ClosedChannelException in Java with Examples
Last Updated :
16 Feb, 2022
The class ClosedChannelException is invoked when an I/O operation is attempted on a closed channel or a channel that is closed to the attempted operation. That is if this exception is thrown, however, does not imply the channel is completely closed but is closed to the attempted operation.
Syntax:
public class ClosedChannelException
extends IOException
The hierarchy of ClosedChannelException is as follows:
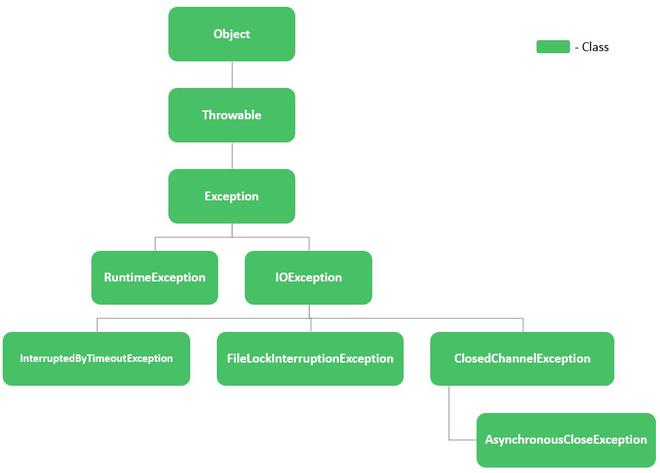
Now let us do see constructor details of this class before proceeding to it’s methods.
Constructor |
Description |
ClosedChannelException() |
Constructs an instance of the class |
Now let us discuss the methods inherited from Throwable class. They are depicted in tabular format below ias follows:
Method |
Description |
addSuppressed(Throwable exception) |
Add this exception to the exceptions that were suppressed so that this exception could be dispatched. |
fillInStackTrace() |
Records within this Throwable object information about the current state of the stack frame for the current thread and fills in the execution stack trace. |
getCause() |
Returns the cause of this Throwable or null if the cause is unknown. |
getLocalizedMessage() |
Returns a localized description of this Throwable. Subclasses may override the description. If the subclass doesn’t override this method then the result will be the same as that of getMessage(). |
getMessage() |
Return the detailed message description of this Throwable. |
getStackTrace() |
Returns an array of stack trace elements, each representing one stack frame. Gives access to the stack trace information printed by printStackTrace(). |
getSuppressed() |
Returns an array containing all the exceptions that were suppressed in order to dispatch this exception. |
initCause(Throwable cause) |
Initializes the cause of this Throwable with the given value. |
printStackTrace() |
Prints this Throwable and its backtrace on the error output stream. |
printStackTrace(PrintStream s) |
Prints this Throwable and its backtrace on the specified PrintStream. |
printStackTrace(PrintWriter s) |
Prints this Throwable and its backtrace to the specified PrintWriter. |
setStackTrace(StackTraceElement[] stackTrace) |
Sets the stack trace elements of this Throwable. It is designed for Remote Procedure Call Frameworks and advanced systems, it allows the client to override the default stack trace. |
toString() |
Returns a short description of this Throwable in the format, Name of the class of this object: Result of invoking the object’s getLocalizedMessage(). If getLocalizedMessage() returns null, then only the class name is returned. |
Note: this refers to the object in whose context the method is being called.
Implementation: We are essentially going to create a channel, closing it, and then trying to perform a read operation on a closed channel. This will trigger the ClosedChannelException. The steps are as follows:
- We will create an instance of RandomAccessFile class to open a text file from your system in “rw” i.e read-write mode.
- Now we create a channel to the opened file using FileChannel class.
- After that, we create a buffer to read bytes of data from this channel using ByteBuffer class.
- Further, Charset class, we define the encoding scheme as “US-ASCII”.
- Finally, before we start the process of reading this file, we close the channel.
Therefore, when a read operation is attempted on this channel a ClosedChannelException is thrown. We catch the Exception in the catch block where you may add any Exception handling that is specific to your requirement, here we are only printing a message.
Example
Java
import java.io.IOException;
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.ClosedChannelException;
import java.nio.channels.FileChannel;
import java.nio.charset.Charset;
public class GFG {
public static void main(String args[])
throws IOException
{
try {
RandomAccessFile randomAccessFile
= new RandomAccessFile(
"D:/Documents/textDoc.txt" , "rw" );
FileChannel fileChannel
= randomAccessFile.getChannel();
ByteBuffer byteBuffer
= ByteBuffer.allocate( 512 );
Charset charset = Charset.forName( "US-ASCII" );
fileChannel.close();
while (fileChannel.read(byteBuffer) > 0 ) {
byteBuffer.rewind();
System.out.print(
charset.decode(byteBuffer));
byteBuffer.flip();
}
randomAccessFile.close();
}
catch (ClosedChannelException e) {
System.out.println(
"ClosedChannelException has occurred" );
}
}
}
|
Output:

Share your thoughts in the comments
Please Login to comment...