What are the Benefits of using Immutable Data in Redux ?
Last Updated :
24 Apr, 2024
In Redux, immutable data means that once you create the state, you can’t directly change it. Instead, whenever you need to update the state, you create a new state object. This keeps your original state safe and offers benefits like predictability, easier debugging, and performance optimizations. In this article, we make a project that implements an Undo/Redo functionality and shows the use case of an immutable state in Redux.
Benefits of Immutable Data in Redux
- Predictability – Predictability means that you can easily understand how and when changes occur in your application’s state. With immutable data in Redux, every time you want to update the state, you create a new state object instead of modifying the existing one. This ensures that the state remains consistent and doesn’t change unexpectedly.
- Debugging – By eliminating the possibility of side effects (unintended consequences of modifying data), immutable data makes testing and debugging more straightforward. You can isolate specific parts of your code and test them with well-defined inputs.
- Performance – Immutable data in Redux improves performance by enabling faster change detection. Components connected to the state can efficiently determine if they need to re-render using shallow equality checks. This optimization is crucial as your app scales, handling larger state objects and complex component structures.
- Undo/Redo –Since every state change produces a new state object, implementing undo and redo functionality becomes straightforward. You can maintain a history of past states and navigate between.
Approach
- Setup Redux Store: We start by setting up a Redux store with actions and reducers to manage the state of the application. This includes defining actions for adding items, undo and redo changes.
- Create Components: We create React components to interact with the Redux store. These components include input fields for adding items and buttons for undoing and redoing actions. Reducers are also handled here.
- Styling : We add CSS styles to improve the appearance of the application and enhance the user experience.
- Connect Redux Store: We connect the Redux store to the application by wrapping the root component with the Provider component and providing the store. This allows components to access the application state and dispatch actions.
Steps to Create Application
Step 1: Create React Application named redo-undo-app and navigate to it using this command.
npx create-react-app undo-redo-app
cd undo-redo-app
Step 2: Install required packages and dependencies.
npm install react-redux @reduxjs/toolkit
Updated dependencies
All required dependencies required for project will look like in package.json file.
"dependencies": {
"@reduxjs/toolkit": "^2.2.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-redux": "^9.1.0",
},
Project Structure
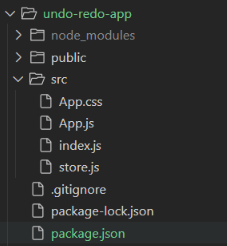
Project Structure
Example: To demonsrtate creating an immutable data in redux which can be controlled by using redux.
CSS
/* App.css */
.App {
text-align: center;
}
button {
background-color: rgb(203, 207, 202);
padding: 10px 20px;
border: 1px solid black;
cursor: pointer;
}
button:hover {
background-color: green;
}
input {
padding: 10px 20px;
border: 1px solid black;
}
JavaScript
// store.js
import { createSlice } from "@reduxjs/toolkit";
import { configureStore } from "@reduxjs/toolkit";
const itemSlice = createSlice({
name: "Item",
initialState: {
past: [],
present: null,
future: [],
},
reducers: {
addItem: (state, action) => {
state.past.push(state.present);
state.present = action.payload;
state.future = [];
},
undo: (state) => {
const previous = state.past.pop();
if (previous) {
state.future.unshift(state.present);
state.present = previous;
}
},
redo: (state) => {
const next = state.future.shift();
if (next) {
state.past.push(state.present);
state.present = next;
}
},
},
});
export const { addItem, undo, redo } = itemSlice.actions;
export default configureStore({
reducer: itemSlice.reducer,
});
JavaScript
// App.js
import "./App.css";
import React, { useState } from "react";
import { useDispatch, useSelector } from "react-redux";
import { undo, addItem, redo } from "./store";
function App() {
const dispatch = useDispatch();
const { future, past, present } = useSelector((state) => state);
const [item, setItem] = useState("");
const addItemHandle = () => {
if (!item) return;
dispatch(addItem(item));
setItem("");
};
return (
<div className="App">
<img
src="https://media.geeksforgeeks.org/gfg-gg-logo.svg"
alt="gfg_logo"
/>
<div>
<input
type="text"
value={item}
onChange={(e) => setItem(e.target.value)}
/>
<button onClick={addItemHandle}>Add Item</button>
<div>
<h2>Current Item: {present}</h2>
<button onClick={() => dispatch(undo())} disabled={past.length === 0}>
Undo
</button>
<button
onClick={() => dispatch(redo())}
disabled={future.length === 0}
>
Redo
</button>
</div>
</div>
</div>
);
}
export default App;
JavaScript
// index.js
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import { Provider } from "react-redux";
import store from "./store";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<Provider store={store}>
<App />
</Provider>
);
Step to Run the Application:
To run application, go to redo-undo-app and enter this command.
npm start
Output:
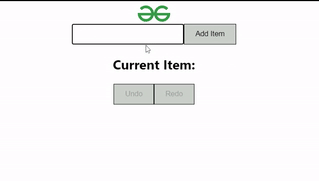
Final Output of Undo-Redo-app
Share your thoughts in the comments
Please Login to comment...