Web API Geolocation
Last Updated :
21 Dec, 2023
Web API Geolocation enables the users to use the E-commerce websites that do the same for getting the location of the user to deliver products, News Web apps need the user’s location to serve the news relevant and based on the user’s location. Many apps, nowadays, request the user to access the location of the device. Websites make use of this Geolocation Web API which provides certain methods and properties that we can use in our web application and get the location details of the user like latitude, longitude, altitude, and velocity of the device.
Concept and Usage
Navigator is the global object containing services that we can use and get the user and browser environment details like OS details, network details, and location details.
Concepts
|
Descriptions
|
navigator.geolocation |
The navigator.geolocation property facilitates to be provided by the navigator global object. Here, navigator.geolocation returns us a Geolocation object, which we will use this object to call the Geolocation Web API methods to retrieve the location of a user.
|
Geolocation.getCurrentPosition() |
It has a return value undefined. In the Geolocation.getCurrentPosition method there are three parameters these are success, error, and options.
|
Geolocation.watchPosition() |
In the Geolocation.watchPosition method there are three parameters success, error, and options. It Returns the current position of the device and sets a callback function which will be called every time the device changes its position and returns an ID.
|
GeolocationPosition |
GeoLocationPosition represents the properties for determining the location of the device as well as the timestamp of the location retrieved.
|
GeolocationPositionError |
This provides us the properties to get the Information about the error raised while getting the location of the device.
|
Interfaces in Geolocation Web API
GeolocationCoordinates Interface
This interface represents the properties to get the location details like altitude, speed, latitude, longitude, direction, and details about the accuracy of the returned data.
Interfaces
|
Descriptions
|
latitude |
GeolocationCoordinates.latitude returns a double value representing the latitude of the current device. |
longitude |
GeolocationCoordinates.longitude returns a double value representing the longitude of the current device. |
altitude |
GeolocationCoordinates.altitude returns a double value representing the height of the device from the sea level. If the underlying machine cannot provide it, it returns null. |
speed |
GeolocationCoordinates.speed returns the current velocity with which the device is traveling, If the underlying machine cannot provide it, it returns null. |
heading |
GeolocationCoordinates.heading returns the direction towards which the device is traveling, the measurements are considered taking true north as the reference. If the speed of the device is zero, then it returns NaN. If the underlying machine cannot provide this functionality, then it returns null. |
accuracy |
GeolocationCoordinates.accuracy returns a double value which represents the accuracy of latitude and longitude in meters. |
altitudeAccuracy |
GeolocationCoordinates.altitudeAccuracy returns a double value which represents the accuracy of the altitude of the device in meters. If the underlying machine cannot provide this functionality, then it returns null. |
GeoLocationPosition Interface
GeoLocationPosition Interface represents the properties for determining the location of the device as well as the timestamp of the location retrieved.
Interfaces
|
Descriptions
|
coords |
In the property coords, GeolocationPosition.coords represents the GeolocationCoordinates object containing details like speed, accuracy, latitude, longitude, altitude, etc. It returns the object containing GeolocationCoordinates properties. |
timestamp |
In the property timestamp, GeolocationPosition.timestamp returns the time stamp in milliseconds at which the location was retrieved. |
GeolocationPositionError Interface
This Interface provides us the properties to get the Information about the error raised while getting the location of the device.
Interfaces
|
Descriptions
|
code |
In the property code, GeolocationPositionError.code returns an unsigned short integer which specifies the error code where error code ‘1’ specifies the error raised during location request due to permission policies, error code ‘2’ specifies the error raised due to some internal error in the process of acquiring location, and error code ‘3’ specifies the error raised when the information is still not received to the browser before the timeout specified. |
message |
In the property message, the GeolocationPositionError.message returns the description of an error that occurred in the form of a string. |
GeoLocation Interface
This Interface provides the methods to obtain the location of the device as well as to get the location dynamically as the device changes it’s position.
