Applications of Recursion in JavaScript
Last Updated :
20 Sep, 2023
Recursion is a programming technique in which a function calls itself directly or indirectly. Using a recursive algorithm, certain problems can be solved quite easily. Examples of such problems are Towers of Hanoi (TOH), Inorder/Preorder/Postorder Tree Traversals, DFS of Graph, etc. Recursion is a technique in which we reduce the length of code and make it easy to read and write. A recursive function solves a problem by calling its own function and also calling for the smaller subproblem.
Recursion is a powerful technique that has many applications in the field of programming. Below are a few common applications of recursion that we frequently use:
- Tree and graph traversal
- Sorting algorithms
- Divide-and-conquer algorithms
- Sieve of Eratosthenes
- Fibonacci Numbers
Let’s deep dive into each application:
Tree traversal
The traversal of trees is very interesting, we can traverse trees in different ways. Recursion is also a very common way to traverse and manipulate tree structures.
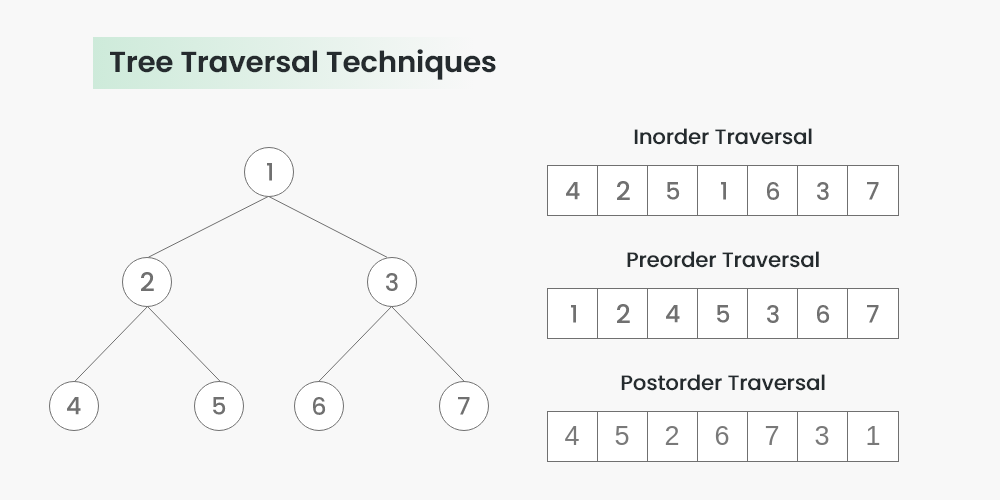
Example: In this example, we will show all three traversal(inorder, postorder and preorder) using recursion.
Javascript
class Node {
constructor(val) {
this .key = val;
this .left = null ;
this .right = null ;
}
}
let root = null ;
function printInorder(node) {
if (node == null )
return ;
printInorder(node.left);
console.log(node.key);
printInorder(node.right);
}
function printPreorder(node) {
if (node == null )
return ;
console.log(node.key);
printPreorder(node.left);
printPreorder(node.right);
}
function printPostorder(node) {
if (node == null )
return ;
printPostorder(node.left);
printPostorder(node.right);
console.log(node.key);
}
root = new Node(1);
root.left = new Node(2);
root.right = new Node(3);
root.left.left = new Node(4);
root.left.right = new Node(5);
console.log( "Inorder traversal of binary tree is " );
printInorder(root);
console.log( "Preorder traversal of binary tree is " );
printPreorder(root);
console.log( "Postorder traversal of binary tree is " );
printPostorder(root);
|
Output
Inorder traversal of binary tree is
4
2
5
1
3
Preorder traversal of binary tree is
1
2
4
5
3
Postorder traversal of binary tree is
4
5
2
3
1
Sorting algorithm
A Sorting Algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. The comparison operator is used to decide the new order of elements in the respective data structure. There are various types of sorting. We are going to see insertion sort using recursion.Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
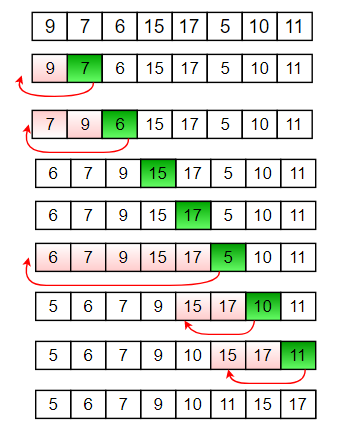
Example: In this example, we will see the insertion sort using recursion.
Javascript
function insertionSortRecursive(arr, n) {
if (n <= 1)
return ;
insertionSortRecursive(arr, n - 1);
let last = arr[n - 1];
let j = n - 2;
while (j >= 0 && arr[j] > last) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = last;
}
let arr = [8,6,1,3,5,9];
insertionSortRecursive(arr, arr.length);
for (let i = 0; i < arr.length; i++) {
console.log(arr[i] + " " );
}
|
Divide-and-conquer algorithms
Divide and Conquer is an algorithmic paradigm in which the problem is solved using the Divide, Conquer, and Combine strategy.
A typical Divide and Conquer algorithm solves a problem using following three steps:
- Divide: This involves dividing the problem into smaller sub-problems.
- Conquer: Solve sub-problems by calling recursively until solved.
- Combine: Combine the sub-problems to get the final solution of the whole problem.
A classic example of Divide and Conquer is Merge Sort demonstrated below. In Merge Sort, we divide array into two halves, sort the two halves recursively, and then merge the sorted halves.
