Spring MVC framework enables separation of modules namely Model, View, and Controller, and seamlessly handles the application integration. This enables the developer to create complex applications also using plain java classes. The model object can be passed between view and controller using maps. The Spring MVC framework is comprised of the following components:
- Model: A model can be an object or collection of objects which basically contains the data of the application.
- View: A view is used for displaying the information to the user in a specific format. Spring supports various technologies like freemarker, velocity, and thymeleaf.
- Controller: It contains the logical part of the application. @Controller annotation is used to mark that class as controller.
- Front Controller: It remains responsible for managing the flow of the web application. DispatcherServlet acts as a front controller in Spring MVC.
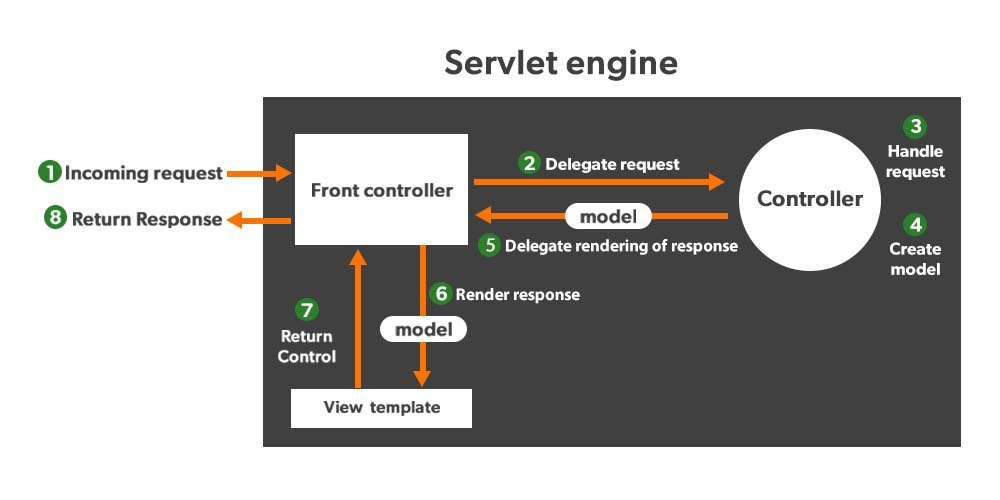
What is WebApplicationContext in Spring MVC?
WebApplicationContext in Spring is a web-aware ApplicationContext i.e it has Servlet Context information. In a single web application, there can be multiple WebApplicationContext. That means each DispatcherServlet is associated with a single WebApplicationContext. The WebApplicationContext configuration file *-servlet.xml is specific to the DispatcherServlet and a web application can have more than one DispatcherServlet configured to handle the requests and each DispatcherServlet would have a separate *-servlet.xml file to configure. Let’s understand these lines through a practical example. Before diving into the example project make sure you have referred to these articles
Example Project
Note: We are going to use Spring Tool Suite 4 IDE for this project. Please refer to this article to install STS in your local machine How to Download and Install Spring Tool Suite (Spring Tools 4 for Eclipse) IDE?
Step 1: Create a Dynamic Web Project in your STS IDE. You may refer to this article to create a Dynamic Web Project in STS: How to Create a Dynamic Web Project in Spring Tool Suite?
Step 2: Download the spring JARs file from this link and go to the src > main > webapp > WEB-INF > lib folder and past these JAR files.
Step 3: Refer to this article Configuration of Apache Tomcat Server and configure the tomcat server with your application. Now we are ready to go.
