0-1 BFS Algorithm for Competitive Programming
Last Updated :
28 Apr, 2024
0-1 BFS Algorithm is a variation of simple BFS and is used to calculate Single vertex_source Shortest Path to all the nodes in a weighted graph provided the edges have weights 0 and 1 only. This algorithm runs in O(|E|) time which is much faster as compared to the Single Source Shortest Path Algorithms.
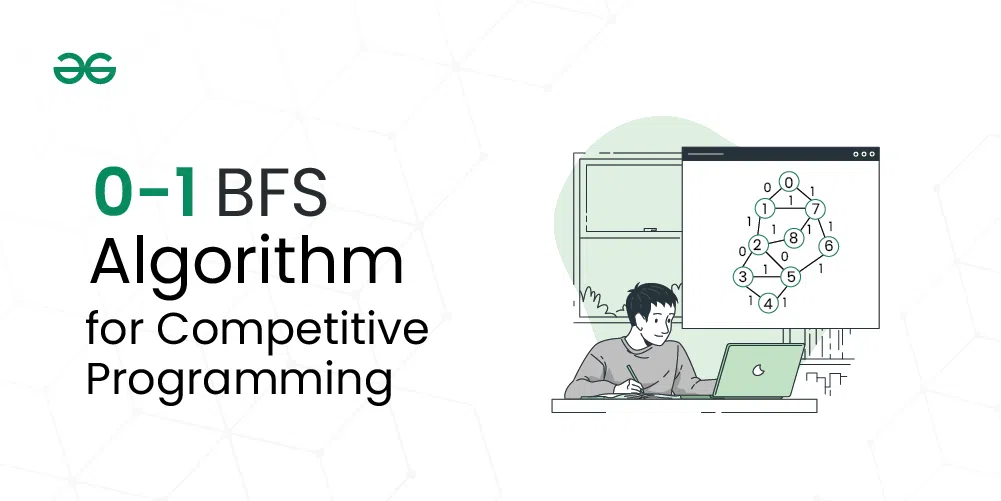
Why to use 0-1 BFS Algorithm?
Using a simple BFS, we can find the minimum distance from a vertex_source node to all other nodes in O(|E|) time provided the graph is an unweighted graph or all the edges have the same weight. In case, the edges have different weights, then we can find the minimum distance to all nodes using Dijkstra’s Algorithm, Bellman-Ford or Shortest Path Faster Algorithm. But, if there is a constraint on the edge weights that they can only be 0 or 1, then applying the above-mentioned algorithms would be an overkill as Dijkstra’s Algorithm runs in O(|E| * log|V|) time and Bellman Ford and SPFA runs in O(|V| * |E|). So, if a graph has edges with weights 0 and 1 only, then we can calculate the minimum distance from a vertex_source node to all the other nodes in O(|E|) time using the 0-1 BFS Algorithm.
Idea behind 0-1 BFS Algorithm:
The idea of 0-1 BFS is similar to Dijkstra’s Algorithm. In the Dijkstra’s Algorithm, we maintain a priority queue or a Min Heap to keep track of all the nodes which can be relaxed in future to get the minimum distance. Initially, we push the vertex_source node with its distance as 0 and then pop it and push all its neighbors who can be relaxed in the future. We keep on doing the same till there is no node to relax further.
Observation in 0-1 BFS Algorithm:
If at any point, we pop a node X which has a distance D from the vertex_source node, then all the nodes which are currently present in the priority queue will have a distance of either D or D+1.
Proof for the above observation:
Since the priority queue is a min heap, therefore no node in the priority queue can have distance less than D, otherwise we would have popped that node first. As all the nodes which were popped before Node X were having the maximum distance as D, so the neighbors of those nodes which still might be there in the priority queue can have the maximum distance as D+1 (as the max edge weight in the graph is 1). In other words, in order to have a node in the priority queue with has a distance greater than D+1, we should have popped a node with distance D+1 before this node X, which is impossible in case of min heap.
Now, since we have proved that at any point all the nodes will have a distance of D or D+1, then we can maintain a deque to store the nodes. If we are popping a node X with distance D, all the neighboring nodes connected with an edge weight of 1 will be pushed at the back of the deque and all the neighboring nodes connected with an edge weight of 0 will be pushed at the front of the deque. This is done so that the neighbors of the nodes which are nearer to the vertex_source are relaxed before the nodes which are farther from the vertex_source.
