Bootstrap 5 Forms Layout
Last Updated :
19 Feb, 2024
Bootstrap is one of the most popular frameworks for web developers. Developers utilize it to improve the front-end’s responsiveness. If we follow the bootstrap documentation, we will see numerous possibilities for incorporating layout into a website.
Bootstrap Layout
Bootstrap 5 layout contains various subtopics, which we have discussed one by one here with the help of examples.
In Bootstrap, form elements should be enclosed within a <form>
tag. While Bootstrap doesn’t style the <form>
element by default, it utilizes browser features effectively. Buttons inside a form default to type="submit"
, so it’s important to specify the type. You can disable all form elements within a form using the disabled
attribute on the <form>
. By default, Bootstrap forms stack vertically due to applied styles, but you can customize this layout using additional classes.
Example
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Forms</ title >
< link href =
rel = "stylesheet" >
</ head >
< body >
< div class = "container" >
< h2 >Bootstrap5 Form</ h2 >
< form >
< div class = "mb-3" >
< label for = "exampleInputEmail1" class = "form-label" >
Email address
</ label >
< input type = "email"
class = "form-control"
id = "exampleInputEmail1"
aria-describedby = "emailHelp" >
< div id = "emailHelp" class = "form-text" >
We'll never share your email with anyone else.
</ div >
</ div >
< div class = "mb-3" >
< label for = "exampleInputPassword1"
class = "form-label" >
Password
</ label >
< input type = "password"
class = "form-control"
id = "exampleInputPassword1" >
</ div >
< div class = "mb-3 form-check" >
< input type = "checkbox"
class = "form-check-input"
id = "exampleCheck1" >
< label class = "form-check-label"
for = "exampleCheck1" >
Check me out
</ label >
</ div >
< button type = "submit"
class = "btn btn-primary" >
Submit
</ button >
</ form >
</ div >
< script src =
</ script >
</ body >
</ html >
|
Output:
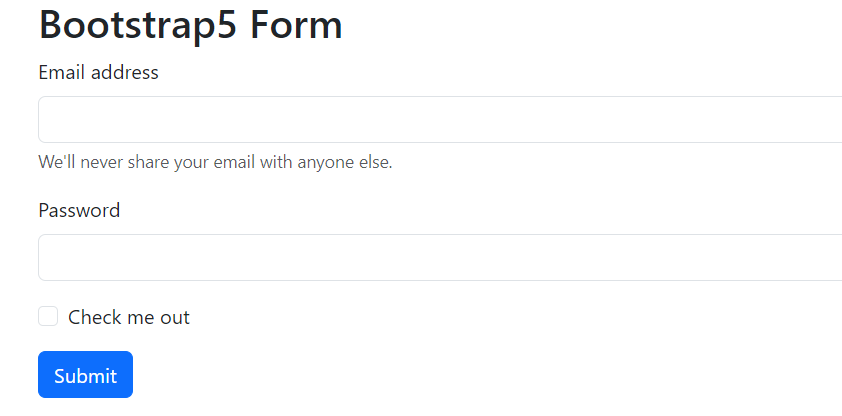
Utilities:
The bootstrap layout includes utility classes, which helps in the creation of outstanding responsive websites. One of the most significant and commonly used classes is “d-flex”, which permits the display property as a flex container and may be customised as needed; below is an example. Here, we can see that after applying the “d-flex” class, we used the “justify-content-center” class to centre the position of the divs, as well as adding margin and padding.
Example: Illustration of Boostrap utilities.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Utility</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >
GeeksforGeeks
</ h2 >
< div class = "container d-flex justify-content-center" >
< div class = "border border-2 border-success m-4 p-4" >
Item 1
</ div >
< div class = "border border-2 border-success m-4 p-4" >
Item 2
</ div >
< div class = "border border-2 border-success m-4 p-4" >
Item 3
</ div >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:
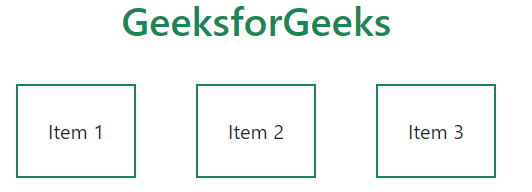
Output
The form grid is simply a normal grid with the same features as we saw earlier. In a typical bootstrap grid system, we employ the “row” and “col” classes, and the same is true for the form layout.
Example: Illustration of form grid in bootstrap.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Form Grid</ title >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< div class = "row" >
< div class = "col" >
< input type = "text"
class = "form-control"
placeholder = "First name"
aria-label = "First name" >
</ div >
< div class = "col" >
< input type = "text"
class = "form-control"
placeholder = "Last name"
aria-label = "Last name" >
</ div >
</ div >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:

Form Grid
Gutters:
In bootstrap gutter we use the “g” classes for adding margin or padding between elements. So we will use the same for the form gutters also. As we can see, there are no such changes, only a change in code, as we utilised the “g-3” class.
