Angular ngx Bootstrap
Last Updated :
27 Sep, 2023
Angular ngx Bootstrap is an open-source independent project that provides Bootstrap components powered by Angular. It is basically a bootstrap framework used with angular to create components with great styling and this framework is very easy to use and is used to make responsive websites. With the help of ngx Bootstrap, we can make an interactive web application that enhances the overall interactivity of the web app.
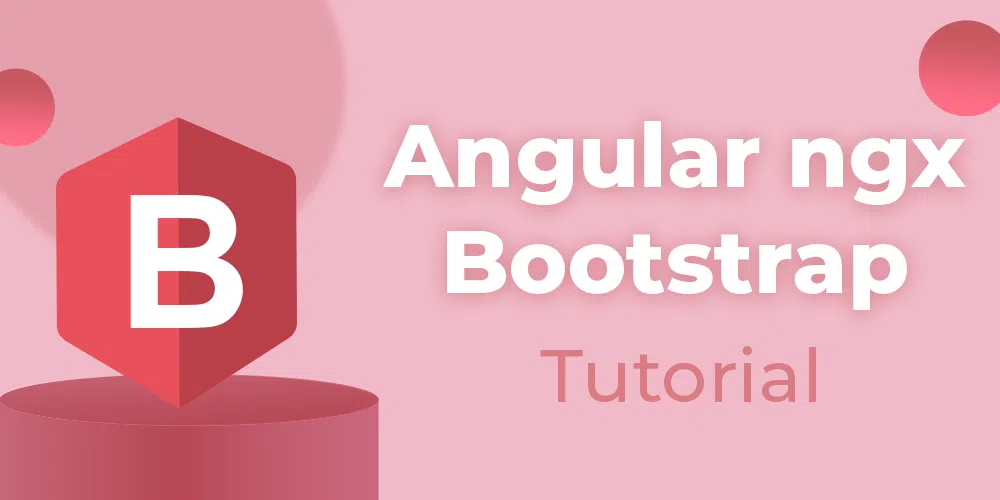
Angular ngx Bootstrap
In order to utilize the ngx Bootstrap in the Angular project, we can implement either of the technique to install:
- Installing using Angular CLI
- Installing using the Manual way with npm
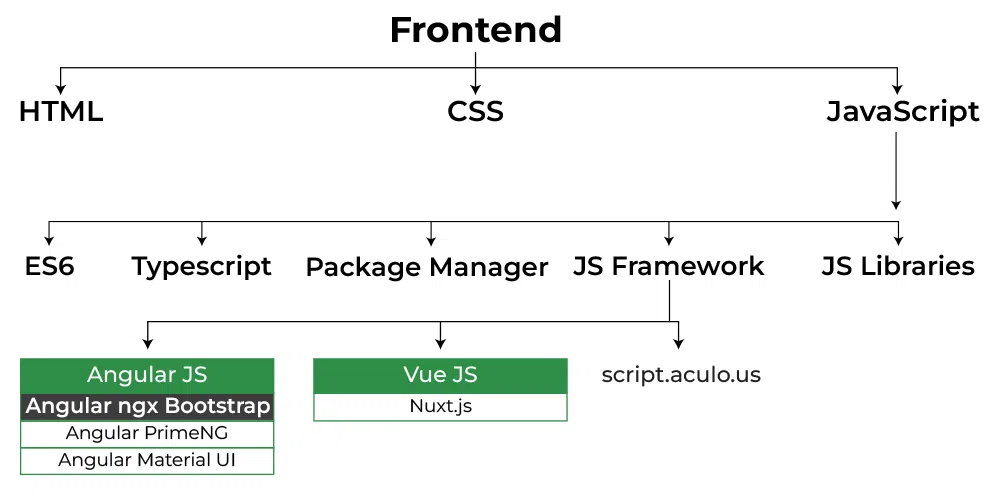
Angular ngx Bootstrap
We will understand both the installation procedures, along with knowing their implementation through the examples.
Installing using the Manual way through npm: Before we proceed to install the Angular ngx Bootstrap, we must have installed the Angular CLI in the system. Please refer to the Angular CLI Angular Project Setup article for the detailed installation procedure.
Make sure the Angular CLI & Node Package Manager is installed properly. To check the version installed, run the below commands:
node --version
npm -V OR npm --version
ng -V or ng --version
We will follow the below steps to install the Angular ngx Bootstrap.