Examples:
Input: V = 6, E = 7, vertex_source = 0, edges = [[0, 1, 1], [1, 2, 1], [2, 3, 1], [3, 4, 1], [4, 5, 0], [5, 0, 0], [1, 4, 1]]
Output: Distance[] = [0, 1, 2, 1, 0, 0]
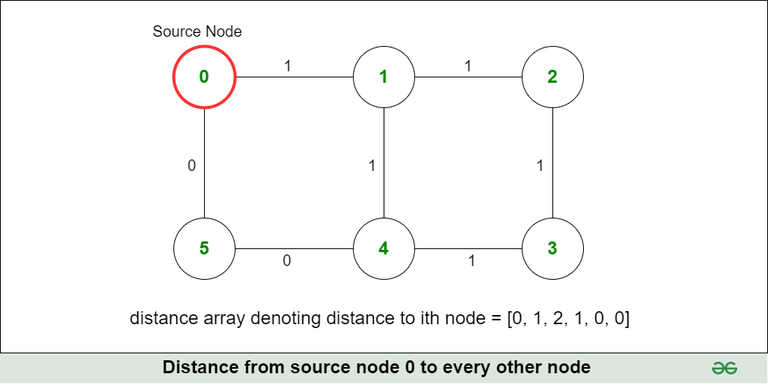
How to identify the problem based on 0-1 BFS Algorithm?
The problems based on 0-1 BFS Algorithm have one of the following patterns:
- All the edges in the graph have weights either 0 or w, where w is not equal to 0
- We have choices to make, where some choices have cost equal to 0, whereas the other choices have cost w, where w is not equal to 0, and we have to minimize the cost while performing these choices.
The following example would help us understand the concept of identification with the help of some problems:
Problem 1: Given a directed graph and a vertex_source node and destination node, we need to find how many edges we need to reverse in order to make at least 1 path from the vertex_source node to the destination node.
Solution 1: In the above problem, we can assign a weight of zero to all the original edges and for each of the original edges, we create a reversed edge with a weight of one. Now, the problem is reduced to find the path with smallest distance to a node where all the edges have weights either 0 or 1.
Problem 2: Given a matrix of size N X M, such that each cell has a number written on it. From one cell, we can move to any of the adjacent cells which share an edge with the current cell. If the neighboring cell has the same number as the original cell, then the move will cost us 0 coins, else the move will cost us W coins. Find the minimum number of coins required to move from cell (0,0) to cell (N-1, M-1).
Solution 2: In the above problem, we can start a bfs from (0,0) and start checking the neighboring cells. If the neighboring cell has same value, then push it at the front of the deque else push it at the back. We also need to keep a cost[][] array to store the minimum cost to reach a cell.
Problem 3: Given a matrix of size N * M, having some empty cells and some blocked cells. Now, at any cell if we continue to move in the same direction as we moved in the previous cell, then the cost is 0, else if we rotate by 90 degree we incur a cost of C coins. Initially, we are standing at cell (0, 0) and we can choose a direction (Up, Down, Left, Right) and start moving in that direction without any cost. At any time, we can either choose to move in the same direction (if the next cell is empty), else we can choose to rotate by 90 degrees. Find the minimum cost to reach cell (N-1, M-1).
Solution 3: We can check for all the directions as starting direction. Whenever, we are standing at any cell, we can make any of these four choices:
- Move in the same direction as we moved in the previous cell, hence cost = 0.
- Turn left, hence cost = C
- Turn right, hence cost = C
- Turn in the opposite direction (same as turning left or right twice), but this move is of no benefit to us because we are simply moving back one cell with a cost of 2 * C. So, we don’t move in opposite direction.
Now, if we move in the same direction, we push the new cell to the front of the deque else we push it at the back of the deque. We also need to keep a cost[][] array to store the minimum cost to reach a cell.