Step 4: Configuring Dispatcher Servlet
Go to the src > main > webapp > WEB-INF > web.xml file and the complete code for web.xml file is given below:
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< display-name >myfirst-mvc-project</ display-name >
< welcome-file-list >
< welcome-file >index.html</ welcome-file >
< welcome-file >index.jsp</ welcome-file >
< welcome-file >index.htm</ welcome-file >
< welcome-file >default.html</ welcome-file >
< welcome-file >default.jsp</ welcome-file >
< welcome-file >default.htm</ welcome-file >
</ welcome-file-list >
< absolute-ordering />
< servlet >
< servlet-name >frontcontroller-dispatcher</ servlet-name >
< servlet-class >org.springframework.web.servlet.DispatcherServlet</ servlet-class >
< load-on-startup >1</ load-on-startup >
</ servlet >
< servlet-mapping >
< servlet-name >frontcontroller-dispatcher</ servlet-name >
< url-pattern >/student.com/*</ url-pattern >
</ servlet-mapping >
</ web-app >
|
Now go to the src > main > webapp > WEB-INF and create an XML file. Actually, this is a Spring Configuration file like beans.xml file. And the name of the file must be in this format
YourServletName-servlet.xml
For example, for this project, the name of the file must be
frontcontroller-dispatcher-servlet.xml
So either you can create a Spring Configuration File or you can just create a simple XML file add the below lines of code inside that file. So the code for the frontcontroller-dispatcher-servlet.xml is given below.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
</ beans >
|
Step 5: Create Your Spring MVC Controller
Now, let’s create some controllers. Go to the src/main/java and create a new controllers package (For ex. com.student.controllers) as per your choice. And inside that create a Java class and name the class as DemoController. Now how to tell the Spring that this is our controller class. So the way we are going to tell the Spring is by marking it with a @Controller annotation.
@Controller
public class DemoController {
}
Note: Spring will automatically initialize the class having a @Controller annotation and register that class with the spring container.
Now let’s create a simple method inside the Controller class and use @RequestMapping and @ResponseBody annotation before the method something like this.
@ResponseBody
@RequestMapping("/hello")
public String helloWorld() {
return "Hello World!";
}
Now let’s understand both annotations.
@RequestMapping(“/hello”), so what does it mean? This line means, in the URL if somebody hits student.com/hello then this particular method will be executed and it is going to perform the operation that is written inside that particular method. For example, for this project, we are just returning a message “Hello World!” and we are expecting this is going to display in the client browser. But this thing will not happen. And to make it happen we need to use the @ResponseBody annotation. So @ResponseBody annotation is bacillary gonna write this particular message, here “Hello World!”, in your HTTP response. Below is the code for the DemoController.java file
Java
package com.student.controllers;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class DemoController {
@ResponseBody
@RequestMapping ( "/hello" )
public String helloWorld() {
return "Hello World!" ;
}
}
|
Step 6: Add the below line inside the frontcontroller-dispatcher-servlet.xml file
<context:component-scan base-package="com.student.controllers"></context:component-scan>
So the complete code for the frontcontroller-dispatcher-servlet.xml is given below.
XML
<? xml version = "1.0" encoding = "UTF-8" ?>
< context:component-scan base-package = "com.student.controllers" ></ context:component-scan >
</ beans >
|
Step 7: Run Your Spring MVC Controller
To run your Spring MVC Application right-click on your project > Run As > Run on Server and run your application as shown in the below image.
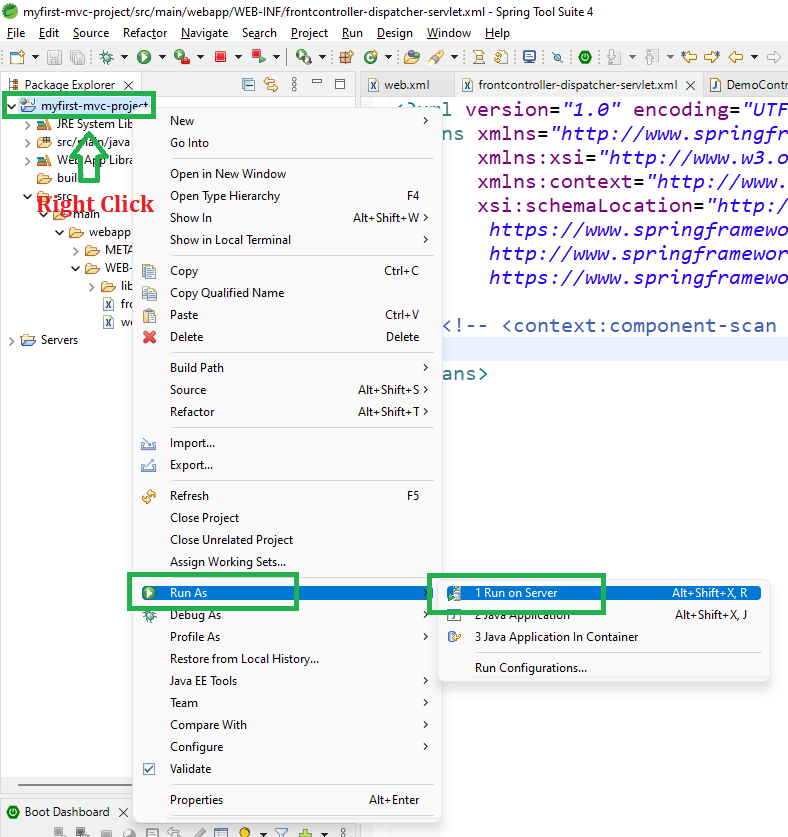
After that use the following URL to run your controller as shown in the below image. All other details are mentioned in the image.
