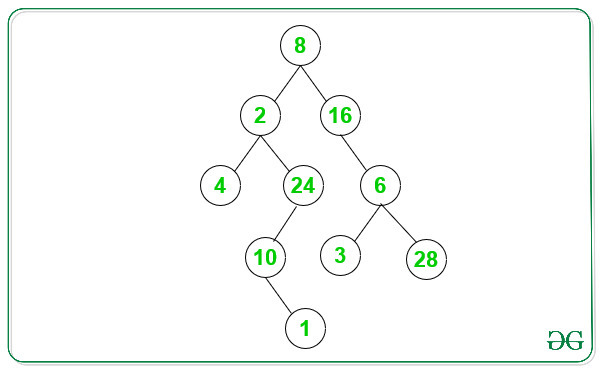
Output: 2
Explanation:
There are 2 even path for the above Binary Tree:
1. 8->2->4
2. 8->16->6->28
Naive Approach: The idea is to generate all the root to leaf path and check whether all nodes in every path is even or not. Count all the paths with even nodes in it and return the count. The above implementation takes extra space to store the path.
Efficient Approach: The idea is to use Preorder Tree Traversal. During preorder traversal of the given binary tree do the following:
- If the current value of the node is odd or the pointer becomes NULL then return the count.
- If the current node is a leaf node then increment the count by 1.
- Recursively call for the left and right subtree with the updated count.
- After the all-recursive calls, the value of count is the number of even paths for a given binary tree.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
struct Node {
int key;
struct Node *left, *right;
};
Node* newNode( int key)
{
Node* temp = new Node;
temp->key = key;
temp->left = temp->right = NULL;
return (temp);
}
int evenPaths( struct Node* node, int count)
{
if (node == NULL || (node->key % 2 != 0)) {
return count;
}
if (!node->left && !node->right) {
count++;
}
count = evenPaths(node->left, count);
return evenPaths(node->right, count);
}
int countEvenPaths( struct Node* node)
{
return evenPaths(node, 0);
}
int main()
{
Node* root = newNode(12);
root->left = newNode(13);
root->right = newNode(12);
root->right->left = newNode(14);
root->right->right = newNode(16);
root->right->left->left = newNode(21);
root->right->left->right = newNode(22);
root->right->right->left = newNode(22);
root->right->right->right = newNode(24);
root->right->right->right->left = newNode(8);
cout << countEvenPaths(root);
return 0;
}
|
Java
import java.util.*;
class GFG{
static class Node {
int key;
Node left, right;
};
static Node newNode( int key)
{
Node temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
static int evenPaths(Node node, int count)
{
if (node == null || (node.key % 2 != 0 )) {
return count;
}
if (node.left == null && node.right == null ) {
count++;
}
count = evenPaths(node.left, count);
return evenPaths(node.right, count);
}
static int countEvenPaths(Node node)
{
return evenPaths(node, 0 );
}
public static void main(String args[])
{
Node root = newNode( 12 );
root.left = newNode( 13 );
root.right = newNode( 12 );
root.right.left = newNode( 14 );
root.right.right = newNode( 16 );
root.right.left.left = newNode( 21 );
root.right.left.right = newNode( 22 );
root.right.right.left = newNode( 22 );
root.right.right.right = newNode( 24 );
root.right.right.right.left = newNode( 8 );
System.out.println(countEvenPaths(root));
}
}
|
Python3
class Node:
def __init__( self , x):
self .key = x
self .left = None
self .right = None
def evenPaths(node, count):
if (node = = None or
(node.key % 2 ! = 0 )):
return count
if ( not node.left and
not node.right):
count + = 1
count = evenPaths(node.left,
count)
return evenPaths(node.right,
count)
def countEvenPaths(node):
return evenPaths(node, 0 )
if __name__ = = '__main__' :
root = Node( 12 )
root.left = Node( 13 )
root.right = Node( 12 )
root.right.left = Node( 14 )
root.right.right = Node( 16 )
root.right.left.left = Node( 21 )
root.right.left.right = Node( 22 )
root.right.right.left = Node( 22 )
root.right.right.right = Node( 24 )
root.right.right.right.left = Node( 8 )
print (countEvenPaths(root))
|
C#
using System;
class GFG{
class Node {
public int key;
public Node left, right;
};
static Node newNode( int key)
{
Node temp = new Node();
temp.key = key;
temp.left = temp.right = null ;
return (temp);
}
static int evenPaths(Node node, int count)
{
if (node == null || (node.key % 2 != 0)) {
return count;
}
if (node.left == null && node.right == null ) {
count++;
}
count = evenPaths(node.left, count);
return evenPaths(node.right, count);
}
static int countEvenPaths(Node node)
{
return evenPaths(node, 0);
}
public static void Main(String []args)
{
Node root = newNode(12);
root.left = newNode(13);
root.right = newNode(12);
root.right.left = newNode(14);
root.right.right = newNode(16);
root.right.left.left = newNode(21);
root.right.left.right = newNode(22);
root.right.right.left = newNode(22);
root.right.right.right = newNode(24);
root.right.right.right.left = newNode(8);
Console.WriteLine(countEvenPaths(root));
}
}
|
Javascript
<script>
class Node
{
constructor(key)
{
this .key = key;
this .left = this .right = null ;
}
}
function evenPaths(node, count)
{
if (node == null || (node.key % 2 != 0))
{
return count;
}
if (node.left == null && node.right == null )
{
count++;
}
count = evenPaths(node.left, count);
return evenPaths(node.right, count);
}
function countEvenPaths(node)
{
return evenPaths(node, 0);
}
let root = new Node(12);
root.left = new Node(13);
root.right = new Node(12);
root.right.left = new Node(14);
root.right.right = new Node(16);
root.right.left.left = new Node(21);
root.right.left.right = new Node(22);
root.right.right.left = new Node(22);
root.right.right.right = new Node(24);
root.right.right.right.left = new Node(8);
document.write(countEvenPaths(root));
</script>
|
Time Complexity: O(N), where N is the number of nodes in the given binary tree.
Auxiliary Space: O(H), where H is the height of the binary tree. This is because the space used by the recursive call stack is proportional to the height of the tree. In the worst case scenario, when the tree is skewed and has a height of N (i.e., a linked list), the space complexity would be O(N).
Share your thoughts in the comments
Please Login to comment...