Step1: Installation syntax: Run the below command to install the ngx Bootstrap:
npm install ngx-bootstrap --save
Step 2: After successful installation, add the required package to NgModule imports in the app.module.ts file, as given below:
import { AccordionModule } from 'ngx-bootstrap/accordion';
@NgModule({
...
imports: [ AccordionModule.forRoot(), … ]
...
})
Step 3: Add the following <link> tag in index.html file:
<link href=”https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css” rel=”stylesheet”>
Step 4: Add the component in the app.component.html file:
<accordion [isAnimated]="true">
<accordion-group heading= "Content">
Text
</accordion-group>
</accordion>
Step 5: Run the app using the following command:
ng serve
It will render the application to the http://localhost:4200/ in the browser.
Installing using Angular CLI:
We can directly add the Boostrap to the Angular project using the ng add command that will update the Bootstrap module in the project.
ng add ngx-bootstrap
Project Structure: After successful installation, the project will contain the following modules & components:
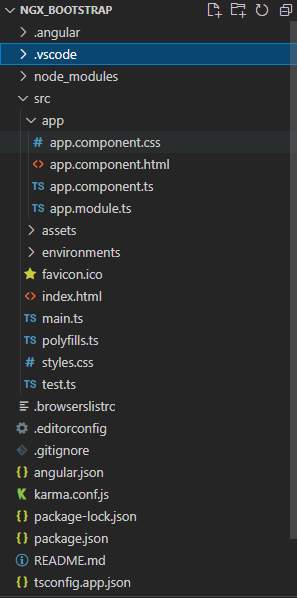
Project Structure
Example: This example illustrates the implementation of Angular ngx Bootstrap in the Angular project.
index.html:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<base href="/" />
<meta name="viewport"
content="width=device-width,
initial-scale=1" />
<link href=
"https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
rel="stylesheet" />
<link rel="icon"
type="image/x-icon"
href="favicon.ico" />
<link rel="preconnect"
href="https://fonts.gstatic.com" />
<link href=
"https://fonts.googleapis.com/css2?family=Roboto:wght@300;400;500&display=swap"
rel="stylesheet" />
<link href=
"https://fonts.googleapis.com/icon?family=Material+Icons"
rel="stylesheet" />
</head>
<body class="mat-typography">
<app-root></app-root>
</body>
</html>
app.component.html:
HTML
<div class="container">
<h1>GeeksforGeeks</h1>
<h3>Angular ngx Bootstrap</h3>
<accordion [isAnimated]="true">
<accordion-group heading="Data Structure">
<p>
The one-stop solution is GeeksforGeeks
DSA Self-Paced Course with Lifetime Access
is a complete package for you to learn and
master all the concepts.
</p>
<a href=
"https://www.geeksforgeeks.org/data-structures/">
Click Here
</a>
</accordion-group>
<accordion-group heading="Web Technology">
<p>
Web Technology refers to the various tools
and techniques that are utilized in the process
of communication between different types of
devices over the internet.
</p>
<a href=
"https://www.geeksforgeeks.org/web-technology//">
Click Here
</a>
</accordion-group>
<accordion-group heading="Aptitude">
<p>
A Computer Science portal for geeks.
It contains well-written, well thought
and well explained computer science and
programming articles.
</p>
<a href=
"https://www.geeksforgeeks.org/aptitude-questions-and-answers/">
Click Here
</a>
</accordion-group>
<accordion-group heading="Placement Course">
<p>
The placement session for any company generally
has 3 – 4 rounds. The first round is the written
test, consisting of quantitative aptitude.
</p>
<a href=
"https://www.geeksforgeeks.org/placements-gq/">
Click Here
</a>
</accordion-group>
</accordion>
</div>
app.component.ts:
Javascript
import { Component, OnInit, LOCALE_ID } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {}
app.component.css:
CSS
.container {
font-family: 'Arial';
margin-top: 15px;
margin-left: 10px;
margin-right: 10px;
text-align: justify;
}
h1 {
color: green;
text-align: center;
}
h3 {
text-align: center;
}
app.module.ts:
Javascript
import { NgModule } from "@angular/core";
import { FormsModule, ReactiveFormsModule } from "@angular/forms";
import { BrowserModule } from "@angular/platform-browser";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
import { AccordionModule } from "ngx-bootstrap/accordion";
import { AppComponent } from "./app.component";
@NgModule({
bootstrap: [AppComponent],
declarations: [AppComponent],
imports: [
FormsModule,
BrowserModule,
BrowserAnimationsModule,
ReactiveFormsModule,
AccordionModule.forRoot(),
],
})
export class AppModule{}
Output:
Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above
Share your thoughts in the comments
Please Login to comment...