Implementation of 0-1 BFS Algorithm:
C++
#include <bits/stdc++.h>
using namespace std;
// Method to implement 0-1 BFS Algorithm
void bfs_01(vector<vector<pair<int, int> > >& graph,
vector<int>& dist, int vertex_source)
{
// Initializing the distance of vertex_source node
// from itself as 0
dist[vertex_source] = 0;
deque<int> dq;
dq.push_front(vertex_source);
while (!dq.empty()) {
int node = dq.front();
dq.pop_front();
// Checking all the neighbors for relaxation
for (auto edge : graph[node]) {
int childNode = edge.first;
int weight = edge.second;
// If the neighbor can be relaxed
if (dist[childNode] > dist[node] + weight) {
dist[childNode] = dist[node] + weight;
// If the edge weight is 1,
// push it at the back of deque
if (weight)
dq.push_back(childNode);
// If the edge weight is 0,
// push it at the front of deque
else
dq.push_front(childNode);
}
}
}
}
int main()
{
// Inputs
int V = 6, E = 7, vertex_source = 0;
vector<vector<pair<int, int> > > graph(V);
vector<vector<int> > edges{ { 0, 1, 1 }, { 1, 2, 1 },
{ 2, 3, 1 }, { 3, 4, 1 },
{ 4, 5, 0 }, { 5, 0, 0 },
{ 1, 4, 1 } };
// Representing the graph as adjacent list
for (auto edge : edges) {
graph[edge[0]].push_back({ edge[1], edge[2] });
graph[edge[1]].push_back({ edge[0], edge[2] });
}
// dist array to store distance of all nodes
// from vertex_source node
vector<int> dist(V, 1e9);
bfs_01(graph, dist, vertex_source);
for (int i = 0; i < V; i++)
cout << dist[i] << " ";
return 0;
}
Java
import java.util.ArrayDeque;
import java.util.Deque;
import java.util.LinkedList;
import java.util.List;
public class ZeroOneBFS {
// Method to implement 0-1 BFS Algorithm
static void bfs01(List<List<Pair>> graph, int[] dist, int vertex_source) {
// Initializing the distance of the vertex_source node
// from itself as 0
dist[vertex_source] = 0;
Deque<Integer> deque = new LinkedList<>();
deque.addFirst(vertex_source);
while (!deque.isEmpty()) {
int node = deque.pollFirst();
// Checking all the neighbors for relaxation
for (Pair edge : graph.get(node)) {
int childNode = edge.first;
int weight = edge.second;
// If the neighbor can be relaxed
if (dist[childNode] > dist[node] + weight) {
dist[childNode] = dist[node] + weight;
// If the edge weight is 1,
// push it at the back of the deque
if (weight == 1)
deque.addLast(childNode);
// If the edge weight is 0,
// push it at the front of the deque
else
deque.addFirst(childNode);
}
}
}
}
public static void main(String[] args) {
// Inputs
int V = 6, E = 7, vertex_source = 0;
List<List<Pair>> graph = new LinkedList<>();
List<int[]> edges = List.of(new int[]{0, 1, 1}, new int[]{1, 2, 1},
new int[]{2, 3, 1}, new int[]{3, 4, 1},
new int[]{4, 5, 0}, new int[]{5, 0, 0},
new int[]{1, 4, 1});
// Representing the graph as an adjacency list
for (int i = 0; i < V; i++) {
graph.add(new LinkedList<>());
}
for (int[] edge : edges) {
graph.get(edge[0]).add(new Pair(edge[1], edge[2]));
graph.get(edge[1]).add(new Pair(edge[0], edge[2]));
}
// dist array to store the distance of all nodes
// from the vertex_source node
int[] dist = new int[V];
for (int i = 0; i < V; i++) {
dist[i] = (int) 1e9;
}
bfs01(graph, dist, vertex_source);
for (int i = 0; i < V; i++) {
System.out.print(dist[i] + " ");
}
}
static class Pair {
int first, second;
Pair(int first, int second) {
this.first = first;
this.second = second;
}
}
}
// This code is contributed by rambabuguphka
Python3
from collections import deque
# Method to implement 0-1 BFS Algorithm
def bfs_01(graph, dist, vertex_source):
# Initializing the distance of vertex_source node
# from itself as 0
dist[vertex_source] = 0
dq = deque()
dq.appendleft(vertex_source)
while dq:
node = dq.popleft()
# Checking all the neighbors for relaxation
for edge in graph[node]:
childNode, weight = edge
# If the neighbor can be relaxed
if dist[childNode] > dist[node] + weight:
dist[childNode] = dist[node] + weight
# If the edge weight is 1,
# push it at the back of deque
if weight:
dq.append(childNode)
# If the edge weight is 0,
# push it at the front of deque
else:
dq.appendleft(childNode)
# Inputs
V = 6
E = 7
vertex_source = 0
graph = [[] for _ in range(V)]
edges = [[0, 1, 1], [1, 2, 1], [2, 3, 1], [3, 4, 1], [4, 5, 0], [5, 0, 0], [1, 4, 1]]