http://localhost:8080/myfirst-mvc-project/student.com/hello
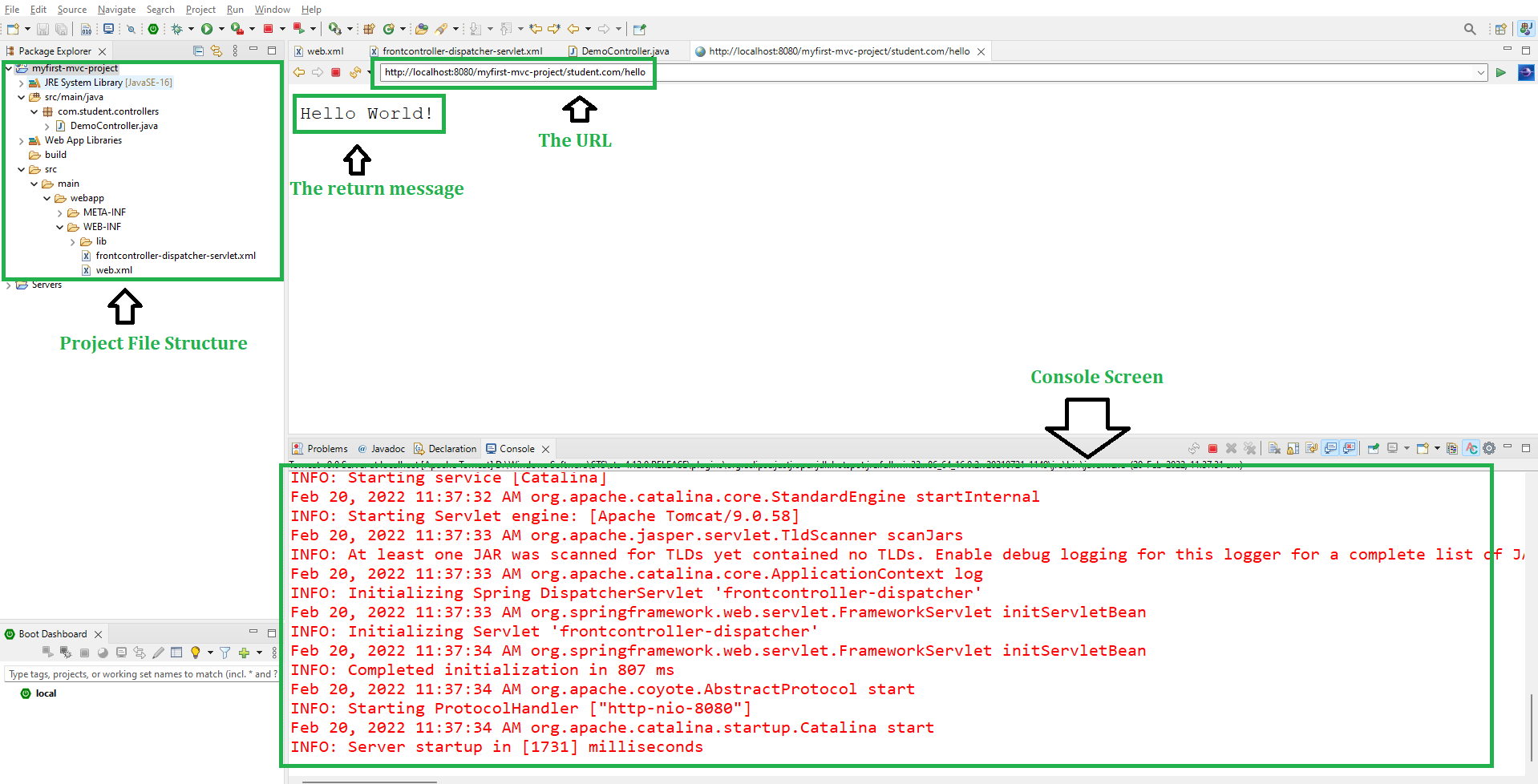
So our project is working fine. Now let’s understand the project flow. And here we’re going to learn the concept behind the WebApplicationContext.