Interfaces
|
Descriptions
|
getCurrentPosition() |
The navigator.geolocation.getCurrentPosition(success_function, error_handler, options) Returns the GeoLocationPosition object as data. |
getWatchPosition() |
It will return the current position of the device (GeolocationPosition Object) and set a callback function which will be called every time the device changes its position and returns an ID. |
clearWatch() |
It clears the callback function that we have registered using getWatchPosition(). It takes an ID as the input and clears the watch function associated with that ID. |
Example 1: In this example, the browser will execute the callback function that needs to be executed when the location request is successful.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Geo Location Web API</ title >
</ head >
< body >
< h2 >Welcome To GFG</ h2 >
< button onclick = "getlocation()" >
Get Location Details
</ button >
< p id = "location_details" ></ p >
< script >
function getlocation() {
var geo_loc_obj = navigator.geolocation;
var geo_position_object = geo_loc_obj
.getCurrentPosition(displaylocation, error_handler, options);
}
const options = {
enableHighAccuracy: true,
timeout: 1000,
maximumAge: 0,
};
function displaylocation(geo_position_object) {
var latitude =
geo_position_object.coords.latitude;
var longitude =
geo_position_object.coords.longitude;
var accuracy =
geo_position_object.coords.accuracy;
var altitude =
geo_position_object.coords.altitude;
var speed =
geo_position_object.coords.speed;
var altitudeAccuracy =
