How to Fix “ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()”
Last Updated :
02 Oct, 2023
If we work with NumPy or Pandas in Python, we might come across the ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all() error. In this article, we will the reason as well as the solution to fix this error.
ValueError: The truth value of an array with more than one element is ambiguous
In Python, boolean values are often used for making binary decisions in programs. Essentially, boolean values can be used to specify whether a certain condition holds true or false. The True and False values represent the two possible states of a boolean variable. However, when evaluating the truthiness of a NumPy or Pandas multi-element array, a clear decision cannot always be made on whether the array as a whole is True or False.
Problem
Suppose there is a NumPy array that contains both True and False values:
Python3
import numpy as np
arr = np.array([ True , False , True ])
if arr:
print ( "The array is evaluated as True." )
else :
print ( "The array is evaluated as False." )
|
Output
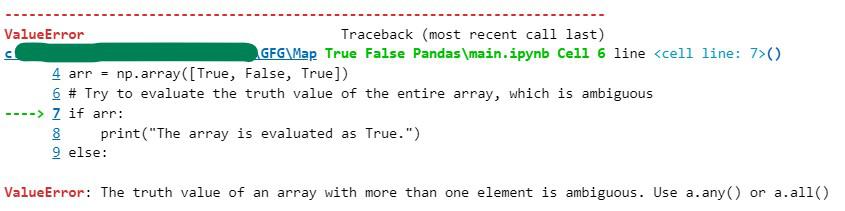
This ambiguity arises because, in Python, an array with multiple elements cannot be implicitly converted to a single boolean value.
Python Resolve “ValueError: The truth value of an array with more than one element is ambiguous”
Below are the ways by which we can resolve the following error:
Using any() method
The numpy.any() function returns True if at least one element in the iterable is True, and False if all elements are False or if the iterable is empty. In this example, we achieve the desired outcome by using the any() function to check whether there is at least one True value in the array or not.
Python3
import numpy as np
arr = np.array([ True , False , True ])
arr2 = np.array([ False , False , False ])
if arr. any ():
print ( "At least one element in the first array is True" )
else :
print ( "No element in the first array is True" )
if arr2. any ():
print ( "At least one element in the second array is True" )
else :
print ( "No element in the second array is True" )
|
Output

Using all() method
The numy.all() function returns True if all elements in the iterable are True, and False if at least one element is False or if the iterable is empty. In this example, we achieve the desired outcome by using the all() function to check whether all elements are of True value in the array or not.
Python3
import numpy as np
arr = np.array([ True , True , True ])
arr2 = np.array([ True , False , False ])
if arr. all ():
print ( "All elements in the first array are True." )
else :
print ( "At least one element in the first array is False." )
if arr2. all ():
print ( "All elements in the second array are True." )
else :
print ( "At least one element in the first array is False." )
|
Output

Using logical_and() and logical_or():
The logical_and() and logical_or() functions from NumPy explicitly perform element-wise logical operations on arrays, returning arrays of Boolean values. This approach avoids the ambiguity of trying to reduce an entire array to a single truth value.
Python3
import numpy as np
array1 = np.array([ True , False , True , False , True ])
array2 = np.array([ False , True , True , False , False ])
result_and = np.logical_and(array1, array2)
result_or = np.logical_or(array1, array2)
print ( "Array 1:" )
print (array1)
print ( "\nArray 2:" )
print (array2)
print ( "\nResult of Element-wise Logical AND (result_and):" )
print (result_and)
print ( "\nResult of Element-wise Logical OR (result_or):" )
print (result_or)
|
Output
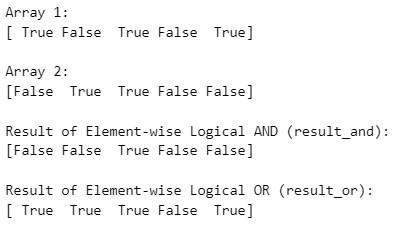
Using Logical AND with “&” and Logical OR with “|”:
Using the & operator for element-wise logical AND and the | operator for element-wise logical OR provides a clear and concise way to perform these operations on arrays. Like logical_and() and logical_or(), these operators return arrays of Boolean values, avoiding ambiguity.
Python3
import numpy as np
array1 = np.array([ True , False , True , False , True ])
array2 = np.array([ False , True , True , False , False ])
result_and = array1 & array2
result_or = array1 | array2
print ( "Array 1:" )
print (array1)
print ( "\nArray 2:" )
print (array2)
print ( "\nResult of Element-wise Logical AND (result_and):" )
print (result_and)
print ( "\nResult of Element-wise Logical OR (result_or):" )
print (result_or)
|
Output
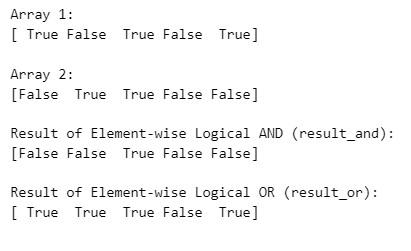
Just like with the logical_and() and logical_or() methods, these operations result in arrays of Boolean values that clarify the logical operations and avoid the ambiguity of reducing an entire array to a single truth value.
Using boolean indexing:
In this case, the condition is a Boolean array that specifies which elements meet the condition array > 3. When you use boolean indexing with array[condition], you are explicitly selecting elements based on the condition, eliminating ambiguity.
Python3
import numpy as np
array = np.array([ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 ])
condition = array % 2 = = 0
filtered_array = array[condition]
print ( "Original Array:" )
print (array)
print ( "\nFiltered Array (Even Numbers):" )
print (filtered_array)
|
Output
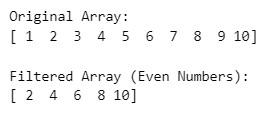
Share your thoughts in the comments
Please Login to comment...