Check if the robot is within the bounds of the grid after given moves
Last Updated :
07 Jun, 2022
Given a grid of size N X M and a robot is placed at cell (N – 1, M – 1). Also, given string str which consists only of the characters ‘U’ (Up), ‘D’ (Down), ‘L’ (Left) and ‘R’ (Right) representing the moves the robot is going to perform within the grid. The task is to find whether the robot will be safe at the end of the last move. Robot is said to be safe if it is within the bounds of the grid.
Note: Consider that the rectangular grid is present below the number line with the top-left corner lying on the origin.
Examples:
Input: N = 1, M = 1, str = “R”
Output: No
As there is only 1 cell, no movement is allowed.
Input: N = 2, M = 3, str = “LLRU”
Output: Yes
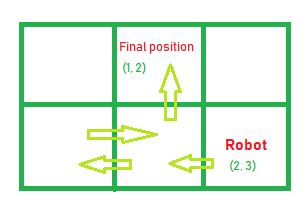
Approach: For every move, update the position of the robot inside the grid. if at any move the position of the robot is outside the grid then the output will be No else print Yes if for all the moves, the robot is within the bounds of the grid.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
bool isSafe( int N, int M, string str)
{
int coll = 0, colr = 0, rowu = 0, rowd = 0;
for ( int i = 0; i < str.length(); i++) {
if (str[i] == 'L' ) {
coll++;
if (colr > 0) {
colr--;
}
if (coll == M) {
break ;
}
}
else if (str[i] == 'R' ) {
colr++;
if (coll > 0) {
coll--;
}
if (colr == M) {
break ;
}
}
else if (str[i] == 'U' ) {
-rowu++;
if (rowd > 0) {
rowd--;
}
if (rowu == N) {
break ;
}
}
else if (str[i] == 'D' ) {
rowd++;
if (rowu > 0) {
rowu--;
}
if (rowd == N) {
break ;
}
}
}
if ( abs (rowd) < N && abs (rowu) < N
&& abs (coll) < M && abs (colr) < M) {
return true ;
}
return false ;
}
int main()
{
int N = 1, M = 1;
string str = "R" ;
if (isSafe(N, M, str))
cout << "Yes" ;
else
cout << "No" ;
return 0;
}
|
Java
class GFG {
static boolean isSafe( int N, int M, char [] str)
{
int coll = 0 , colr = 0 , rowu = 0 , rowd = 0 ;
for ( int i = 0 ; i < str.length; i++) {
if (str[i] == 'L' ) {
coll++;
if (colr > 0 ) {
colr--;
}
if (coll == M) {
break ;
}
}
else if (str[i] == 'R' ) {
colr++;
if (coll > 0 ) {
coll--;
}
if (colr == M) {
break ;
}
}
else if (str[i] == 'U' ) {
rowu++;
if (rowd > 0 ) {
rowd--;
}
if (rowu == N) {
break ;
}
}
else if (str[i] == 'D' ) {
rowd++;
if (rowu > 0 ) {
rowu--;
}
if (rowd == N) {
break ;
}
}
}
if (Math.abs(rowd) < N && Math.abs(rowu) < N
&& Math.abs(coll) < M && Math.abs(colr) < M) {
return true ;
}
return false ;
}
public static void main(String[] args)
{
int N = 1 , M = 1 ;
String str = "R" ;
if (isSafe(N, M, str.toCharArray()))
System.out.println( "Yes" );
else
System.out.println( "No" );
}
}
|
Python3
def isSafe(N, M, str ):
coll = 0
colr = 0
rowu = 0
rowd = 0
for i in range ( len ( str )):
if ( str [i] = = 'L' ):
coll + = 1
if (colr > 0 ):
colr - = 1
if (coll = = M):
break
elif ( str [i] = = 'R' ):
colr + = 1
if (coll > 0 ):
coll - = 1
if (colr = = M):
break
elif ( str [i] = = 'U' ):
rowu + = 1
if (rowd > 0 ):
rowd - = 1
if (rowu = = N):
break
elif ( str [i] = = 'D' ):
rowd + = 1
if (rowu > 0 ):
rowu - = 1
if (rowd = = N):
break
if ( abs (rowd) < N and abs (rowu) < N and
abs (coll) < M and abs (colr) < M):
return True
return False
if __name__ = = '__main__' :
N = 1
M = 1
str = "R"
if (isSafe(N, M, str )):
print ( "Yes" )
else :
print ( "No" )
|
C#
using System;
class GFG {
static bool isSafe( int N, int M, char [] str)
{
int coll = 0, colr = 0, rowu = 0, rowd = 0;
for ( int i = 0; i < str.Length; i++) {
if (str[i] == 'L' ) {
coll++;
if (colr > 0) {
colr--;
}
if (coll == M) {
break ;
}
}
else if (str[i] == 'R' ) {
colr++;
if (coll > 0) {
coll--;
}
if (colr == M) {
break ;
}
}
else if (str[i] == 'U' ) {
rowu++;
if (rowd > 0) {
rowd--;
}
if (rowu == N) {
break ;
}
}
else if (str[i] == 'D' ) {
rowd++;
if (rowu > 0) {
rowu--;
}
if (rowd == N) {
break ;
}
}
}
if (Math.Abs(rowd) < N && Math.Abs(rowu) < N
&& Math.Abs(coll) < M && Math.Abs(colr) < M) {
return true ;
}
return false ;
}
public static void Main(String[] args)
{
int N = 1, M = 1;
String str = "R" ;
if (isSafe(N, M, str.ToCharArray()))
Console.WriteLine( "Yes" );
else
Console.WriteLine( "No" );
}
}
|
Javascript
<script>
function isSafe(N, M, str)
{
var coll = 0, colr = 0, rowu = 0, rowd = 0;
for ( var i = 0; i < str.length; i++) {
if (str[i] == 'L' ) {
coll++;
if (colr > 0) {
colr--;
}
if (coll == M) {
break ;
}
}
else if (str[i] == 'R' ) {
colr++;
if (coll > 0) {
coll--;
}
if (colr == M) {
break ;
}
}
else if (str[i] == 'U' ) {
-rowu++;
if (rowd > 0) {
rowd--;
}
if (rowu == N) {
break ;
}
}
else if (str[i] == 'D' ) {
rowd++;
if (rowu > 0) {
rowu--;
}
if (rowd == N) {
break ;
}
}
}
if (Math.abs(rowd) < N && Math.abs(rowu) < N
&& Math.abs(coll) < M && Math.abs(colr) < M) {
return true ;
}
return false ;
}
var N = 1, M = 1;
var str = "R" ;
if (isSafe(N, M, str))
document.write( "Yes" );
else
document.write( "No" );
</script>
|
Time Complexity: O(|str|)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...