geo_position_object.altitudeAccuracy;
var heading =
geo_position_object.heading;
document.getElementById("location_details")
.innerHTML = "Latitude : " + latitude + "< br >" +
"Longitude : " + longitude + "< br >" +
"Accuracy : " + accuracy + "< br >" +
"Altitude : " + altitude + "< br >" +
"Speed : " + speed + "< br >" +
"Altitude Accuracy : " + altitudeAccuracy + "< br >" +
"Heading : " + heading + "< br >"
}
function error_handler(geo_position_error_obj) {
const error_messg =
geo_position_error_obj.message;
const error_code =
geo_position_error_obj.code;
console.log(
"Location access failed. " +
"The error code and descriptions are:")
console.log("error_code " + error_code);
console.log("error_message " + error_messg);
}
</ script >
</ body >
</ html >
|
Output :
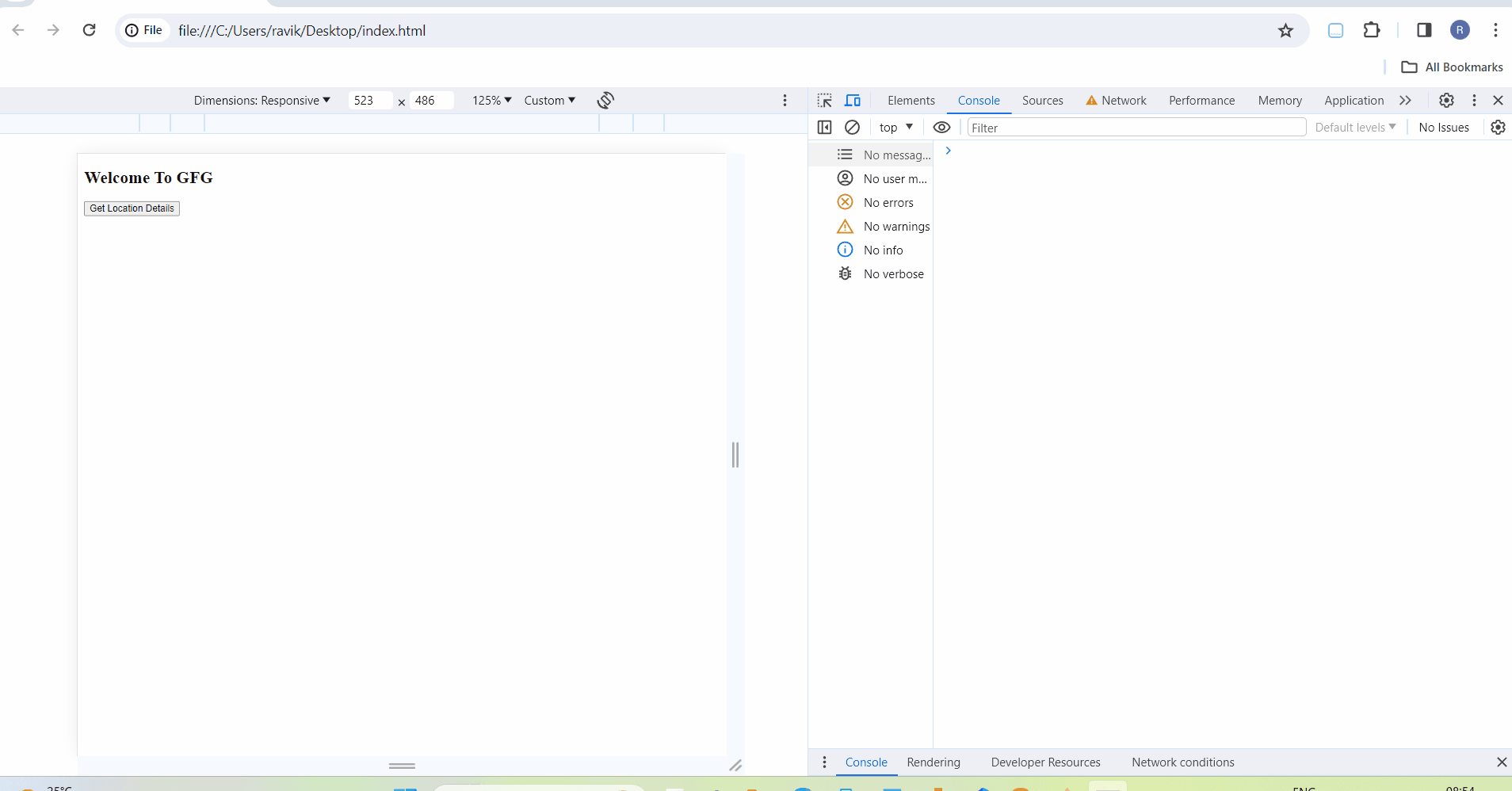
Demonstration of Successful Geolocation Retrieval.
Example 2: In this example, when a user rejects the location request the browser will execute the callback function that needs to be executed when the location request fails due to some reason, here we get the output as “1” and the description of the error message as “user rejected the request”.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Geo Location Web API</ title >
</ head >
< body >
< h2 >Welcome To GFG</ h2 >
< button onclick = "getlocation()" >
Get Location Details
</ button >
< p id = "location_details" ></ p >
< script >
function getlocation() {
var geo_loc_obj = navigator.geolocation;
var geo_position_object = geo_loc_obj
.getCurrentPosition(
