TypeScript Construct Signatures
Last Updated :
08 Nov, 2023
TypeScript Construct Signatures defines the shape of a constructor function for creating instances of a class or object type. This signature specifies the parameters that the constructor function expects when using the new operator to create objects, as well as the type of object it constructs. You can write a construct signature by adding the new keyword in front of a call signature.
Syntax:
type Constructor = new (param1: Type1, param2: Type2, ...) => ReturnType;
Parameters:
- Constructor: This is the name you give to the type that represents the constructor function.
- new: The new keyword indicates that this is a construct signature for creating new instances.
- ( and ): These parentheses enclose the parameter list for the constructor function.
- param1, param2, …: These are the names of the parameters that the constructor function expects when creating instances. Each parameter is followed by a colon and its respective type (e.g., Type1, Type2, …).
ReturnType:
This is the type of object that the constructor function constructs and returns when called with the new operator.
Example 1: In this example, we define a TypeScript class Person with a constructor that takes a name parameter and sets the name property of the instance. Inside the class, we have a greet method that logs a greeting message with the person’s name. We create an instance of the Person class using the new operator and provide “GeeksforGeeks” as the name parameter.
Javascript
class Person {
name: string;
constructor(name: string) {
this .name = name;
}
greet() {
console.log(`Hello, my name is ${ this .name}`);
}
}
const person = new Person( "GeeksforGeeks" );
person.greet();
|
Output:
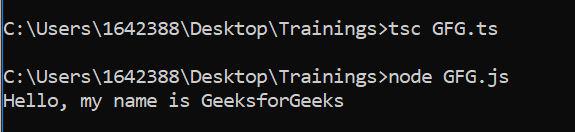
Example 2: In this example, we have created a constructor in a class named book and for creating an objcet of that book class we are calling a function createBook and passing the needed parameters and it returns a new object of Book.
Javascript
class Book {
constructor(
public title: string, public author: string) { }
}
type BookConstructor =
new (title: string, author: string) => Book;
function createBook(BookConstructor: BookConstructor,
title: string, author: string) {
return new BookConstructor(title, author);
}
const bookInstance = createBook(Book,
"The Story of My Experiments" , "Mahatma Gandhi" );
console.log( "Title:" , bookInstance.title);
console.log( "Author:" , bookInstance.author);
|
Output:
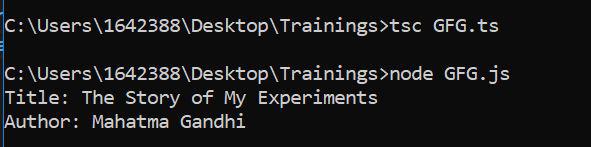
Share your thoughts in the comments
Please Login to comment...