Example: Implementation to create form gutter.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Form Gutters</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< div class = "row g-3" >
< div class = "col" >
< input type = "text" class = "form-control"
placeholder = "First name" aria-label = "First name" >
</ div >
< div class = "col" >
< input type = "text" class = "form-control"
placeholder = "Last name" aria-label = "Last name" >
</ div >
</ div >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:

Form Gutters
To create horizontal forms using the grid, add the.row class to form groups and use the.col-*-* classes to set the width of your labels and controls. Add.col-form-label to your <label>s to centre them vertically with the corresponding form controls. As we can see, this is a rather sophisticated form, therefore we’re using a grid system with radio buttons, a checkbox button, and a typical submit button. And they are all horizontally aligned, which is why we call them horizontal forms.
Example: Implementation to create horizontal form .
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Horizontal Form</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< form >
< div class = "row mb-3" >
< label for = "inputEmail3"
class = "col-sm-2 col-form-label" >
Email
</ label >
< div class = "col-sm-10" >
< input type = "email"
class = "form-control"
id = "inputEmail3" >
</ div >
</ div >
< div class = "row mb-3" >
< label for = "inputPassword3"
class = "col-sm-2 col-form-label" >
Password
</ label >
< div class = "col-sm-10" >
< input type = "password"
class = "form-control"
id = "inputPassword3" >
</ div >
</ div >
< fieldset class = "row mb-3" >
< legend class = "col-form-label col-sm-2 pt-0" >Radios</ legend >
< div class = "col-sm-10" >
< div class = "form-check" >
< input class = "form-check-input"
type = "radio"
name = "gridRadios"
id = "gridRadios1"
value = "option1"
checked>
< label class = "form-check-label" for = "gridRadios1" >
First radio
</ label >
</ div >
< div class = "form-check" >
< input class = "form-check-input"
type = "radio"
name = "gridRadios"
id = "gridRadios2"
value = "option2" >
< label class = "form-check-label"
for = "gridRadios2" >
Second radio
</ label >
</ div >
< div class = "form-check disabled" >
< input class = "form-check-input"
type = "radio"
name = "gridRadios"
id = "gridRadios3"
value = "option3"
disabled>
< label class = "form-check-label" for = "gridRadios3" >
Third disabled radio
</ label >
</ div >
</ div >
</ fieldset >
< div class = "row mb-3" >
< div class = "col-sm-10 offset-sm-2" >
< div class = "form-check" >
< input class = "form-check-input"
type = "checkbox" id = "gridCheck1" >
< label class = "form-check-label" for = "gridCheck1" >
Example checkbox
</ label >
</ div >
</ div >
</ div >
< button type = "submit" class = "btn btn-primary" >Sign in</ button >
</ form >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:
.png)
Horizontal Form
This component allows us to resize the form columns as well as the labels, making it an extremely important class for making a website responsive. To align with the size of.form-control-lg and.form-control-sm, use.col-form-label-sm or.col-form-label-lg for your <label>s or <legend>. The code indicates that “col-form-label-sm” or “col-form-label-lg” are appropriate sizes for the project.
Example: Implementation to create horizontal form with label sizing.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Horizontal Form label sizing</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< div class = "row mb-3" >
< label for = "colFormLabelSm"
class = "col-sm-2 col-form-label col-form-label-sm" >
Email
</ label >
< div class = "col-sm-10" >
< input type = "email"
class = "form-control form-control-sm"
id = "colFormLabelSm"
placeholder = "col-form-label-sm" >
</ div >
</ div >
< div class = "row mb-3" >
< label for = "colFormLabel" class = "col-sm-2 col-form-label" >
Email
</ label >
< div class = "col-sm-10" >
< input type = "email"
class = "form-control"
id = "colFormLabel"
placeholder = "col-form-label" >
</ div >
</ div >
< div class = "row" >
< label for = "colFormLabelLg"
class = "col-sm-2 col-form-label col-form-label-lg" >
Email
</ label >
< div class = "col-sm-10" >
< input type = "email"
class = "form-control form-control-lg" id = "colFormLabelLg"
placeholder = "col-form-label-lg" >
</ div >
</ div >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:
.png)
Horizontal form label sizing
Column sizing:
As demonstrated in the preceding instances, our grid system allows you to include any number of.cols within a “row”. They will divide the available breadth equally between themselves. You can also select a subset of your columns to take up more or less space, while the remaining “cols” divide the rest equally, using column classes like “col-sm-7”. Here we set different sizes for the columns with the col and row classes.
