Check if a File is Hidden in Java
Last Updated :
18 Apr, 2022
isHidden() method of File class in Java can use be used to check if a file is hidden or not. This method returns a boolean value – true or false.
Syntax:
public static boolean isHidden(Path path)
throws IOException
Parameters: Path to the file to test.
Return Type: A boolean value, true if file is found hidden else returns false as a file is not found hidden
Exceptions Thrown:
- IOException: If an I/O error occurs
- SecurityException: In the case of the default provider, and a security manager is installed, the checkRead() method is invoked to check read access to the file.
Remember: Depending on the implementation the isHidden() method may require to access the file system to determine if the file is considered hidden.
Example:
Java
import java.io.File;
import java.io.IOException;
public class GFG {
public static void main(String[] args)
throws IOException, SecurityException
{
File file = new File(
"/users/mayanksolanki/Desktop/demo.rtf" );
if (file.isHidden())
System.out.println(
"The specified file is hidden" );
else
System.out.println(
"The specified file is not hidden" );
}
}
|
Output:
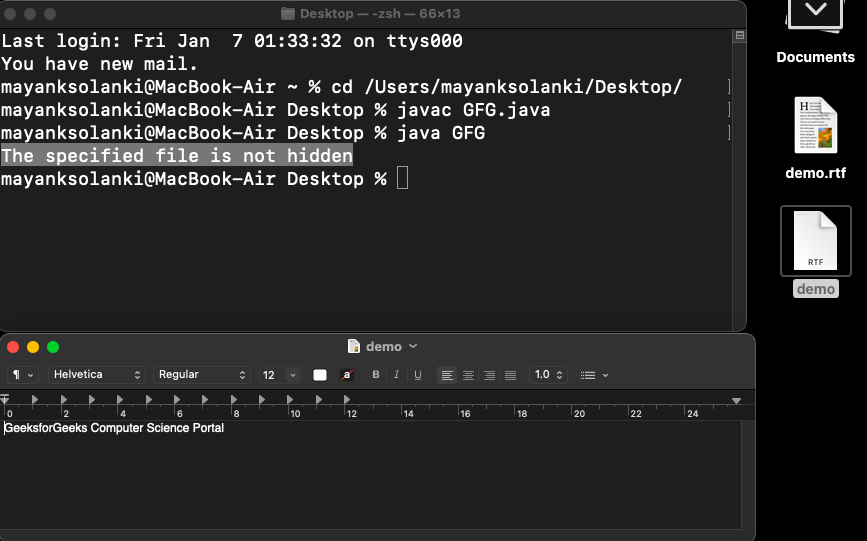
Output Explanation: As it can easily be visualized from the background of the output that the ‘demo.rtf’ file popping icon is clearly seen. The code reflects that a specific file is not hidden on the terminal output as seen above.
Note: The precise definition of hidden is a platform or provider-dependent.
- UNIX: A file is hidden if its name begins with a period character (‘.’).
- Windows: A file is hidden if it is not a directory and the DOS hidden attribute is set.
Share your thoughts in the comments
Please Login to comment...