Spring Web MVC Project Flow
In the below console screen you can see our Spring DispatcherServlet has been initialized, but what’s next? So when it is getting initialized, it is looking for the frontcontroller-dispatcher-servlet.xml file and once it got that particular file it is trying to create a container out of this frontcontroller-dispatcher-servlet.xml file. And what type of container it is trying to create? It is trying to create a container called WebApplicationContext.
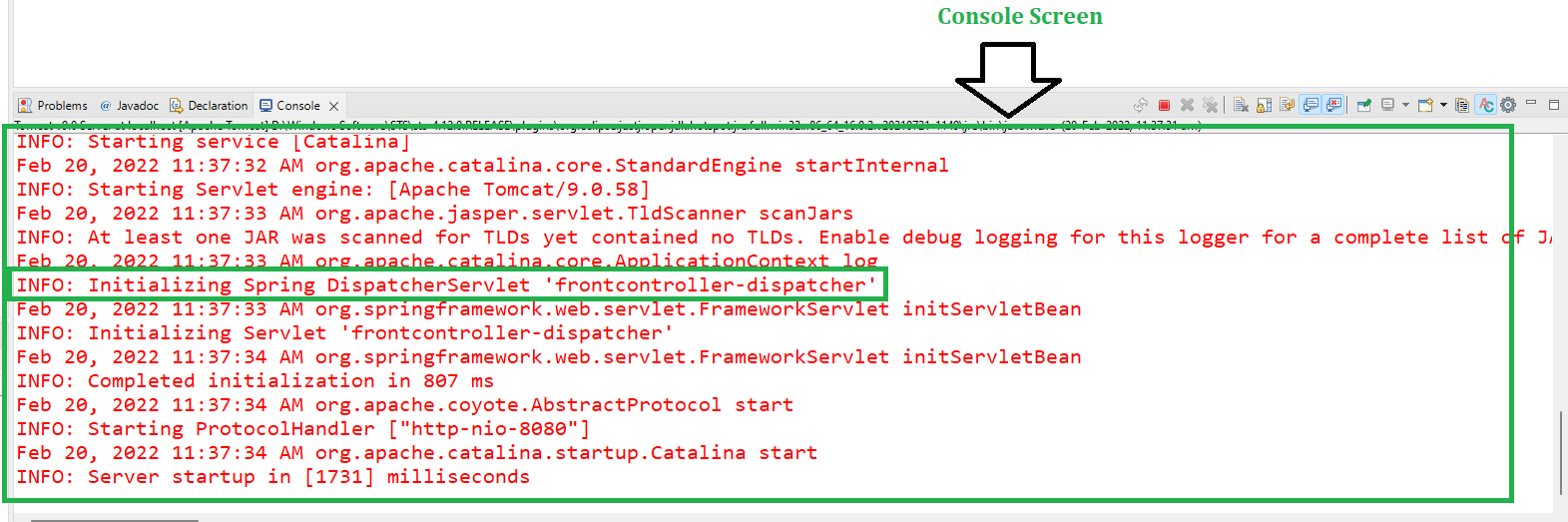
Let’s now try to understand WebApplicationContext by differentiating it with ApplicationContext.
