Check for star graph
Last Updated :
21 Mar, 2023
You are given an n * n matrix which represents a graph with n-vertices, check whether the input matrix represents a star graph or not.
Example:
Input : Mat[][] = {{0, 1, 0},
{1, 0, 1},
{0, 1, 0}}
Output : Star graph
Input : Mat[][] = {{0, 1, 0},
{1, 1, 1},
{0, 1, 0}}
Output : Not a Star graph
Star graph: Star graph is a special type of graph in which n-1 vertices have degree 1 and a single vertex have degree n – 1. This looks like n – 1 vertex is connected to a single central vertex. A star graph with total n – vertex is termed as Sn.
Here is an illustration for the star graph :
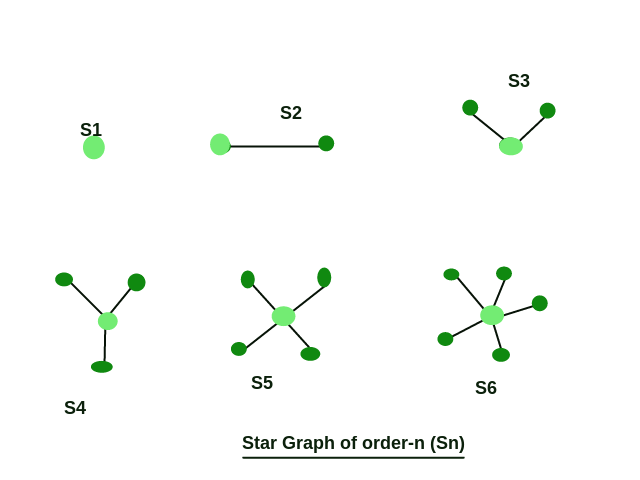
Approach: Just traverse whole matrix and record the number of vertices having degree 1 and degree n-1. If number of vertices having degree 1 is n-1 and number of vertex having degree n-1 is 1 then our graph should be a star graph other-wise it should be not.
Note:
- For S1, there must be only one vertex with no edges.
- For S2, there must be two vertices each with degree one or can say, both are connected by a single edge.
- For Sn (n>2) simply check the above-explained criteria.
Implementation:
C++
#include<bits/stdc++.h>
using namespace std;
#define size 4
bool checkStar( int mat[][size])
{
int vertexD1 = 0, vertexDn_1 = 0;
if (size == 1)
return (mat[0][0] == 0);
if (size == 2)
return (mat[0][0] == 0 && mat[0][1] == 1 &&
mat[1][0] == 1 && mat[1][1] == 0 );
for ( int i = 0; i < size; i++)
{
int degreeI = 0;
for ( int j = 0; j < size; j++)
if (mat[i][j])
degreeI++;
if (degreeI == 1)
vertexD1++;
else if (degreeI == size-1)
vertexDn_1++;
}
return (vertexD1 == (size-1) &&
vertexDn_1 == 1);
}
int main()
{
int mat[size][size] = { {0, 1, 1, 1},
{1, 0, 0, 0},
{1, 0, 0, 0},
{1, 0, 0, 0}};
checkStar(mat) ? cout << "Star Graph" :
cout << "Not a Star Graph" ;
return 0;
}
|
Java
import java.io.*;
class GFG
{
static int size = 4 ;
static boolean checkStar( int mat[][])
{
int vertexD1 = 0 ,
vertexDn_1 = 0 ;
if (size == 1 )
return (mat[ 0 ][ 0 ] == 0 );
if (size == 2 )
return (mat[ 0 ][ 0 ] == 0 &&
mat[ 0 ][ 1 ] == 1 &&
mat[ 1 ][ 0 ] == 1 &&
mat[ 1 ][ 1 ] == 0 );
for ( int i = 0 ; i < size; i++)
{
int degreeI = 0 ;
for ( int j = 0 ; j < size; j++)
if (mat[i][j] == 1 )
degreeI++;
if (degreeI == 1 )
vertexD1++;
else if (degreeI == size - 1 )
vertexDn_1++;
}
return (vertexD1 == (size - 1 ) &&
vertexDn_1 == 1 );
}
public static void main(String args[])
{
int mat[][] = {{ 0 , 1 , 1 , 1 },
{ 1 , 0 , 0 , 0 },
{ 1 , 0 , 0 , 0 },
{ 1 , 0 , 0 , 0 }};
if (checkStar(mat))
System.out.print( "Star Graph" );
else
System.out.print( "Not a Star Graph" );
}
}
|
Python3
size = 4
def checkStar(mat) :
global size
vertexD1 = 0
vertexDn_1 = 0
if (size = = 1 ) :
return (mat[ 0 ][ 0 ] = = 0 )
if (size = = 2 ) :
return (mat[ 0 ][ 0 ] = = 0 and
mat[ 0 ][ 1 ] = = 1 and
mat[ 1 ][ 0 ] = = 1 and
mat[ 1 ][ 1 ] = = 0 )
for i in range ( 0 , size) :
degreeI = 0
for j in range ( 0 , size) :
if (mat[i][j]) :
degreeI = degreeI + 1
if (degreeI = = 1 ) :
vertexD1 = vertexD1 + 1
elif (degreeI = = size - 1 ):