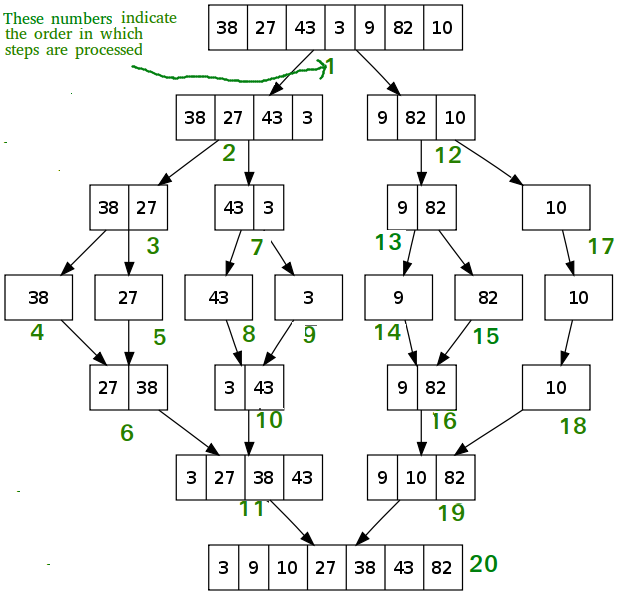
Example: In this example, we will show the merge sorting using recursion:
Javascript
function merge(arr, l, m, r) {
let n1 = m - l + 1;
let n2 = r - m;
let L = new Array(n1);
let R = new Array(n2);
for (let i = 0; i < n1; i++)
L[i] = arr[l + i];
for (let j = 0; j < n2; j++)
R[j] = arr[m + 1 + j];
let i = 0;
let j = 0;
let k = l;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
}
else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
function mergeSort(arr, l, r) {
if (l >= r) {
return ;
}
let m = l + parseInt((r - l) / 2);
mergeSort(arr, l, m);
mergeSort(arr, m + 1, r);
merge(arr, l, m, r);
}
function printArray(A, size) {
for (let i = 0; i < size; i++)
console.log(A[i] + " " );
}
let arr = [3,9,6,4,5,7];
let arr_size = arr.length;
mergeSort(arr, 0, arr_size - 1);
console.log( "Sorted array is " );
printArray(arr, arr_size);
|
Output
Sorted array is
3
4
5
6
7
9
Sieve of Eratosthenes
This algorithm is most optimised solution for finding the prime number.
Example: In this example, we will show the Sieve of Eratosthenes.
Javascript
function sieveOfEratosthenes(num) {
const primeNum = new Array(num + 1).fill( true );
primeNum[0] = primeNum[1] = false ;
for (let i = 2; i * i <= num; i++) {
if (primeNum[i]) {
for (let j = i * i; j <= num; j += i) {
primeNum[j] = false ;
}
}
}
return primeNum;
}
function isPrime(num) {
if (num < 2) {
return false ;
}
const primes = sieveOfEratosthenes(num);
return primes[num];
}
console.log(isPrime(97));
console.log(isPrime(66));
|
Fibonacci Numbers
In mathematical terms, the sequence Fn of Fibonacci numbers is defined by the recurrence relation: F_{n} = F_{n-1} + F_{n-2} with seed values and F_0 = 0 and F_1 = 1 .
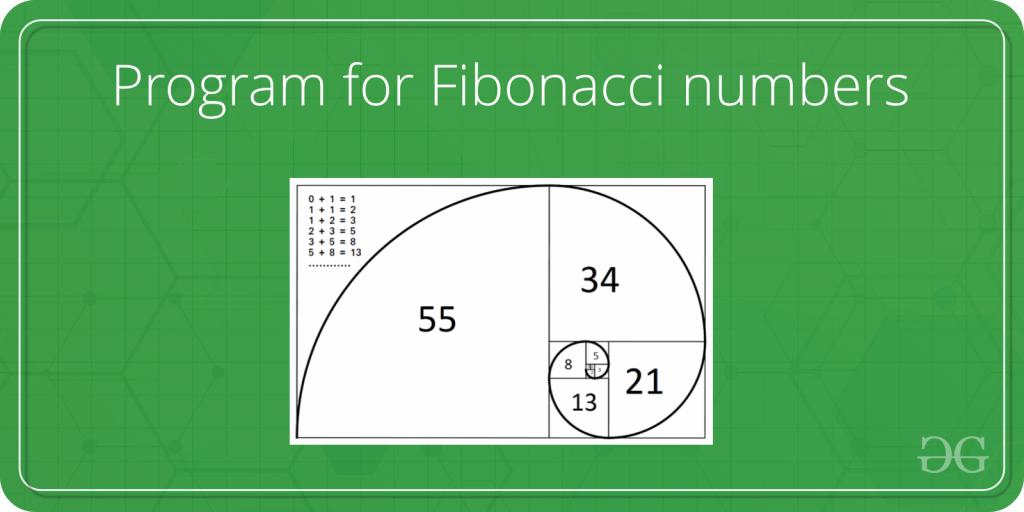
The Nth Fibonacci Number can be found using the recurrence relation shown above:
- if n = 0, then return 0.
- If n = 1, then it should return 1.
- For n > 1, it should return Fn-1 + Fn-2
Example: In this example, we will find the nth Fibonacci Number using Recursion.
Javascript
function Fib(n) {
if (n <= 1) {
return n;
} else {
return Fib(n - 1) + Fib(n - 2);
}
}
let n = 6;
console.log(n + "th Fibonacci Number: " + Fib(n));
|
Output
6th Fibonacci Number: 8
Share your thoughts in the comments
Please Login to comment...