Difference Between ApplicationContext and WebApplicationContext in Spring MVC
ApplicationContext is our core container but right now in this project, we are dealing with a web application. ApplicationContext is used to create standalone applications. But for the web applications, we have to deal with a container called WebApplicationContext. But the good thing is we don’t have to create the WebApplicationContext instance manually. In web applications, it is not our responsibility to start and close the WebApplicationContext.
Important Point to Remember
A. Standalone App
// Creating container objects manually
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
// Destroying container object manually
context.close();
B. Web App
You don’t need to create and destroy the container object. The container object will be created automatically with the server startup and destroyed when we stop the server.
One more interesting difference is both ApplicationContext and WebApplicationContext are the spring containers where WebApplicationContext is the child of the ApplicationContext interface.
public interface WebApplicationContext extends ApplicationContext {
...............
}
Now come to the flow of the project again. The DispatcherServlet will create a WebApplicationContext container from the frontcontroller-dispatcher-servlet.xml file. Now come to this line
<context:component-scan base-package="com.student.controllers"></context:component-scan>
which is written inside the frontcontroller-dispatcher-servlet.xml file. When the DispatcherServlet will load this particular file it is going to look for the “com.student.controllers” package and it will search in every class. If any class has an annotation called @Component or @Controller then the framework will create the object for it. For example, in this project, the DemoController.java file has a @Controller annotation. So now this class is going to register with your WebApplicationContext container. Below is a pictorial representation of this working flow
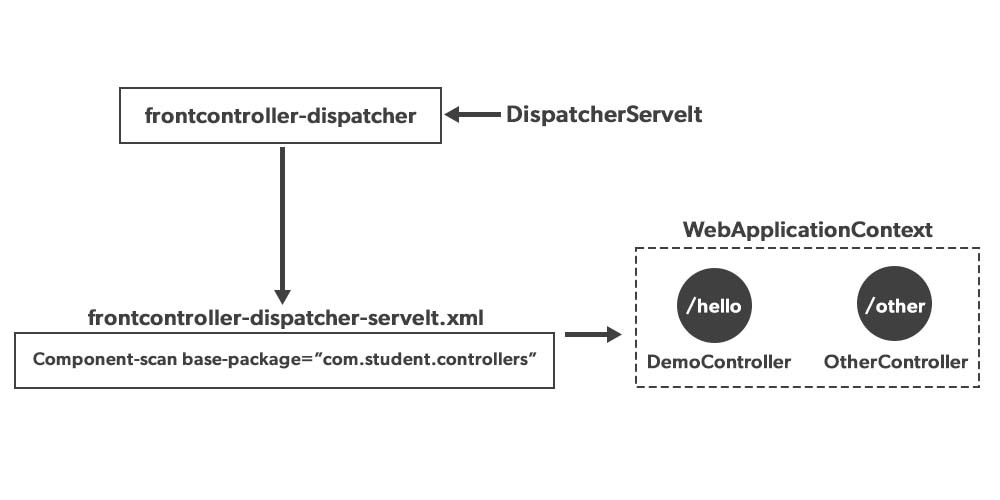
So now all the classes having @Controller annotation have registered with your WebApplicationContext container. And when you hit the following URL ending with the /student.com/hello then first, the request is coming to the DispatcherServlet(inside the web.xml file) and if it matches with the /student.com the “frontcontroller-dispatcher” servlet will handle that request. Once it matches then it will look for the /hello URL pattern, now the “frontcontroller-dispatcher” DispatcherServlet will try to resolve this particular request. And it has already a container and inside that container, it has already the class (DemoController.java) has been registered. So right now the DispatcherServlet is going to scan all the handler methods and it will check the desired URL pattern. And it will execute the particular method.
Share your thoughts in the comments
Please Login to comment...