# Representing the graph as adjacent list
for edge in edges:
graph[edge[0]].append((edge[1], edge[2]))
graph[edge[1]].append((edge[0], edge[2]))
# dist array to store distance of all nodes
# from vertex_source node
dist = [float('inf')] * V
bfs_01(graph, dist, vertex_source)
for i in range(V):
print(dist[i], end=" ")
C#
using System;
using System.Collections.Generic;
public class ZeroOneBFS
{
// Method to implement 0-1 BFS Algorithm
static void BFS01(List<List<Pair>> graph, int[] dist, int vertex_source)
{
// Initializing the distance of the vertex_source node
// from itself as 0
dist[vertex_source] = 0;
LinkedList<int> deque = new LinkedList<int>();
deque.AddLast(vertex_source);
while (deque.Count > 0)
{
int node = deque.First.Value;
deque.RemoveFirst();
// Checking all the neighbors for relaxation
foreach (Pair edge in graph[node])
{
int childNode = edge.first;
int weight = edge.second;
// If the neighbor can be relaxed
if (dist[childNode] > dist[node] + weight)
{
dist[childNode] = dist[node] + weight;
// If the edge weight is 1,
// push it at the back of the deque
if (weight == 1)
deque.AddLast(childNode);
// If the edge weight is 0,
// push it at the front of the deque
else
deque.AddFirst(childNode);
}
}
}
}
public static void Main(string[] args)
{
// Inputs
int V = 6, vertex_source = 0;
List<List<Pair>> graph = new List<List<Pair>>();
List<int[]> edges = new List<int[]>
{
new int[] {0, 1, 1},
new int[] {1, 2, 1},
new int[] {2, 3, 1},
new int[] {3, 4, 1},
new int[] {4, 5, 0},
new int[] {5, 0, 0},
new int[] {1, 4, 1}
};
// Representing the graph as an adjacency list
for (int i = 0; i < V; i++)
{
graph.Add(new List<Pair>());
}
foreach (int[] edge in edges)
{
graph[edge[0]].Add(new Pair(edge[1], edge[2]));
graph[edge[1]].Add(new Pair(edge[0], edge[2]));
}
// dist array to store the distance of all nodes
// from the vertex_source node
int[] dist = new int[V];
for (int i = 0; i < V; i++)
{
dist[i] = int.MaxValue;
}
BFS01(graph, dist, vertex_source);
for (int i = 0; i < V; i++)
{
Console.Write(dist[i] + " ");
}
}
public class Pair
{
public int first, second;
public Pair(int first, int second)
{
this.first = first;
this.second = second;
}
}
}
Javascript
// Method to implement 0-1 BFS Algorithm
function bfs_01(graph, dist, vertex_source) {
// Initializing the distance of vertex_source node
// from itself as 0
dist[vertex_source] = 0;
let dq = [];
dq.push(vertex_source);
while (dq.length > 0) {
let node = dq.shift();
// Checking all the neighbors for relaxation
for (let edge of graph[node]) {
let childNode = edge[0];
let weight = edge[1];
if (dist[childNode] > dist[node] + weight) {
dist[childNode] = dist[node] + weight;
// If the edge weight is 1,
// push it at the back of deque
if (weight) {
dq.push(childNode);
}
// If the edge weight is 0,
// push it at the front of deque
else {
dq.unshift(childNode);
}
}
}
}
}
// Inputs
let V = 6, E = 7, vertex_source = 0;
let graph = Array.from({ length: V }, () => []);
// Representing the graph as adjacent list
let edges = [
[0, 1, 1], [1, 2, 1],
[2, 3, 1], [3, 4, 1],
[4, 5, 0], [5, 0, 0],
[1, 4, 1]
];
for (let edge of edges) {
graph[edge[0]].push([edge[1], edge[2]]);
graph[edge[1]].push([edge[0], edge[2]]);
}
// dist array to store distance of all nodes
// from vertex_source node
let dist = Array(V).fill(1e9);
bfs_01(graph, dist, vertex_source);
for (let i = 0; i < V; i++) {
process.stdout.write(dist[i] + " ");
}
Use case of 0-1 BFS Algorithm in Competitive Programming:
1. Minimum edges to reverse to make path from a source to a destination:
In this problem, we are given a unweighted directed graph, a vertex_source node and a destination node and we need to find the minimum number of edges we need to reverse to make a path from the vertex_source node to the destination node. This problem can be easily solved by making a fake edge in reverse direction for each of the original edge. All the original edges can be assigned a weight of 0 and all the fake edges can be assigned a weight of 1. Now, we simply use 0-1 BFS Algorithm to calculate the minimum distance between the vertex_source and the destination node. You can refer this article for the implementation.
