When should we use curly braces for ES6 import ?
Last Updated :
12 Apr, 2023
The ES6 module can be imported with or without curly braces, making it essential to understand the scenarios in which curly braces should be used. By clarifying when curly braces are necessary for importing ES6 modules, developers can ensure their code is written efficiently and with accuracy.
There are two types of exports in ES6:
Default Export:
export default App; //example of default export
...
import App from path...
Named Export: The curly braces {} is ES6 is used to import the Named Exports
export Gfg = 'GeeksforGeeks' //example of named export
...
import {Gfg} from path...
ES6 modules are JavaScript built-in module formats. The module contains a variable, function, class, or object. If we want to access these in other files or modules, we use keywords like export and import.
ES6 import and export:
Import: You can import variables, functions, or classes using the import keyword.
Syntax:
import x from “path_to_js_file”;
// If you want to import all the members at once
// then you can do that using a ' * ' symbol.
import * as exp from "./convert.js";
Export: We can export values from a JavaScript module using export declaration. Here values refer to variables, functions, classes, or objects.
Syntax for variable:
export let variable_A = 10;
Syntax for function:
export function fun_A() { /* … */ };
Syntax for class:
export class Class_Name {
constructor() {
// Statements
}
}
Steps to run:
Step 1: Create a file named convert.js, in which we will create a function named convert_dollar_to_rupee which will convert dollar amount to rupees.
Step 2:
- Create a file named index.html, we will create a simple webpage with one input box and button.
- First, we will import the function convert_dollar_to_rupee which will convert the dollar value to the rupee.
- We will display the output as an alert on our webpage.
Example 1: Let’s create a currency converter application, in which we will convert dollars to the rupee.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Currency Converter</ title >
< style >
h2 {
text-align: center;
}
.convert {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
input,
select {
padding: .8em;
margin-bottom: 1%;
font-family: sans-serif;
}
select {
cursor: pointer;
}
button {
padding: .5em;
font-size: .8rem;
cursor: pointer;
margin-top: 1%;
}
</ style >
</ head >
< body >
< h2 >Currency Conversion</ h2 >
< div class = "convert" >
< input type = "number" id = "amount"
placeholder = "Enter Amount" >
< button id = "convertButton" >
Convert
</ button >
< button id = "exchangeRate" >
Exchange Rate
</ button >
</ div >
</ body >
< script type = "module" >
import convert_dollar_to_rupee, { MULTIPLIER } from './convert.js';
const amountInput = document.getElementById("amount");
const choice = document.getElementById("choice");
const convertButton = document.getElementById("convertButton");
const exchangeRateButton = document.getElementById("exchangeRate");
let exchangeRate = MULTIPLIER;
// Add event listener to button
convertButton.addEventListener('click', () => {
let amount = amountInput.value;
// Display
alert(convert_dollar_to_rupee(amount));
// Clear the amount input
amountInput.value = "";
})
// Display Exchange Rate
exchangeRateButton.addEventListener("click", () => {
let str = `1 US Dollar equals ${exchangeRate}`;
alert(str); //82.77
})
</ script >
</ html >
|
Javascript
export const MULTIPLIER = 82.77;
export default function (amount) {
return amount * MULTIPLIER;
}
|
Output:
In this example type of export implemented in the convert.js file is called named export. In this case, we need to add curly braces and make sure that the import name should be same as the export because you’re importing a specific thing by its export name.
Example 2:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Currency Converter</ title >
< style >
h2 {
text-align: center;
}
.convert {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
input {
padding: .8em;
margin-bottom: 1%;
font-family: sans-serif;
}
button {
padding: .5em;
font-size: .8rem;
cursor: pointer;
}
</ style >
</ head >
< body >
< h2 >Rupee to Dollar Conversion</ h2 >
< div class = "convert" >
< input type = "number" id = "amount"
placeholder = "Enter Amount" >
< button id = "convertButton" >Convert</ button >
</ div >
< script type = "module" >
import convert_dollar_to_rupee from './convert.js';
const amountInput = document.getElementById("amount");
const convertButton = document.getElementById("convertButton");
// Add event listener to button
convertButton.addEventListener('click', () => {
let amount = amountInput.value;
// Display
alert(convert_dollar_to_rupee(amount));
// Clear the amount input
amountInput.value = "";
})
</ script >
</ body >
</ html >
|
Javascript
const MULTIPLIER = 82.77;
export default function (amount) {
return amount * MULTIPLIER;
}
|
Output:
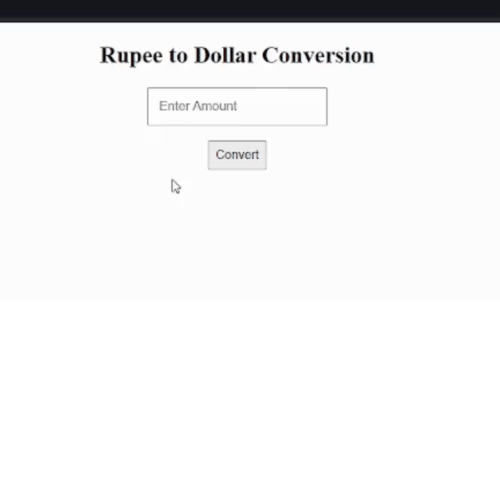
When should I use curly braces for ES6 import?
Note: In this case, it doesn’t matter what name you assign to it when importing, Because it will always resolve to whatever is the default export of convert.js.
Share your thoughts in the comments
Please Login to comment...