displaylocation, error_handler, options);
}
const options = {
enableHighAccuracy: true,
timeout: 1000,
maximumAge: 0,
};
function displaylocation(geo_position_object) {
var latitude =
geo_position_object.coords.latitude;
var longitude =
geo_position_object.coords.longitude;
var accuracy =
geo_position_object.coords.accuracy;
var altitude =
geo_position_object.coords.altitude;
var speed =
geo_position_object.coords.speed;
var altitudeAccuracy =
geo_position_object.altitudeAccuracy;
var heading =
geo_position_object.heading;
document.getElementById("location_details")
.innerHTML = "Latitude : " + latitude + "< br >" +
"Longitude : " + longitude + "< br >" +
"Accuracy : " + accuracy + "< br >" +
"Altitude : " + altitude + "< br >" +
"Speed : " + speed + "< br >" +
"Altitude Accuracy : " + altitudeAccuracy + "< br >" +
"Heading : " + heading + "< br >"
}
function error_handler(geo_position_error_obj) {
const error_messg =
geo_position_error_obj.message;
const error_code =
geo_position_error_obj.code;
console.log(
"Location access failed. "+
"The error code and descriptions are:")
console.log("error_code " + error_code);
console.log("error_message " + error_messg);
}
</ script >
</ body >
</ html >
|
Output:
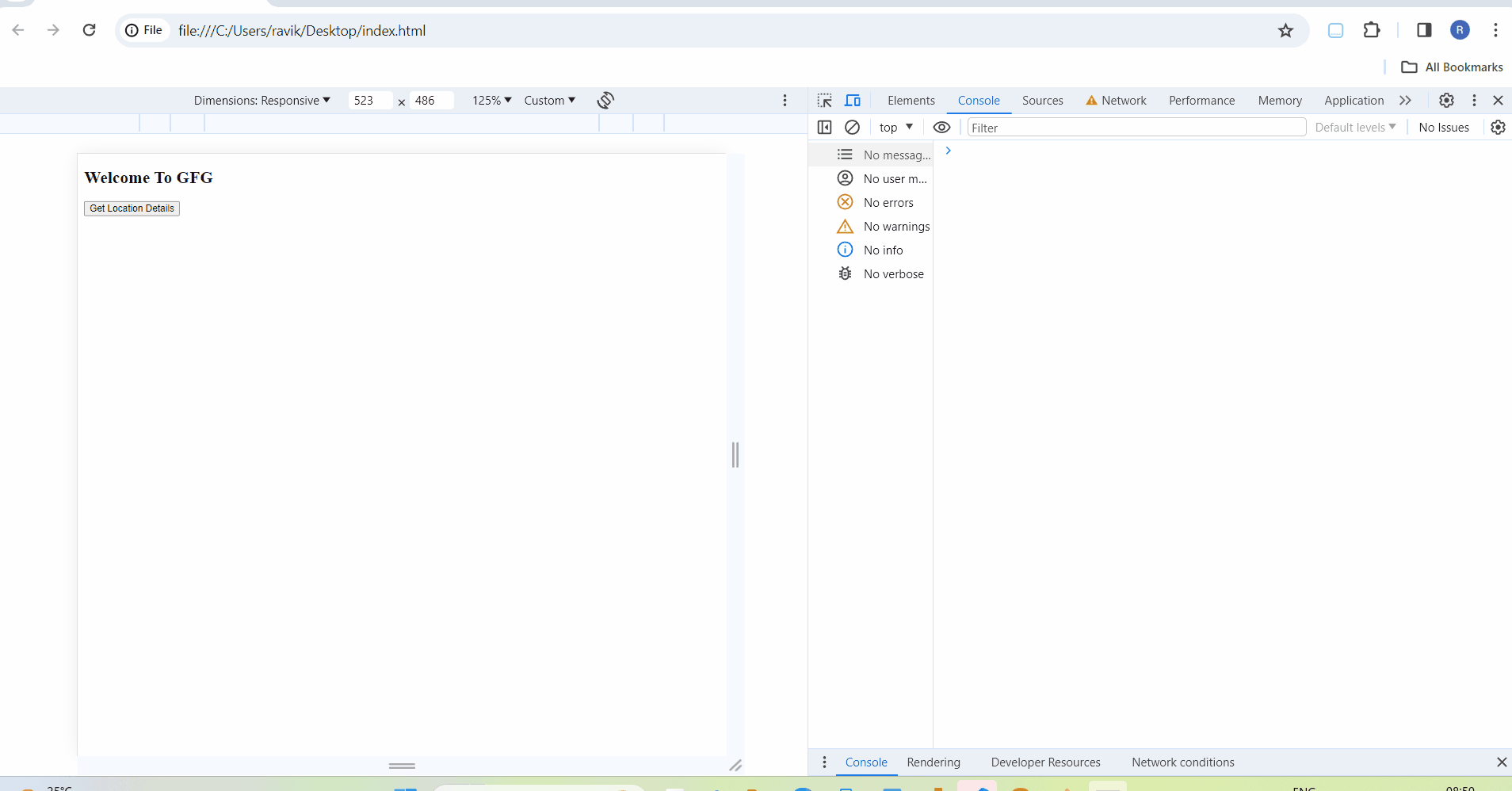
Demonstration of Location Retrieval Failure due to the user rejecting the request.
Browser Support
- Google Chrome 5.0 & above
- Microsoft Edge 12.0 & above
- Mozilla Firefox 3.5 & above
- Opera 10.6 & above
- Safari 5.0 & above
Share your thoughts in the comments
Please Login to comment...