What’s the difference between forceUpdate vs render in ReactJS ?
Last Updated :
29 Nov, 2023
In this article, we will learn about the difference between forceUpdate vs render in ReactJS. In ReactJS, both forceUpdate
and render
are related to updating the UI, but they serve different purposes.
Prerequisites:
render():
In React, the render() method is most important when you are working with a class Component. Without this method, a class component cannot return the value. All the HTML code is written inside the render() method. render() is a part of the React component lifecycle method. It is called at different app stages. E.g. When the component is first made or ready.
Example: Now write down the following code in the App.js file.
App.js
import React,{ Component } from 'react' ;
class App extends Component {
render() {
return (
<div>
<h1 style={{color: "green" }}>
Learn React from GFG!!
</h1>
</div>
)
}
}
export default App;
|
Output:
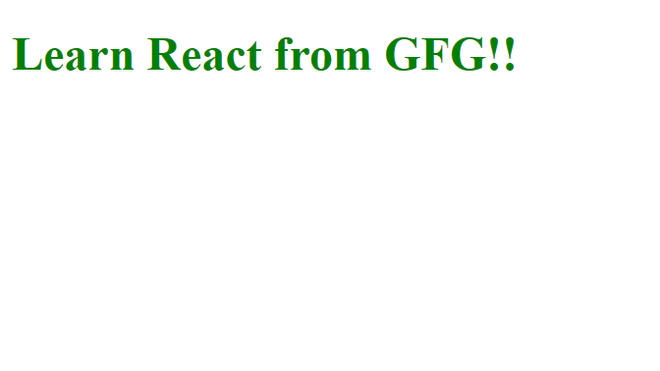
forceUpdate():
In React, when the props and state of the component are changed the component automatically re-render but when the component is dependent on some data other than state and props. In that situation forceUpdate is called to tell that component requires re-rendering. Calling forceUpdate() will forcibly re-render the component and thus calls the render() method on the component skipping the shouldComponentUpdate() method.
Example: Now write down the following code in the App.js file.
App.js
import React from 'react' ;
class App extends React.Component{
forceUpdateHandler=()=>{
this .forceUpdate();
};
render(){
console.log( 'Component is re-rendered' );
return (
<div>
<h3 style={{backgroundColor: "green" }>
Example of forceUpdate()
method to show re-rendering
</h3>
<button onClick= { this .forceUpdateHandler} >
FORCE UPDATE
</button>
<h4>Random Number :
{ Math.floor(Math.random() * (100 - 1 +1)) + 1 }
</h4>
</div>
);
}
}
export default App;
|
Output:
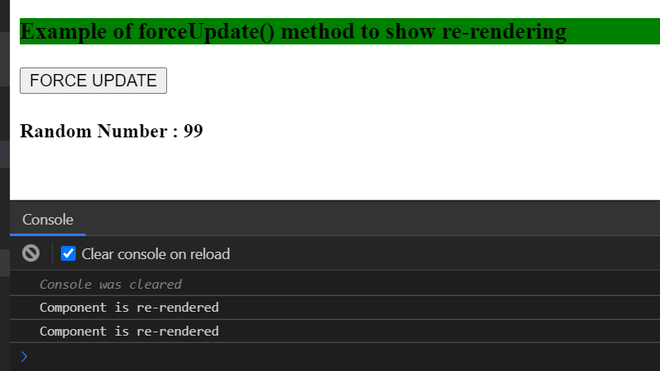
ForceUpdate
Difference between forceUpdate vs render:
render()
|
forceUpdate()
|
This method is called in the following conditions:
- When a component is instantiated.
- When state or props is updated.
|
It will be called when some data changes other than state or props. |
It does not skip any lifecycle method. |
It skips the lifecycle shouldComponentUpdate method. |
It is not user-callable. |
It is called manually. |
It automatically re-renders whenever required. |
It re-render the component forcefully. |
It is compulsory to use as it is the only required method in the Class component. |
It is not recommended to use. It should be avoided. |
Share your thoughts in the comments
Please Login to comment...