YACC program for Conversion of Infix to Postfix expression
Last Updated :
02 May, 2019
Problem: Write a YACC program for conversion of Infix to Postfix expression.
Explanation:
YACC (Yet another Compiler-Compiler) is the standard parser generator for the Unix operating system. An open source program, yacc generates code for the parser in the C programming language. The acronym is usually rendered in lowercase but is occasionally seen as YACC or Yacc.
Examples:
Input: a*b+c
Output: ab*c+
Input: a+b*d
Output: abd*+
Lexical Analyzer Source Code:
%{
%}
ALPHA [A-Z a-z]
DIGIT [0-9]
%%
{ALPHA}({ALPHA}|{DIGIT})* return ID;
{DIGIT}+ {yylval= atoi (yytext); return ID;}
[\n \t] yyterminate();
. return yytext[0];
%%
|
Parser Source Code:
%{
#include <stdio.h>
#include <stdlib.h>
%}
%token ID
%left '+' '-'
%left '*' '/'
%left UMINUS
%%
S : E
E : E '+' {A1();}T{A2();}
| E '-' {A1();}T{A2();}
| T
;
T : T '*' {A1();}F{A2();}
| T '/' {A1();}F{A2();}
| F
;
F : '(' E{A2();} ')'
| '-' {A1();}F{A2();}
| ID{A3();}
;
%%
#include"lex.yy.c"
char st[100];
int top=0;
int main()
{
printf ( "Enter infix expression: " );
yyparse();
printf ( "\n" );
return 0;
}
A1()
{
st[top++]=yytext[0];
}
A2()
{
printf ( "%c" , st[--top]);
}
A3()
{
printf ( "%c" , yytext[0]);
}
|
Output:
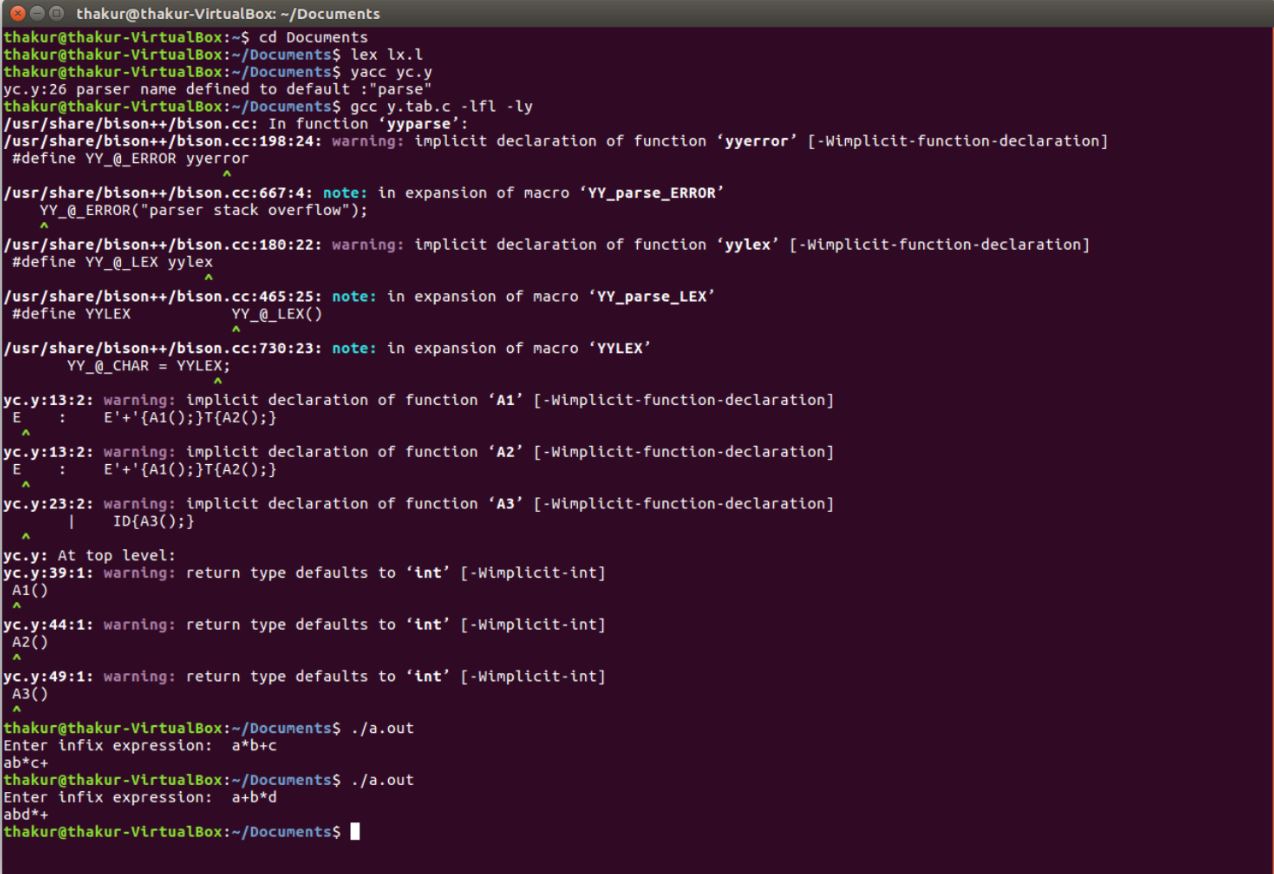
Share your thoughts in the comments
Please Login to comment...