vertexDn_1 = vertexDn_1 + 1
return (vertexD1 = = (size - 1 ) and
vertexDn_1 = = 1 )
mat = [[ 0 , 1 , 1 , 1 ],
[ 1 , 0 , 0 , 0 ],
[ 1 , 0 , 0 , 0 ],
[ 1 , 0 , 0 , 0 ]]
if (checkStar(mat)) :
print ( "Star Graph" )
else :
print ( "Not a Star Graph" )
|
C#
using System;
class GFG
{
static int size = 4;
static bool checkStar( int [,]mat)
{
int vertexD1 = 0, vertexDn_1 = 0;
if (size == 1)
return (mat[0, 0] == 0);
if (size == 2)
return (mat[0, 0] == 0 &&
mat[0, 1] == 1 &&
mat[1, 0] == 1 &&
mat[1, 1] == 0);
for ( int i = 0; i < size; i++)
{
int degreeI = 0;
for ( int j = 0; j < size; j++)
if (mat[i, j] == 1)
degreeI++;
if (degreeI == 1)
vertexD1++;
else if (degreeI == size - 1)
vertexDn_1++;
}
return (vertexD1 == (size - 1) &&
vertexDn_1 == 1);
}
static void Main()
{
int [,]mat = new int [4, 4]{{0, 1, 1, 1},
{1, 0, 0, 0},
{1, 0, 0, 0},
{1, 0, 0, 0}};
if (checkStar(mat))
Console.Write( "Star Graph" );
else
Console.Write( "Not a Star Graph" );
}
}
|
PHP
<?php
$size = 4;
function checkStar( $mat )
{
global $size ;
$vertexD1 = 0;
$vertexDn_1 = 0;
if ( $size == 1)
return ( $mat [0][0] == 0);
if ( $size == 2)
return ( $mat [0][0] == 0 &&
$mat [0][1] == 1 &&
$mat [1][0] == 1 &&
$mat [1][1] == 0 );
for ( $i = 0; $i < $size ; $i ++)
{
$degreeI = 0;
for ( $j = 0; $j < $size ; $j ++)
if ( $mat [ $i ][ $j ])
$degreeI ++;
if ( $degreeI == 1)
$vertexD1 ++;
else if ( $degreeI == $size - 1)
$vertexDn_1 ++;
}
return ( $vertexD1 == ( $size - 1) &&
$vertexDn_1 == 1);
}
$mat = array ( array (0, 1, 1, 1),
array (1, 0, 0, 0),
array (1, 0, 0, 0),
array (1, 0, 0, 0));
if (checkStar( $mat ))
echo ( "Star Graph" );
else
echo ( "Not a Star Graph" );
?>
|
Javascript
<script>
var size = 4;
function checkStar( mat)
{
var vertexD1 = 0, vertexDn_1 = 0;
if (size == 1)
return (mat[0][0] == 0);
if (size == 2)
return (mat[0][0] == 0 && mat[0][1] == 1 &&
mat[1][0] == 1 && mat[1][1] == 0 );
for ( var i = 0; i < size; i++)
{
var degreeI = 0;
for ( var j = 0; j < size; j++)
if (mat[i][j])
degreeI++;
if (degreeI == 1)
vertexD1++;
else if (degreeI == size-1)
vertexDn_1++;
}
return (vertexD1 == (size-1) &&
vertexDn_1 == 1);
}
var mat = [ [0, 1, 1, 1],
[1, 0, 0, 0],
[1, 0, 0, 0],
[1, 0, 0, 0]];
checkStar(mat) ? document.write( "Star Graph" ) :
document.write( "Not a Star Graph" );
</script>
|
Approach 2: Breath First Search:
The BFS approach of the code first initializes an integer array ‘degree’ of size ‘size’ to store the degree of each vertex in the graph. It then traverses the graph using two nested loops and calculates the degree of each vertex by counting the number of edges that are incident on the vertex. The degree of each vertex is stored in the corresponding position of the ‘degree’ array.
Next, the code searches for a center vertex of the graph. A center vertex is a vertex that is connected to all other vertices in the graph. The code checks the degree of each vertex in the ‘degree’ array and looks for a vertex whose degree is equal to the size of the graph minus one. If such a vertex is found, it is stored in the ‘center’ variable.
