TypeScript Inference with Template Literals
Last Updated :
08 Nov, 2023
TypeScript Inference with Template Literals helps to create specific types based on string patterns. However, they’re not mainly used for making sure that an attribute’s type matches its callback function’s argument type. TypeScript uses different methods like inference and generics for that job. Template literal types are more about working with strings and creating types based on them, not directly for linking attribute types with their callback function arguments.
Example 1: In this example, we showcase the definition of AttributeTypes
specific data types for attributes. The setAttribute
function takes an attribute key, its new value, and a callback function. The function ensures the callback parameter aligns with the attribute’s data type. The examples demonstrate how to use setAttribute
to update attributes and trigger respective callbacks.
Javascript
type AttributeTypes = {
name: string;
age: number;
email: string;
};
function setAttribute<K extends keyof AttributeTypes>(
attribute: K,
value: AttributeTypes[K],
callback: (newValue: AttributeTypes[K]) => void
) {
callback(value);
}
setAttribute( "name" , "GeeksforGeeks" , (newValue) => {
console.log( "Setting name to:" , newValue);
});
setAttribute( "age" , 25, (newValue) => {
console.log( "Setting age to:" , newValue);
});
setAttribute( "email" , "GeeksforGeeks@geeksforgeeks.org" , (newValue) => {
console.log( "Setting email to:" , newValue);
});
|
Output:
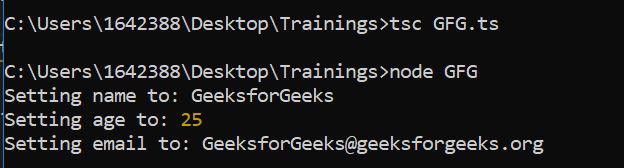
Example 2: In this example, we will see the literal type can be validated as being in the union of valid attributes in the generic. The type of the validated attribute can be looked up in the generic’s structure using Indexed Access. This typing information can then be applied to ensure the argument to the callback function is of the same type.
Javascript
type AttributeNames = 'name' | 'age' | 'email' ;
type AttributeTypes = {
name: string;
age: number;
email: string;
};
type AttributeCallbacks = {
[K in AttributeNames]:
(value: AttributeTypes[K]) => void;
};
function setAttribute<K extends AttributeNames>(
attribute: K,
value: AttributeTypes[K],
callbacks: AttributeCallbacks
) {
const callback = callbacks[attribute];
callback(value);
}
const callbacks: AttributeCallbacks = {
name: (newValue) => {
console.log( "Setting name to:" , newValue);
},
age: (newValue) => {
console.log( "Setting age to:" , newValue);
},
email: (newValue) => {
console.log( "Setting email to:" , newValue);
},
};
setAttribute( "name" , "Akshit" , callbacks);
setAttribute( "age" , 25, callbacks);
setAttribute( "email" , "akshit@geeksforgeeks.org" , callbacks);
|
Output: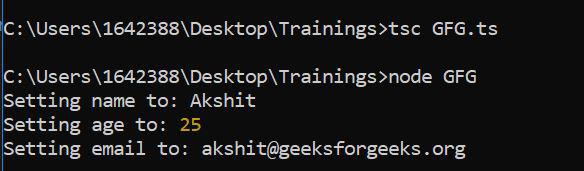
Conclusion :This article highlights how template literal types enable aligning an attribute’s data type with the first argument of its corresponding callback.
Share your thoughts in the comments
Please Login to comment...