What is {this.props.children} and when you should use it ?
Last Updated :
30 Oct, 2023
{this.props.children} refers to children that are a special prop that is used to pass the data from the parent component to the children component but this data must be enclosed within the parent’s opening and closing tag. This is used mostly in some wrapper components by which we have to pass the data onto the next component and also the data which we pass its static data ( in most cases ) because for dynamic data there is another way to pass props to the component.
Prerequisites
Approach
{this.props.children} is a way to pass the state data to a component as the props. This method is used in React JS class-based components to pass props and access that data in the child component.
For example, consider the code snippet below which shows how to pass dynamic data to any component. Now we’ll understand the usage of {this.props.children } with an example.
<SomeComponent title={state.Title} description={state.Description} />
Creating React Application
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder, move into it using the following command:
cd foldername
Project structure
It will look like this.
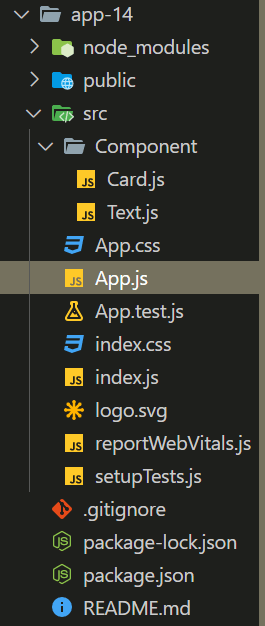
Example: This example demonstrates the passing of data form parent to card component and render using {this.prop.children} in class components.
Javascript
import "./App.css" ;
import Card from "./Component/Card" ;
function App() {
let Projects = [
{
Topic: "Python projects" ,
title: "Make notepad using Tkinter" ,
},
{
Topic: "Python projects" ,
title: "Color game using Tkinter in Python" ,
},
{
Topic: "OpenCV projects" ,
title: "OpenCV C++ Program for Face Detection" ,
},
{
Topic: "OpenCV projects" ,
title: "OpenCV C++ Program for coin detection" ,
},
{
Topic: "Java projects" ,
title: "Generating Password and OTP in Java" ,
},
{
Topic: "Java projects" ,
title: "A Group chat application in Java" ,
},
];
return (
<div className= "App" >
<h1 className= "geeks" >GeeksforGeeks</h1>
<h3>
React Example for { "{this.props.children}" }
</h3>
<div className= "container" >
{Projects.map((project, idx) => {
return (
<div className= "item" >
<Card
Topic={project.Topic}
URL={project.URL}
title={project.title}
key={idx}
/>
</div>
);
})}
</div>
</div>
);
}
export default App;
|
Javascript
import React, { Component } from "react" ;
import Text from "./Text" ;
export class Card extends Component {
render() {
return (
<div
>
<h3> { this .props.Topic} </h3>
<Text>
<a
href={ this .props.URL}
style={{
textDecoration: "none" ,
color: "black" ,
}}
target= "_blank"
>
{ this .props.title}
</a>
</Text>
</div>
);
}
}
export default Card;
|
Javascript
import React, { Component } from "react" ;
export class Text extends Component {
render() {
return <h6>{ this .props.children}</h6>;
}
}
export default Text;
|
CSS
.App {
text-align : center ;
margin : auto ;
}
.geeks {
color : green ;
}
.container {
padding : 2% ;
display : flex;
flex- direction : row;
flex-wrap: wrap;
}
.item {
width : 20 rem;
border : 2px solid green ;
margin : 5px ;
padding : 2px ;
}
|
Steps to Run the Application: Use this command in the terminal in project directory.
npm start
Output: This output will be visible on http://localhost:3000/ on browser window.
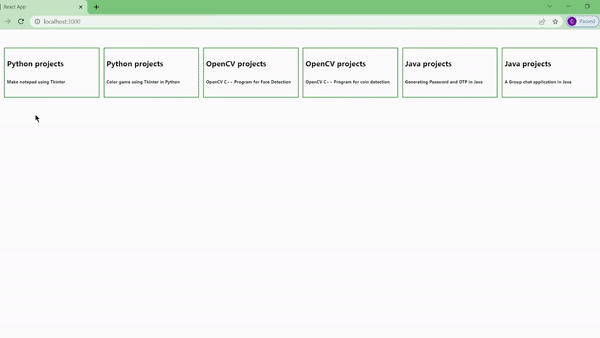
Share your thoughts in the comments
Please Login to comment...