Concrete GenericAPIViews in Django Rest Framework.
Last Updated :
10 Apr, 2024
Django Rest Framework (DRF) provides powerful tools for building RESTful APIs in Django projects. One of the key components of DRF is Concrete GenericAPIViews, which offer pre-implemented views for common CRUD (Create, Read, Update, Delete) operations. In this article, we will see Concrete GenericAPIViews in Django Rest Framework.
What is Concrete GenericAPIViews in Django?
Concrete GenericAPIViews in Django are pre-defined view classes that simplify the creation of RESTful APIs by providing ready-to-use functionalities for common HTTP methods like GET, POST, PUT and DELETE. They include classes like ListAPIView
, CreateAPIView
, RetrieveAPIView
, UpdateAPIView
, and DestroyAPIView
, reducing the need for writing repetitive code.
Concrete GenericAPIViews in Django Rest Framework
Below, are the Implementation of Concrete GenericAPIViews in the Django Rest Framework in Python:
Starting the Project Folder
To start the project use this command
django-admin startproject bookstore
cd bookstore
To start the app use this command
django-admin startapp books
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"books",
"rest_framework",
]
For install the Django rest_framework use the below command
pip install djangorestframework
File Structure
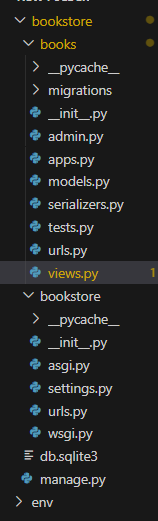
File Structure
Setting Necessary Files
books/models.py: Define the Book model as follows:
Python3
# books/models.py
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=100)
publication_year = models.IntegerField()
books/serializers.py : Create a file named serializers.py and define the Book serializer.
Python3
# books/serializers.py
from rest_framework import serializers
from .models import Book
class BookSerializer(serializers.ModelSerializer):
class Meta:
model = Book
fields = '__all__'
books/views.py : Create a file named views.py and define the views for handling CRUD operations:
Python3
# books/views.py
from rest_framework.generics import ListCreateAPIView, RetrieveUpdateDestroyAPIView
from .models import Book
from .serializers import BookSerializer
class BookListCreateAPIView(ListCreateAPIView):
queryset = Book.objects.all()
serializer_class = BookSerializer
class BookRetrieveUpdateDestroyAPIView(RetrieveUpdateDestroyAPIView):
queryset = Book.objects.all()
serializer_class = BookSerializer
books/urls.py : Define the URLs for the views using the following code:
Python3
# books/urls.py
from django.urls import path
from .views import BookListCreateAPIView, BookRetrieveUpdateDestroyAPIView
urlpatterns = [
path('books/', BookListCreateAPIView.as_view(), name='book-list-create'),
path('books/<int:pk>/', BookRetrieveUpdateDestroyAPIView.as_view(),
name='book-retrieve-update-destroy'),
]
bookstore/urls.py: Include the URLs of the books app using the following code.
Python3
# bookstore/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('/', include('books.urls')),
]
Deployment of the Project
Run these commands to apply the migrations:
python3 manage.py makemigrations
python3 manage.py migrate
Run the server with the help of following command:
python3 manage.py runserver
Output
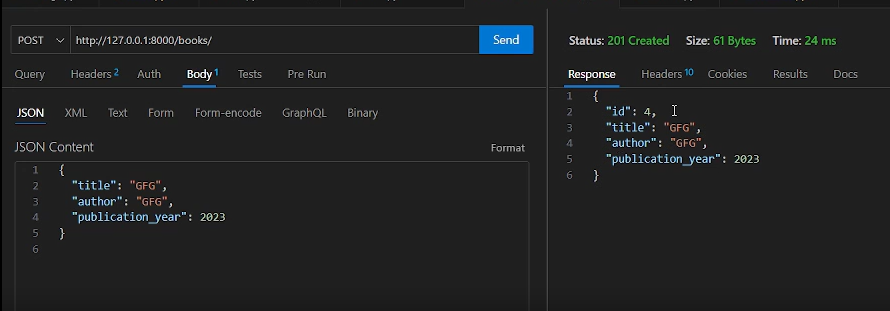
Post Concrete GenericAPIViews in Django
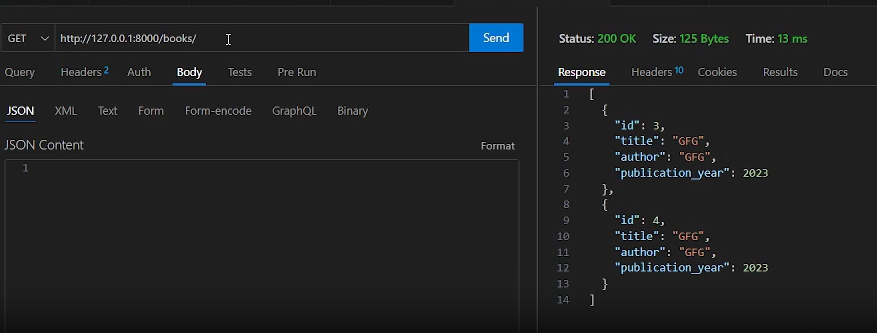
Get Concrete GenericAPIViews in Django
Video Demonstration
Share your thoughts in the comments
Please Login to comment...