Example: Implementation to create column sizing.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Column Sizing</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< div class = "row g-3" >
< div class = "col-sm-7" >
< input type = "text" class = "form-control"
placeholder = "City" aria-label = "City" >
</ div >
< div class = "col-sm" >
< input type = "text" class = "form-control"
placeholder = "State" aria-label = "State" >
</ div >
< div class = "col-sm" >
< input type = "text" class = "form-control"
placeholder = "Zip" aria-label = "Zip" >
</ div >
</ div >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:
.png)
Column Sizing
Auto Sizing:
It is a significant attribute since it uses a flexbox utility to vertically centre the items and converts “col” to “col-auto” so that your columns only take up the space they require. In other words, the column size is determined by its contents. Here we can clearly see how auto scaling works because the form we generated resizes itself as the screen size changes.
Example: Implementation to showcase auto sizing.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Auto Sizing</ title >
< meta charset = "UTF-8" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< form class = "row gy-2 gx-3 align-items-center" >
< div class = "col-auto" >
< label class = "visually-hidden"
for = "autoSizingInput" >
Name
</ label >
< input type = "text"
class = "form-control"
id = "autoSizingInput"
placeholder = "Jane Doe" >
</ div >
< div class = "col-auto" >
< label class = "visually-hidden"
for = "autoSizingInputGroup" >
Username
</ label >
< div class = "input-group" >
< div class = "input-group-text" >@</ div >
< input type = "text"
class = "form-control"
id = "autoSizingInputGroup"
placeholder = "Username" >
</ div >
</ div >
< div class = "col-auto" >
< label class = "visually-hidden"
for = "autoSizingSelect" >
Preference
</ label >
< select class = "form-select" id = "autoSizingSelect" >
< option selected>Choose...</ option >
< option value = "1" >One</ option >
< option value = "2" >Two</ option >
< option value = "3" >Three</ option >
</ select >
</ div >
< div class = "col-auto" >
< div class = "form-check" >
< input class = "form-check-input" type = "checkbox"
id = "autoSizingCheck" >
< label class = "form-check-label" for = "autoSizingCheck" >
Remember me
</ label >
</ div >
</ div >
< div class = "col-auto" >
< button type = "submit" class = "btn btn-primary" >Submit</ button >
</ div >
</ form >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:
.png)
Auto Sizing
Use the “row-cols-*” classes to construct responsive horizontal layouts. By adding gutter modifier classes, we will have gutters in both horizontal and vertical directions. On small mobile viewports, the “col-12” helps stack form controls and other elements. The “align-items-center” property moves the form elements to the centre, ensuring that the “form-check” is properly aligned.
Example: Illustration of Inline Form in Bootstrap.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Form Layouts</ title >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link href =
rel = "stylesheet"
integrity =
"sha384-rbsA2VBKQhggwzxH7pPCaAqO46MgnOM80zW1RWuH61DGLwZJEdK2Kadq2F9CUG65"
crossorigin = "anonymous" >
</ head >
< body >
< h2 class = "text-center text-success" >GeeksforGeeks</ h2 >
< div class = "container" >
< form class = "row row-cols-lg-auto g-3 align-items-center" >
< div class = "col-12" >
< label class = "visually-hidden"
for = "inlineFormInputGroupUsername" >
Username
</ label >
< div class = "input-group" >
< div class = "input-group-text" >@</ div >
< input type = "text" class = "form-control"
id = "inlineFormInputGroupUsername"
placeholder = "Username" >
</ div >
</ div >
< div class = "col-12" >
< label class = "visually-hidden"
for = "inlineFormSelectPref" >
Preference
</ label >
< select class = "form-select"
id = "inlineFormSelectPref" >
< option selected>Choose...</ option >
< option value = "1" >One</ option >
< option value = "2" >Two</ option >
< option value = "3" >Three</ option >
</ select >
</ div >
< div class = "col-12" >
< div class = "form-check" >
< input class = "form-check-input"
type = "checkbox"
id = "inlineFormCheck" >
< label class = "form-check-label"
for = "inlineFormCheck" >
Remember me
</ label >
</ div >
</ div >
< div class = "col-12" >
< button type = "submit" class = "btn btn-primary" >Submit</ button >
</ div >
</ form >
</ div >
< script src =
integrity =
"sha384-kenU1KFdBIe4zVF0s0G1M5b4hcpxyD9F7jL+jjXkk+Q2h455rYXK/7HAuoJl+0I4"
crossorigin = "anonymous" >
</ script >
</ body >
</ html >
|
Output:
.png)
Inline Form
Share your thoughts in the comments
Please Login to comment...