2. Calculating minimum cost to move from one cell to another, such that the cost of moves is limited to 0 and W, where W > 0:
In this problem, we are given a matrix, and we need to move from one node start to another node end, with some allowed moves. Some of these moves cost us nothing whereas others cost us some fixed coins and we need to find the minimum amount to coins needed to reach the end node from the start node.
We can also extend 0-1 BFS Algorithm for a graph having multiple weights until all the edge weights are less than W, where W is a small integer. We can maintain K buckets in the queue and start popping from the front of the queue. This way, we start moving from smaller weight buckets to larger weight buckets.
Example :
C++
#include <cstring>
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// Structure to represent an edge
struct Edge {
int to;
int weight;
};
// Function to add an edge to the graph
void addEdge(vector<vector<Edge> >& graph, int from, int to,
int weight)
{
graph[from].push_back({ to, weight });
}
// Function to perform 0-1 BFS algorithm
int zeroOneBFS(vector<vector<Edge> >& graph, int source,
int destination)
{
const int INF = 1e9;
vector<int> distance(graph.size(), INF);
deque<int> dq;
// Push source node to the queue with distance 0
dq.push_front(source);
distance[source] = 0;
// Loop until the queue is empty
while (!dq.empty()) {
int node = dq.front();
dq.pop_front();
// Traverse all adjacent nodes of the current node
for (const Edge& edge : graph[node]) {
int newDistance = distance[node] + edge.weight;
if (newDistance < distance[edge.to]) {
distance[edge.to] = newDistance;
// If the weight of the edge is 0
// push to the front of the queue
if (edge.weight == 0) {
dq.push_front(edge.to);
}
// If the weight of the edge is 1
// push to the back of the queue
else {
dq.push_back(edge.to);
}
}
}
}
// Return the distance to destination node
return distance[destination];
}
int main()
{
// Example usage
int vertices = 5;
vector<vector<Edge> > graph(vertices);
// Add edges to the graph
addEdge(graph, 0, 2, 0);
addEdge(graph, 0, 2, 1);
addEdge(graph, 1, 3, 0);
addEdge(graph, 2, 3, 1);
addEdge(graph, 3, 4, 0);
// Find the minimum number of edges to reverse from node
// 0 to node 4
int source = 0, destination = 4;
int minEdgesToReverse
= zeroOneBFS(graph, source, destination);
cout << minEdgesToReverse << endl;
return 0;
}
Java
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Deque;
import java.util.List;
// Structure to represent an edge
class Edge {
int to;
int weight;
public Edge(int to, int weight)
{
this.to = to;
this.weight = weight;
}
}
public class Main {
// Function to add an edge to the graph
public static void addEdge(List<List<Edge> > graph,
int from, int to, int weight)
{
graph.get(from).add(new Edge(to, weight));
}
// Function to perform 0-1 BFS algorithm
public static int zeroOneBFS(List<List<Edge> > graph,
int source,
int destination)
{
final int INF = (int)1e9;
int[] distance = new int[graph.size()];
for (int i = 0; i < graph.size(); i++) {
distance[i] = INF;
}
Deque<Integer> dq = new ArrayDeque<>();
// Push source node to the queue with distance 0
dq.offerFirst(source);
distance[source] = 0;
// Loop until the queue is empty
while (!dq.isEmpty()) {
int node = dq.pollFirst();
// Traverse all adjacent nodes of the current
// node
for (Edge edge : graph.get(node)) {
int newDistance
= distance[node] + edge.weight;
if (newDistance < distance[edge.to]) {
distance[edge.to] = newDistance;
// If the weight of the edge is 0, push
// to the front of the queue
if (edge.weight == 0) {
dq.offerFirst(edge.to);
}
// If the weight of the edge is 1, push
// to the back of the queue
else {
dq.offerLast(edge.to);
}
}
}
}
// Return the distance to destination node
return distance[destination];
}
public static void main(String[] args)
{
int vertices = 5;
List<List<Edge> > graph = new ArrayList<>(vertices);
for (int i = 0; i < vertices; i++) {
graph.add(new ArrayList<>());
}
// Add edges to the graph
addEdge(graph, 0, 2, 0);
addEdge(graph, 0, 2, 1);
addEdge(graph, 1, 3, 0);
addEdge(graph, 2, 3, 1);
addEdge(graph, 3, 4, 0);
// Find the minimum number of edges to reverse from
// node 0 to node 4