C++
#include<bits/stdc++.h>
using namespace std;
#define size 4
bool checkStar( int mat[][size]) {
int degree[size] = {0};
for ( int i=0; i<size; i++) {
for ( int j=0; j<size; j++) {
if (mat[i][j]) {
degree[i]++;
}
}
}
int center = -1;
for ( int i=0; i<size; i++) {
if (degree[i] == size-1) {
center = i;
break ;
}
}
if (center == -1) {
return false ;
}
for ( int i=0; i<size; i++) {
if (i != center && degree[i] != 1) {
return false ;
}
}
return true ;
}
int main() {
int mat[size][size] = { {0, 1, 1, 1},
{1, 0, 0, 0},
{1, 0, 0, 0},
{1, 0, 0, 0}};
if (checkStar(mat)) {
cout << "Star Graph" ;
} else {
cout << "Not a Star Graph" ;
}
return 0;
}
|
Java
import java.util.*;
public class StarGraph {
static int size = 4 ;
static boolean checkStar( int [][] mat) {
int [] degree = new int [size];
for ( int i= 0 ; i<size; i++) {
for ( int j= 0 ; j<size; j++) {
if (mat[i][j] == 1 ) {
degree[i]++;
}
}
}
int center = - 1 ;
for ( int i= 0 ; i<size; i++) {
if (degree[i] == size- 1 ) {
center = i;
break ;
}
}
if (center == - 1 ) {
return false ;
}
for ( int i= 0 ; i<size; i++) {
if (i != center && degree[i] != 1 ) {
return false ;
}
}
return true ;
}
public static void main(String[] args) {
int [][] mat = {{ 0 , 1 , 1 , 1 },
{ 1 , 0 , 0 , 0 },
{ 1 , 0 , 0 , 0 },
{ 1 , 0 , 0 , 0 }};
if (checkStar(mat)) {
System.out.println( "Star Graph" );
} else {
System.out.println( "Not a Star Graph" );
}
}
}
|
Python3
size = 4
def checkStar(mat):
degree = [ 0 ] * size
for i in range (size):
for j in range (size):
if mat[i][j]:
degree[i] + = 1
center = - 1
for i in range (size):
if degree[i] = = size - 1 :
center = i
break
if center = = - 1 :
return False
for i in range (size):
if i ! = center and degree[i] ! = 1 :
return False
return True
mat = [[ 0 , 1 , 1 , 1 ],
[ 1 , 0 , 0 , 0 ],
[ 1 , 0 , 0 , 0 ],
[ 1 , 0 , 0 , 0 ]]
if checkStar(mat):
print ( "Star Graph" )
else :
print ( "Not a Star Graph" )
|
C#
using System;
public class Program {
const int size = 4;
static bool CheckStar( int [,] mat) {
int [] degree = new int [size];
for ( int i=0; i<size; i++) {
for ( int j=0; j<size; j++) {
if (mat[i,j] != 0) {
degree[i]++;
}
}
}
int center = -1;
for ( int i=0; i<size; i++) {
if (degree[i] == size-1) {
center = i;
break ;
}
}
if (center == -1) {
return false ;
}
for ( int i=0; i<size; i++) {
if (i != center && degree[i] != 1) {
return false ;
}
}
return true ;
}
public static void Main() {
int [,] mat = {{0, 1, 1, 1},
{1, 0, 0, 0},
{1, 0, 0, 0},
{1, 0, 0, 0}};
if (CheckStar(mat)) {
Console.WriteLine( "Star Graph" );
} else {
Console.WriteLine( "Not a Star Graph" );
}
}
}
|
Javascript
const size = 4;
function checkStar(mat) {
const degree = new Array(size).fill(0);
for (let i = 0; i < size; i++) {
for (let j = 0; j < size; j++) {
if (mat[i][j] !== 0) {
degree[i]++;
}
}
}
let center = -1;
for (let i = 0; i < size; i++) {
if (degree[i] === size - 1) {
center = i;
break ;
}
}
if (center === -1) {
return false ;
}
for (let i = 0; i < size; i++) {
if (i !== center && degree[i] !== 1) {
return false ;
}
}
return true ;
}
const mat = [
[0, 1, 1, 1],
[1, 0, 0, 0],
[1, 0, 0, 0],
[1, 0, 0, 0],
];
if (checkStar(mat)) {
console.log( "Star Graph" );
} else {
console.log( "Not a Star Graph" );
}
|
Time Complexity: O(size^2), where size is the size of the adjacency matrix.
Auxiliary Space: O(size), where size is the size of the adjacency matrix.
Share your thoughts in the comments
Please Login to comment...