Animated Slideshow App in HTML CSS & JavaScript
Last Updated :
28 Feb, 2024
We will learn to create a slideshow of multiple images. This slideshow will transition between the images every few seconds. we will further learn to align the images, style the images, and make the animation look better.
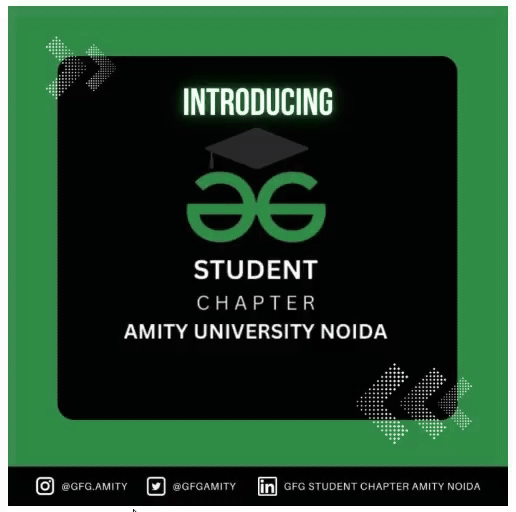
Prerequisites
Approach
- Create 3 files one for HTML, one for CSS, and one for JS in a single folder.
- In HTML file, Add the below HTML code. In this code, we have two main tags one is Heading and the other is a Division with 6 images. Adding the CSS Stylesheet link and the script to the JS file which is created in the same folder. Also, add slide, and fade classes to every image div.
- In CSS, we define the classes mentioned in the HTML class attributes. To create animation we have used a class called fade which creates a fading effect. Inside the fade class, we have an animation property with a duration of 2 seconds and triggered infinity. Using @keyframes we toggle the opacity of the slide between 0.4 to 1.0 and create fade effect.
- In JS file, we have nextSlide( ) function that is invoked every 2 seconds using setInterval( ) and is used to call other function showSlide( ) in the same file. showSlide( ) iterates through every slide and toggles the display of the slide which matches with the passed index. The index is generated by taking the modulus between currentSlide (Starts with zero) number and 6 as there are 6 slides and this generates numbers in sequential order starting from 1.
Example: This example shows the implementation of the above-explained approach.
Javascript
let currentSlide = 0;
function showSlide(index) {
const slides = document.querySelectorAll( '.slide' );
slides.forEach((slide, i) => {
slide.style.display = i === index ? 'flex' : 'none' ;
});
}
function nextSlide() {
currentSlide = (currentSlide + 1) % 6;
showSlide(currentSlide);
}
setInterval(nextSlide, 2000);
|
HTML
<!DOCTYPE html>
< html >
< head >
< title >GFG</ title >
< link rel = "stylesheet" href = "style.css" />
</ head >
< body >
< h1 >Slide Show</ h1 >
< div class = "slideshow-container" >
< div class = "slide fade" >
< img src =
alt = "Slide 1" >
</ div >
< div class = "slide fade" >
< img src =
</ div >
< div class = "slide fade" >
< img src =
alt = "Slide 3" >
</ div >
< div class = "slide fade" >
< img src =
alt = "Slide 3" >
</ div >
< div class = "slide fade" >
< img src =
</ div >
< div class = "slide fade" >
< img src =
</ div >
</ div >
< script src = "./script.js" ></ script >
</ body >
</ html >
|
CSS
body {
padding-bottom : 20px ;
font-family : Arial , sans-serif ;
display : flex;
flex- direction : column;
justify- content : center ;
align-items: center ;
height : 100 vh;
box-sizing: border-box;
overflow : hidden ;
}
h 1 {
font-size : 4 vw;
}
.slideshow-container {
width : 60% ;
aspect-ratio: 1 ;
position : relative ;
margin : auto ;
overflow : hidden ;
border-radius: 10px ;
box-shadow: 2px 2px 5px
}
.slide {
display : none ;
width : 100% ;
height : 100% ;
overflow : hidden ;
justify- content : center ;
align-items: center ;
}
img {
width : 100% ;
height : 100% ;
object-fit: contains;
}
.fade {
animation: fade 2 s ease-in-out infinite;
}
@keyframes fade {
from {
opacity: 0.4 ;
}
to {
opacity: 1 ;
}
}
|
Output:
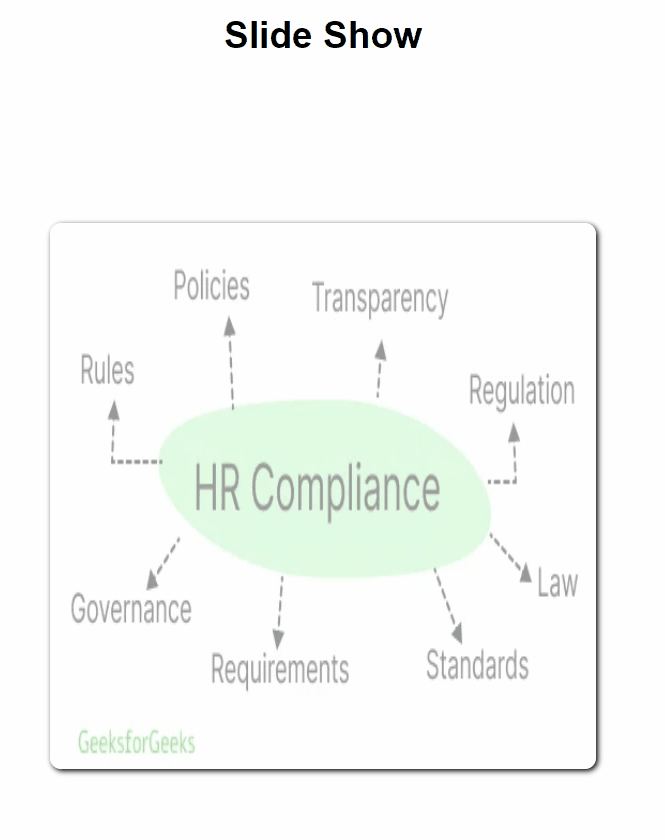
Share your thoughts in the comments
Please Login to comment...