What is the use of the localStorage.setItem Method ?
Last Updated :
26 Mar, 2024
localStorage.
setItem
method is used to store data in the browser’s localStorage object. When we call localStorage.setItem(key, value), it stores the specified value under the specified key in the browser’s localStorage.
The data stored using localStorage.setItem persists even after the browser is closed and reopened, making it useful for storing user preferences, session data, or other information that needs to be retained between sessions.
Syntax:
localStorage.setItem("key", string)
In this method, we take two arguments as a key, which is a string representing the name of the item we want to store, and a value, which can be of any data type supported by the JSON format (e.g., strings, numbers, arrays, objects).
Example 1: In this example, the we store string “Aayush” with the key “name” in the browser’s localStorage, then retrieve and print it.
JavaScript
// Storing a string in localStorage
localStorage.setItem('name', 'aayush');
// Retrieving the stored string
const storedName = localStorage
.getItem('name');
console.log('Stored name:', storedName);
Output:
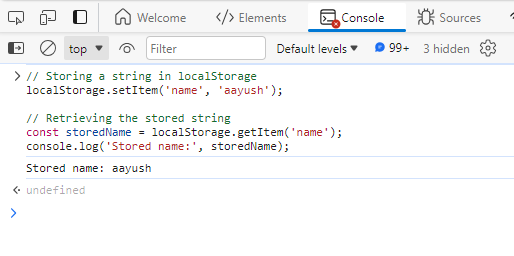
Example 2 : To demonstrate storing a number 30 with the key “age” in the browser’s localStorage, then retrieves, parses, and prints it.
JavaScript
// Storing a number in localStorage
localStorage.setItem('age', 30);
// Retrieving the stored number
const storedAge = parseInt(localStorage
.getItem('age'));
console.log('Stored age:', storedAge);
Output:
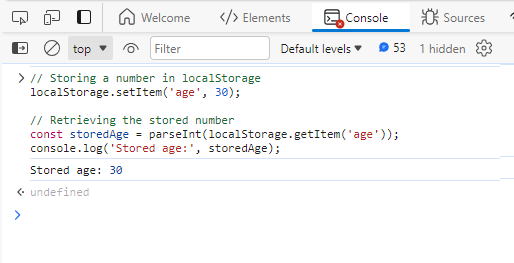
Example 3: In this example we store an array [“red”, “green”, “blue”] with the key “colors” in the browser’s localStorage after converting it to a JSON string, then retrieves, parses, and prints it.
JavaScript
// Storing an array in localStorage
const colors = ['red', 'green', 'blue'];
localStorage.setItem('colors', JSON.stringify(colors));
// Retrieving the stored array
const storedColors = JSON.
parse(localStorage.getItem('colors'));
console.log('Stored colors:', storedColors);
Output:
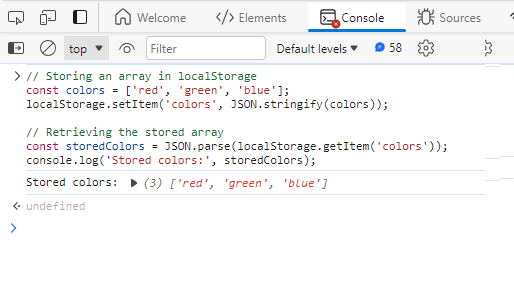
Example 4: In this example we store an object { name: ‘Atul’, age: 25, city: ‘India’ } with the key “person” in the browser’s localStorage after converting it to a JSON string, then retrieves, parses, and prints it.
JavaScript
// Storing an object in localStorage
const person = {
name: 'Atul',
age: 25,
city: 'India'
};
localStorage.setItem('person', JSON.stringify(person));
// Retrieving the stored object
const storedPerson = JSON
.parse(localStorage.getItem('person'));
console.log('Stored person:', storedPerson);
Output:
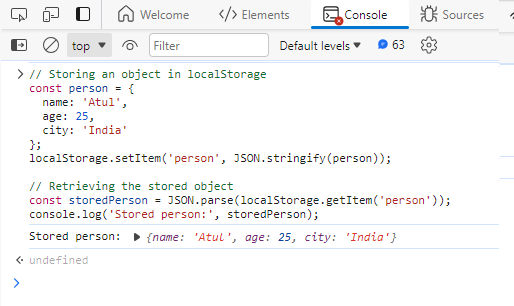
Share your thoughts in the comments
Please Login to comment...