AngularJS ReferenceError: $http is not defined
Last Updated :
19 Dec, 2023
The provides a control service named AJAX – $http, which serves the task of reading all the data that is available on the remote servers. The demand for the requirement of desired records gets met when the server makes the database call by using the browser. The data is mostly needed in JSON format. This is primarily because for transporting the data, JSON is an amazing method and it is straightforward & effortless to use within AngularJS, JavaScript, etc.
Suppose we created a service in our AngularJS project, and we are getting the error $http not defined. In this article, we will see the possible reasons and approaches to resolve that error.
Syntax
function controller($scope, $http) {
var url = "...";
$https:.get(url).success(function (response) {
// Implementation
});
}
Example: The following code example will produce an error, ie, AngularJS ReferenceError: $http is not defined.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
AngularJS AJAX - $http
</ title >
< script src =
</ script >
</ head >
< body >
< h2 style = "color:green" >GeeksforGeeks</ h2 >
< h2 >AngularJS ReferenceError: $http is not defined</ h2 >
< div ng-app = "" ng-controller = "studentController" >
< table >
< tr >
< th >User ID</ th >
< th >Title</ th >
< th >Body</ th >
</ tr >
< tr ng-repeat = "student in students" >
< td >{{ student.userId }}</ td >
< td >{{ student.title}}</ td >
< td >{{ student.body}}</ td >
</ tr >
</ table >
</ div >
< script >
function studentController($scope) {
$http.get(url).then(function (response) {
$scope.students = response.data;
});
}
</ script >
</ body >
</ html >
|
Output:
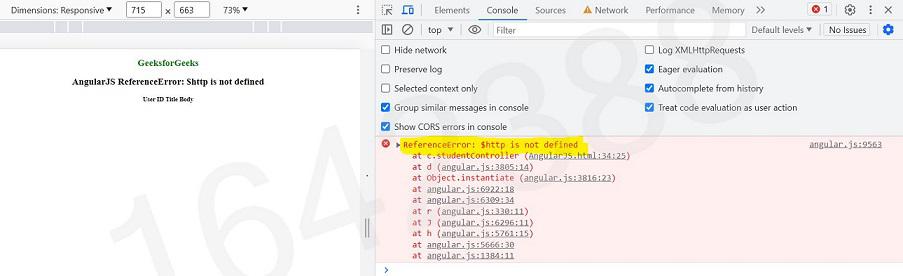
Explanation
This error is there because we have not imported the $http service, ie., AngularJS $http service has not been properly injected into the controller or service where we are trying to implement it. For eg, suppose we want to use a function from any library, then we need to import the library in our project then only we can use that function.
To resolve this issue, we need to inject the $http service into the controller or service before invoking & implementing it. Here, as we haven’t injected the $http service into our controller, we need to import/inject it like the below syntax:
function MyController($scope, $http) {
// ... Your code
}
Example: In this example, just like to use any function from a library, we need to import that library. So, to use the $http service, we need to inject it.
HTML
<!DOCTYPE html>
< html >
< head >
< title >AngularJS AJAX - $http</ title >
< script src =
</ script >
</ head >
< body >
< h2 style = "color:green" >GeeksforGeeks</ h2 >
< h2 >AngularJS ReferenceError: $http is not defined</ h2 >
< div ng-app = "" ng-controller = "studentController" >
< table >
< tr >
< th >User ID</ th >
< th >Title</ th >
< th >Body</ th >
</ tr >
< tr ng-repeat = "student in students" >
< td >{{ student.userId }}</ td >
< td >{{ student.title}}</ td >
< td >{{ student.body}}</ td >
</ tr >
</ table >
</ div >
< script >
function studentController($scope, $http) {
$http.get(url).then(function (response) {
$scope.students = response.data;
});
}
</ script >
</ body >
</ html >
|
Output:
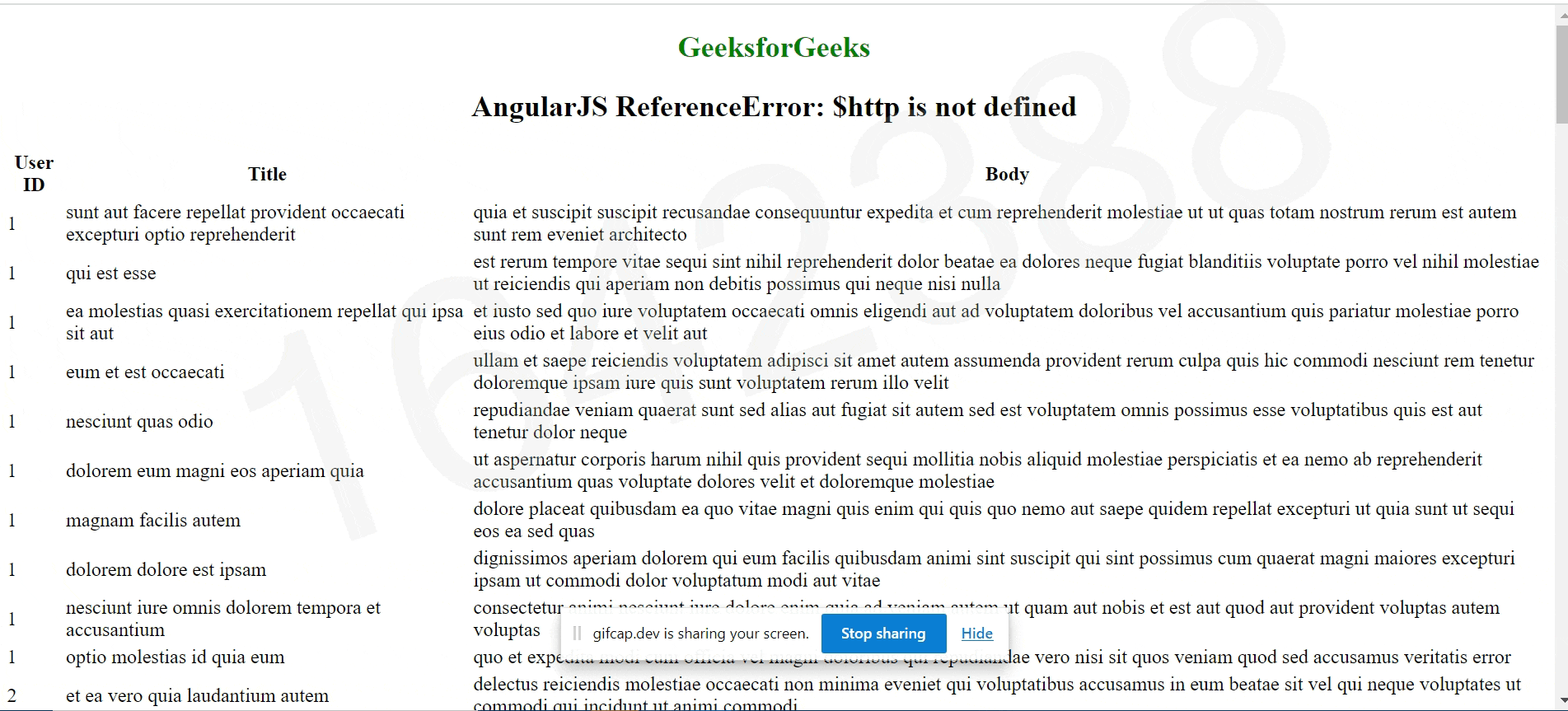
Share your thoughts in the comments
Please Login to comment...