Anagram Count using HTML CSS and JavaScript
Last Updated :
22 Sep, 2023
In this article, we will create an Anagram Counting app using HTML, CSS, and JavaScript. Anagrams are the words of rearranged characters or letters in random order. These words contain the same letters. These rearranged words on sorting result in the same words with the same letters used.
Consider the below example to check whether the specific word is an anagram or not with their count:
Input: forxxorfxdofr
for
Output: 3
Explanation: Anagrams of the word for – for, orf, ofr appear in the text and hence the count is 3.
Here, we will take the input string and input word from the user and count the number of possible anagrams of that word in the string. It will show the number and also which anagrams of the word exist as the output.
Prerequisites:
The prerequisites of this application are
Approach:
- First, create a Web page basic structure using HTML tags like inputs and labels for taking user input, a button to submit that input, and <h3> and <p> tags for output text. Also, use divs and span for outer body structure along with relevant Class names and IDs.
- Style the web page using the class names with CSS properties like box-shadow for card view, margins, padding to add space, text-align for alignment, and display type for display styling of individual elements.
- In JavaScript, create a function fun that triggers when the submit button is clicked. Calculate and show the existing anagrams of the word in the given string.
- Inside this function use HTML IDs to access the elements for taking user inputs and showing outputs as shown above in the sample image.
Example: The below example illustrates to check the Anagram of the string using JavaScript.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 " />
< link href = "style.css"
rel = "stylesheet" />
</ head >
< body >
< div class = "root" >
< h1 >Count Anagrams</ h1 >
< div class = "option" >
< span >
< label for = "inputString" >
Input String
</ label >
< input id = "inputString"
type = "text"
value = "forxxorfxdofr" />
</ span >
< span >
< label for = "inputWord" >
Enter
</ label >
< input id = "inputWord"
type = "text"
value = "for" />
</ span >
< span style = "text-align: center; width: 50%" >
< input style = "text-align: center; width: 50%;"
type = "button"
value = "Submit"
onclick = "fun()" />
</ span >
</ div >
< h3 id = "nums" ></ h3 >
< p id = "explain" ></ p >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
* {
box-sizing: border-box;
margin : 2% ;
}
body {
text-align : center ;
margin : auto ;
display : flex;
flex- direction : column;
}
.root {
margin-top : 5% ;
display : flex;
flex- direction : column;
width : 30 rem;
margin : auto ;
margin-top : 5% ;
box-shadow: 0 4px 10px rgb ( 46 , 63 , 57 );
background-color : rgb ( 122 , 199 , 173 );
border-radius: 0 10px ;
padding : 3% ;
}
.option {
text-align : left ;
display : flex;
flex- direction : column;
font-size : x-large ;
padding : 2% ;
margin : auto ;
}
.option>span {
display : flex;
padding : 2% ;
}
span>label {
width : 50% ;
}
input {
width : 50% ;
font-size : large ;
}
|
Javascript
inputString.value = "" ;
inputWord.value = "" ;
nums.innerText = "" ;
explain.innerText = "" ;
function fun() {
let count = 0;
let res = [];
str = inputString.value;
word = inputWord.value;
if (str === "" || word === "" ) {
window.alert( "Incorrect input!" );
return ;
}
let n = str.length;
let wordLen = word.length;
srtWord = word.split( "" ).sort().join( "" );
for (let i = 0; i < n - wordLen + 1; ++i) {
let sub = str
.slice(i, i + wordLen)
.split( "" )
.sort()
.join( "" );
if (sub === srtWord) {
count += 1;
res.push( "'" + str.slice(i, i + wordLen) + "'" );
}
}
let explainres;
if (count === 0) explainres = "none" ;
else explainres = res.join( "," );
nums.innerText =
"Input string contains " + count + " Anagrams" ;
explain.innerText =
"Anagrams of the word '" +
word +
"' are: " +
explainres;
}
|
Output:
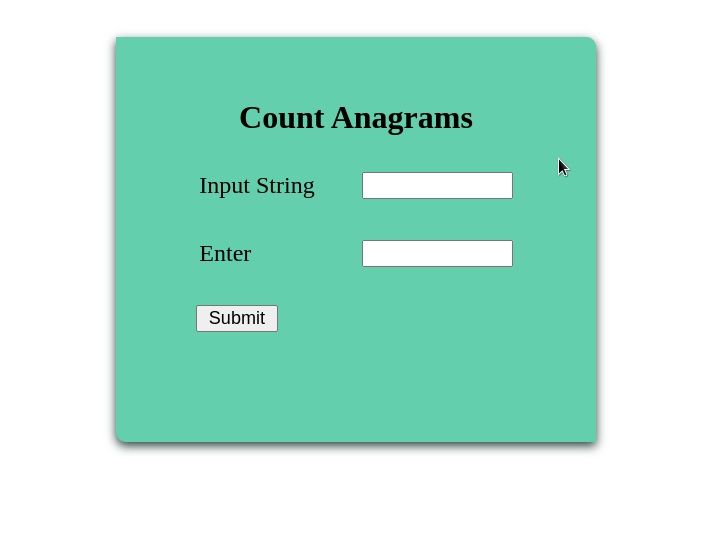
Share your thoughts in the comments
Please Login to comment...