int source = 0, destination = 4;
int minEdgesToReverse
= zeroOneBFS(graph, source, destination);
System.out.println(minEdgesToReverse);
}
}
Python3
from collections import deque
# Structure to represent an edge
class Edge:
def __init__(self, to, weight):
self.to = to
self.weight = weight
# Function to add an edge to the graph
def addEdge(graph, fromNode, to, weight):
graph[fromNode].append(Edge(to, weight))
# Function to perform 0-1 BFS algorithm
def zeroOneBFS(graph, source, destination):
INF = int(1e9)
distance = [INF] * len(graph)
dq = deque()
# Push source node to the queue with distance 0
dq.appendleft(source)
distance[source] = 0
# Loop until the queue is empty
while dq:
node = dq.pop()
# Traverse all adjacent nodes of the current node
for edge in graph[node]:
newDistance = distance[node] + edge.weight
if newDistance < distance[edge.to]:
distance[edge.to] = newDistance
# If the weight of the edge is 0, push to the front of the queue
if edge.weight == 0:
dq.appendleft(edge.to)
# If the weight of the edge is 1, push to the back of the queue
else:
dq.append(edge.to)
# Return the distance to destination node
return distance[destination]
# Example usage
vertices = 5
graph = [[] for _ in range(vertices)]
# Add edges to the graph
addEdge(graph, 0, 2, 0)
addEdge(graph, 0, 2, 1)
addEdge(graph, 1, 3, 0)
addEdge(graph, 2, 3, 1)
addEdge(graph, 3, 4, 0)
# Find the minimum number of edges to reverse from node 0 to node 4
source = 0
destination = 4
minEdgesToReverse = zeroOneBFS(graph, source, destination)
print(minEdgesToReverse)
# this code is contributed by Adarsh
JavaScript
// Define a class to represent an edge in the graph
class Edge {
constructor(to, weight) {
this.to = to; // Destination node of the edge
this.weight = weight; // Weight of the edge (0 or 1)
}
}
// Function to add an edge to the graph
function addEdge(graph, from, to, weight) {
graph[from].push(new Edge(to, weight)); // Add a new edge to the adjacency list of the 'from' node
}
// Function to perform the 0-1 BFS algorithm
function zeroOneBFS(graph, source, destination) {
const INF = 1e9; // Infinity value for representing unreachable distances
const distance = new Array(graph.length).fill(INF); // Array to store distances from the source node
const dq = []; // Deque (double-ended queue) for BFS traversal
dq.push(source); // Push the source node to the deque
distance[source] = 0; // Distance to the source node is 0
// Loop until the deque is empty
while (dq.length > 0) {
const node = dq.shift(); // Remove the first node from the deque
// Traverse all adjacent nodes of the current node
for (const edge of graph[node]) {
const newDistance = distance[node] + edge.weight; // Calculate the new distance to the adjacent node
// Update the distance if the new distance is shorter
if (newDistance < distance[edge.to]) {
distance[edge.to] = newDistance; // Update the distance to the adjacent node
// Push the adjacent node to the front or back of the deque based on the edge weight
if (edge.weight === 0) {
dq.unshift(edge.to); // Push to the front of the deque if the edge weight is 0
} else {
dq.push(edge.to); // Push to the back of the deque if the edge weight is 1
}
}
}
}
// Return the distance to the destination node
return distance[destination];
}
// Main function to execute the program
function main() {
const vertices = 5; // Total number of vertices in the graph
const graph = new Array(vertices).fill(null).map(() => []); // Initialize the adjacency list for the graph
// Add edges to the graph
addEdge(graph, 0, 2, 0);
addEdge(graph, 0, 2, 1);
addEdge(graph, 1, 3, 0);
addEdge(graph, 2, 3, 1);
addEdge(graph, 3, 4, 0);
// Find the minimum number of edges to reverse from node 0 to node 4
const source = 0, destination = 4; // Source and destination nodes for the BFS traversal
const minEdgesToReverse = zeroOneBFS(graph, source, destination); // Perform 0-1 BFS algorithm
console.log(minEdgesToReverse); // Output the result
}
// Call the main function to execute the program
main();
//This code is contributed by Prachi.
output :
1
Practice Problems on 0-1 BFS Algorithm for Competitive Programming:
Share your thoughts in the comments
Please Login to comment...